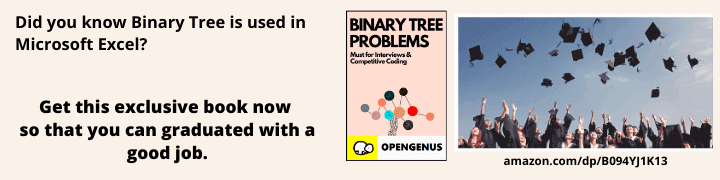
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes
The new operator denotes a request for memory allocation on the Heap. If sufficient memory is available, new operator initializes the memory and returns the address of the newly allocated and initialized memory to the pointer variable.
Syntax :
pointer-variable = new data-type;
Initialize memory:
We can also initialize the memory using new operator:
pointer-variable = new data-type(value);
Example:
int *p = new int(25);
float *q = new float(75.25);
Allocate block of memory:
new operator is also used to allocate a block(an array) of memory of type data-type.
pointer-variable = new data-type[size];
where size(a variable) specifies the number of elements in an array.
Example:
int *p = new int[10]
Dynamically allocates memory for 10 integers continuously of type int and returns pointer to the first element of the sequence, which is assigned to p(a pointer). p[0] refers to first element, p[1] refers to second element and so on.
dynamic
Normal Array Declaration vs Using new
There is a difference between declaring a normal array and allocating a block of memory using new. The most important difference is, normal arrays are deallocated by compiler (If array is local, then deallocated when function returns or completes). However, dynamically allocated arrays always remain there until either they are deallocated by programmer or program terminates.
How does memory gets allocated
Case 1: Using "new" to allocate an array, as in
int* Array = new int[10];
In this case, each element of foo will be in contiguous virtual memory.
Case 2: Using consecutive "new" operations non-atomically, as in
int* foo = new int;
int* bar = new int;
In this case, there is never a guarantee that the memory allocated between calls to "new" will be adjacent in virtual memory.
What if enough memory is not available during runtime?
If enough memory is not available in the heap to allocate, the new request indicates failure by throwing an exception of type std::bad_alloc, unless “nothrow” is used with the new operator, in which case it returns a NULL pointer Exception handling of new operator . Therefore, it may be good idea to check for the pointer variable produced by new before using it program.
int *p = new(nothrow) int;
if (!p)
{
cout << "Memory allocation failed\n";
}
Difference between new and malloc
- new calls constructor while malloc doesnot calls constructors.
- new is an operator while malloc is a function
- new returns exact data type while malloc returns void *
- On failure new throws while malloc returns NULL
- Memory allocated from free store in new while memory allocated is from heap in malloc.
- new can be overridden and malloc cannot be overridden
- size is calculated by compiler for new while size is calculated manually in malloc.
Question :
Consider the following C++ program:
#include <iostream>
using namespace std;
class Test
{
int x;
Test() { x = 5;}
};
int main()
{
Test *t = new Test;
cout << t->x;
}