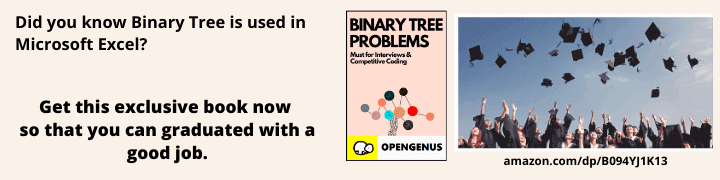
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will discuss several ways to program a Progress bar in Java in command line and as GUI.
Pre-requsites:
- Java
- String
- StringBuffer / StringBuilder
- Swing library
Progress bar in command line (easy method)
In this method we will make our program by just using simple java without using strings and string built-in functions.
- We create void method printMsgWithProgressBar with three parameters, String message to print the "Loading...." string, integer length to pass the length of our loading string and the integer timeInterval to pass the time we need the loading to take.
- We intialize two char variables, incomplete as 'â–‘' to show that this part of loading is not completed and 'â–ˆ' to show that this part of loading is completed.
- Initialize a loop variable i to 0 and iterate while i is less than or equal to length.
- For each iteration of the outer loop, print an opening bracket to start the progress bar.
- Initialize a loop variable j to 0 and iterate while j is less than length.
For each iteration of the inner loop, check if j is less than i. - If j is less than i, print the complete character. This indicates that the progress has reached or passed the current position.
- Otherwise, print the incomplete character. This indicates that the progress has not yet reached the current position.
- After printing all the characters for the progress bar, print a carriage return character to move the cursor back to the beginning of the line.
- Pause the program for the specified time interval using the Thread.sleep() method.
- Increment the loop variable i and repeat from step 2 until i is greater than length.
- Finally we call our method with given arguments in the main method.
public class ProgressBar {
public static void printMsgWithProgressBar(String message, int length, long timeInterval) throws Exception {
char incomplete = 'â–‘';
char complete = 'â–ˆ';
System.out.println(message);
for (int i = 0; i <= length; i++) {
for (int j = 0; j < length; j++) {
if (j < i) {
System.out.print(complete);
}
else {
System.out.print(incomplete);
}
}
System.out.print("\r");
Thread.sleep(timeInterval);
}
}
public static void main(String[] args) throws Exception {
printMsgWithProgressBar("Loading....", 60, 200);
}
}
Output:
Progress Bar in command line (Using StringBuilder)
In this method we will make our program by using java with using StringBuilder and StringBuffer.
- The code creates a progress bar display in the command line using a loop and a combination of StringBuilder and StringBuffer objects.
- The progress bar display is used to represent the completion of a task and is updated during each iteration of the loop to reflect the progress made.
- The progress bar display consists of a left square bracket, followed by a series of equal signs to represent the progress, and then a series of spaces to fill out the remaining width of the progress bar.
- The StringBuilder object is used to build the initial progress bar display, and the StringBuffer object is used to update the progress bar display during each iteration of the loop.
- The progressBarWidth variable represents the width of the progress bar display, and the progress variable represents the progress made so far.
- The progressBarTemplate string is used as a template for the progress bar display, with a placeholder for the progress percentage.
- The append() method of StringBuilder and StringBuffer is used to concatenate strings together and build the progress bar display.
- The progressBarBuffer.append(progressBarTemplate) line uses the append() method to add the initial progress bar display to the progressBarBuffer object.
- The System.out.print("\r" + progressBarBuffer) line prints the current progress bar display to the console, with the \r character used to overwrite the previous display.
- The progressBarBuffer.setCharAt(i, '=') line updates the progress bar display during each iteration of the loop by replacing the appropriate equal sign with a > character to show the progress made.
- The complex line progressBarBuffer.append("] ").append(progress * 100 / progressBarWidth).append('%') appends a right square bracket, the progress percentage (calculated as progress * 100 / progressBarWidth), and a percentage sign to the progressBarBuffer object. This line is used to update the progress bar display during each iteration of the loop.
public class ProgressBarGUI {
public static void main(String[] args) {
int totalTasks = 100;
int currentTask = 0;
int progressBarWidth = 50;
System.out.println("Loading....");
StringBuilder progressStringBuilder = new StringBuilder();
for (int i = 0; i < progressBarWidth; i++) {
progressStringBuilder.append(" ");
}
while (currentTask <= totalTasks) {
int progress = (int) (currentTask / (float) totalTasks * progressBarWidth);
StringBuffer progressBarBuffer = new StringBuffer(progressBarWidth + 10);
progressBarBuffer.append('[');
for (int i = 0; i < progressBarWidth; i++) {
if (i < progress) {
progressBarBuffer.append('=');
} else if (i == progress) {
progressBarBuffer.append('>');
} else {
progressBarBuffer.append(' ');
}
}
progressBarBuffer.append("] ").append(progress * 100 / progressBarWidth).append('%');
String progressBarWithBuffer = progressBarBuffer.toString();
System.out.print("\r" + progressBarWithBuffer);
try {
Thread.sleep(50);
} catch (Exception e) {
e.printStackTrace();
}
currentTask++;
}
}
}
Output:
Progress Bar GUI using Java Swing library
In this method we will use Java Swing library and we will use JProgressBar from it to display our Progress bar in GUI.
import javax.swing.*; // Importing java swing library
public class ProgressBarGUI extends JFrame { // Making our class extends JFrame to inherit it's properties
JProgressBar jp; // Creating a new object from JProgress built-in class
int i = 0; // Initializing variable i
ProgressBarGUI() { // Making a constructor of our class
jp = new JProgressBar(0, 100); // Making progress bar counting from 0 to 100
jp.setBounds(40, 40, 160, 30); // Setting the width and height and position of our Progress bar
jp.setStringPainted(true); // Setting our counter to painted that it shows in our progress bar
setSize(250, 150); // Setting width and height of our frame
setLayout(null); // Setting layout manager to be null so that our progress bar manually resizes
add(jp); // Making our progress bar displayed
}
public void iterate() {
while (i <= 100) { // Iterating from 0 to 100, so that the progress bar counts from 0 to 100
jp.setValue(i); // sets the value of our progress bar counter to i
i += 1; // incrementing i each time by 1
try { // Making an exception for thread.sleep() method
Thread.sleep(150); // Making progress bar loop takes 150 Milliseconds per operation
} catch(Exception e) {
}
}
}
public static void main(String[] args) {
ProgressBarGUI p = new ProgressBarGUI(); // Creating a new object from our class ProgressBarGUI
p.setVisible(true); // to pop up our frame and progress bar
p.iterate(); // calling the iterate() method
}
}
Output:
With this article at OpenGenus, you have a strong idea of how to program a progress bar in Java using multiple methods like coding it easily without using strings, coding it using StringBuffer and StringBuilder and how to code it to show as a GUI.