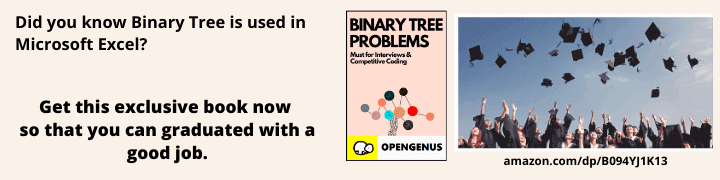
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
StringBuilder is a standard library class in Java which can be used in handling string data and is alternative to String Buffer and String data type.
The String class in Java creates an immutable object i.e once a string object is created it cannot be altered.To create a string which can be altered,String Builder Class is used,it creates a mutable sequence of characters.The java.lang.StringBuilder Class contains many methods which can perform various operations such as insert,append,trim,find index of certain character etc.
String Builder v/s String Buffer
String Builder Class and String Buffer Class have similar functionality except the fact that String Builder Class has no guarantee of synchronization whereas String Buffer Class does.By release of JDK5,String Builder was designed for a drop-in replacement for StringBuffer in places where the string buffer was being used by a single thread only.
If synchronization is not required then String Builder should be preferred over String Buffer as both of them supports same operations and String Builder is much faster as it does not guarantee synchronization.
NOTE:Instances of StringBuilder are not safe for use by multiple threads.In case synchronization is required StringBuffer should be used.
Ways to construct a StringBuilder object
- Using StringBuilder(): Constructs an object with no characters in it and an initial capacity of 16 characters.
StringBuilder str=new StringBuilder() //creates an object with no characters
System.out.println("StringBuilder Capacity= "+ str.capacity()); //will print 16 as initial capacity is 16
2.StringBuilder(int capacity): Constructs a string builder with no characters in it and an initial capacity specified by the capacity argument.
StringBuilder str2=new StringBuilder(10); //creates an object with no characters
System.out.println("StringBuilder2 capacity = "+ str2.capacity()); //will print 10 as 10 was specified as argument to the parameter:capacity
Note:This method will throw NegativeArraySizeException - if the capacity argument is less than 0.
3.StringBuilder(String str):Constructs an object initialized to the contents of the specified string.
StringBuilder str3=new StringBuilder("OpenGenus");
System.out.println("StringBuilder3 = "+ str3); //will print OpenGenus
4.StringBuilder(CharSequence seq):Constructs an object that contains the same characters as the specified CharSequence.
CharSequence phrase = "Cosmos";
StringBuilder buffer = new StringBuilder(phrase);
System.out.println(buffer); //prints Cosmos
Note:This method will throw NullPointerException - if argument sent to seq is null.
Java StringBuilder Methods
-
append(Data_type parameter_name):This method is used to append the string representation of the argument to the StringBuilder object.There are various overloaded append() methods available in the StringBuilder class. These methods are differentiated by the data type of their parameters.The data types are: boolean ,char , char[] ,CharSequence ,double ,float ,int ,long ,Object ,String and StringBuffer.Some of methods are listed down below:
1.append(boolean b):This method appends the string representation of the boolean argument to the StringBuilder object.
- Parameters: b - Data type of parameter is boolean.
- Returns:a reference to this object.
StringBuilder str=new StringBuilder("Cosmos "); System.out.println("StringBuilder = "+ str); str.append(true); //appends boolean argument System.out.println("After append StringBuilder is = "+ str);//prints Cosmos true
2.append(char c):This method appends the string representation of the char argument to to the StringBuilder object.
- Parameters:c - Data type of parameter is char.
- Returns:a reference to this object.
StringBuilder str=new StringBuilder("Cosmo"); System.out.println("StringBuilder = "+ str); str.append('s'); //appends 's' to str System.out.println("After append StringBuilder is = "+ str); //prints Cosmos
3.append(char[] str)
This method appends the string representation of the char array argument to the StringBuilder object.- Parameters:str - the characters to be appended.
- Returns:a reference to this object.
StringBuilder sb = new StringBuilder("OpenGenus_is_"); System.out.println("Before appending"+sb); char[] str = {'h','e','l','p','f','u','l'}; // appending char array argument sb.append(str); // print the StringBuilder after appending System.out.println("After appending = " + sb); //prints OpenGenus_is_helpful
4.append(Object obj):This method appends the String representation of the Object argument.
- Parameter:Object to be appended
- Returns:reference to the same StringBuilder object
StringBuilder str=new StringBuilder("Cosmos "); System.out.println("StringBuilder = "+ str); Object o="is amazing"; str.append(o); //appends object to string System.out.println("After append StringBuilder is = "+ str);//prints Cosmos is amazing
5.append(int i):This method appends the String representation of the Integer argument.
- Parameter:Integer to be appended
- Returns:reference to the same StringBuilder object
StringBuilder str=new StringBuilder("Cosmos "); System.out.println("StringBuilder = "+ str); int i=2021; str.append(i); //appends int argument System.out.println("After append StringBuilder is = "+ str);//prints Cosmos 2021
2.capacity():This method returns the current capacity of the StringBuilder object.
StringBuilder str2=new StringBuilder(10); //creates an object with 10 capacity
System.out.println("StringBuilder2 capacity = "+ str2.capacity()); // prints 10
3.length(): Returns the length (character count).
StringBuilder str2=new StringBuilder(10); //creates an object with 10 capacity
System.out.println("StringBuilder2 capacity = "+ str2.capacity()); // prints 10
str2.append("Cosmos");
System.out.println("StringBuilder2 length = "+ str2.length()); //len of string is 6
Note:The length() methods returns the actual size of the StringBuilder object whereas the capacity() method returns the maximum size that it can currently fit.Therefore,as seen in the above snippet,Capacity of object is 10 whereas length is 6.
4.charAt(int index):This method returns the char value in this sequence at the specified index.
- Parameter:index:the index of character to be returned
- Return value:Char value at specified index
StringBuilder str=new StringBuilder("Cosmos");
System.out.println("StringBuilder charAt(0) = "+ str.charAt(0)); //prints C
Note:The method will throw IndexOutOfBoundsException - if index is negative or greater than or equal to length().
5.deleteCharAt(int index):This method removes the char at the specified position given by index in the StringBuilder object.
- Parameters:index specifies position of char to be deleted
- Return Type:Same String Builder object after change is returned
StringBuilder str=new StringBuilder("Cosmos");
System.out.println("Before deletion = " + str);
str.deleteCharAt(0);
System.out.println("After deletion = "+ str); //prints osmos
Note:This method will throw StringIndexOutOfBoundsException - if the index is negative or greater than or equal to length().
6.delete(int start,int end):Removes the characters of the StringBuilder object specified by start(inclusive) to the end(exclusive).
- Parameters:start - the beginning index, inclusive and end - the ending index, exclusive.
- Returns:Same object after changes.
StringBuilder str=new StringBuilder("Cosmos");
System.out.println("Before deletion = " + str);
str.delete(1,6);
System.out.println("After deletion = "+ str); //prints C
Notes:
1.If end is greater than or equal to length of StringBuilder Object then characters till the end are removed.
2.Method throws StringIndexOutOfBoundsException - if start is negative, greater than length(), or greater than end.
7.indexOf(String str):This method returns the index within this string of the first occurrence of the specified substring.
- Parameter:str - any string
- Returns:int,if the str occurs as a substring within the StringBuilder object, then the index of the first character of the first substring observed is returned; if it does not occur as a substring, -1 is returned.
StringBuilder str=new StringBuilder("OpenGenus");
int ind1=str.indexOf("Cosmos"); //ind1=-1
int ind2=str.indexOf("Open"); //ind2= 0
System.out.println("ind1 "+ind1);
System.out.println("ind2 "+ind2);
Note:This method will throw NullPointerException - if str is null.
8.insert(int offset, X x):This method inserts the string representation of the argument into StringBuilder at the indicated offset.There are various overloaded insert() methods available in StringBuilder class X = boolean ,char ,char[] ,CharSequence ,double,float ,int,long ,Object ,String.Offset must greater than or equal to zero and less than or equal to length of StringBuilder object.
- Return Type:Same StringBuilder Object after changes.
StringBuilder str1=new StringBuilder("OpenGenus");
int i=2021;
str1.insert(9,i); //inserts int
System.out.println("after insert: "+str1);//OpenGenus2021
StringBuilder str2=new StringBuilder("OpenGenus");
float f=2021.111f;
str2.insert(9,f); //inserts float
System.out.println("after insert: "+str2);//OpenGenus2021.111
StringBuilder str3=new StringBuilder("OpenGenus");
double d=1999.1111d;
str3.insert(9,d); //inserts double
System.out.println("after insert: "+str3);//OpenGenus1999.1111
StringBuilder str4=new StringBuilder("OpenGenus is awesome:");
boolean b=true;
str4.insert(21,b); //inserts boolean
System.out.println("after insert: "+str4);//OpenGenus is awesome:true
StringBuilder str5=new StringBuilder("OenGenus");
char c='p';
str5.insert(1,c); //inserts char
System.out.println("after insert: "+str5);//OpenGenus
Object obj="Open";
StringBuilder str6=new StringBuilder("Genus");
str6.insert(0,obj); //inserts object
System.out.println("after insert: "+str6);//OpenGenus
CharSequence cs="Welcome ";
StringBuilder str7=new StringBuilder("to OpenGenus");
str7.insert(0,cs); //inserts CharSequence
System.out.println("after insert: "+str7);//Welcome to OpenGenus
Note:Method will throw StringIndexOutOfBoundsException - if the offset is invalid.
9.substring(int start):This method returns a new String,it contains characters which begin at the specified index(start) and extends to the end of the StringBuilder object.
- Parameters:start - starting index,it is inclusive.
- Returns: new string.
StringBuilder str=new StringBuilder("OpenGenus");
String new_string=str.substring(4);
System.out.println("New String :"+new_string); //Genus
Note:This method will throw StringIndexOutOfBoundsException - if start is less than zero, or greater than the length of the StringBuilder object.
10.substring(int start,int end):Returns a new String that contains a subsequence of characters contained in the String Builder object. The substring begins at the specified index:start and extends to the character at index end - 1.
- Parameters:
- start -starting index, inclusive.
- end - ending index,exclusive.
- Return type: String
StringBuilder str=new StringBuilder("xxxxOpenGenusxxxx");
String new_substring=str.substring(4,13);
System.out.println("New Substring : "+new_substring); //OpenGenus
Note:This method will throw StringIndexOutOfBoundsException - if end or start are negative or greater than length(), or start's value is greater than end's value.
11.toString():A new String object is allocated and contains the same character sequence which is present in StringBuilder object.
- Parameter:None
- Returns:A new string
StringBuilder str=new StringBuilder("OpenGenus");
String new_substring=str.toString();
System.out.println("New string : "+new_substring); //OpenGenus
System.out.println(new_substring.getClass()); //class java.lang.String
12.setCharAt(int index, char ch):This method sets the character at the specified index to ch.
- Parameters:
- index - the index of the character which is to be changed
- ch - new character
- Return type:void,as changes are made to the original StringBuilder object.
StringBuilder str=new StringBuilder("OpenXenus");
str.setCharAt(4,'G');
System.out.println("Changed StringBuilder : "+str); //OpenGenus
Note:This method throws IndexOutOfBoundsException - if index is greater than or equal to length() or negative.
Difference between String,String Buffer and String Builder
- String is immutable whereas StringBuffer and StringBuilder are mutable and support methods like insert() ,deleteCharAt() ,setCharAt() etc.
- String Builder is not synchronised whereas String Buffer is synchronised and is thread safe.
- String Builder is faster than String Buffer as it is not synchronized and thus avoids overhead of synchronization.