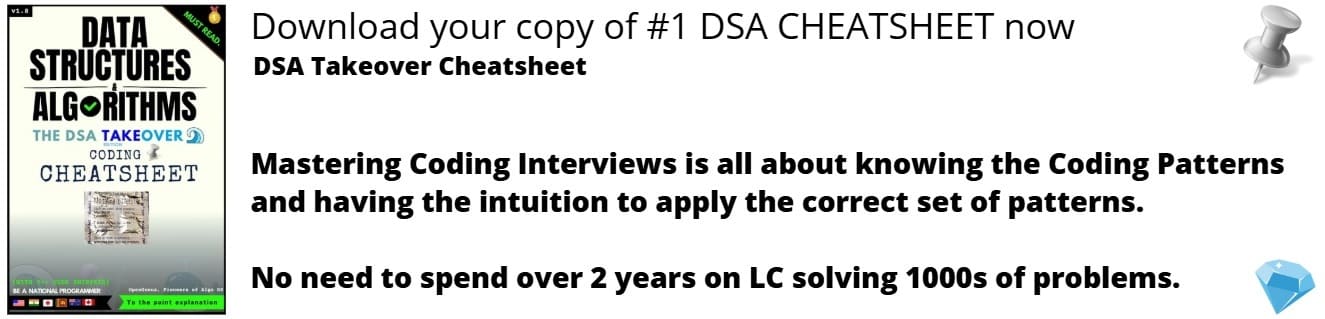
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of Contents:
- Number and Bit
- Set
- Clear
- Toggle
- Other ways of Implementation
- Conclusion
In this article, we will discuss about how to Set a bit, Clear a bit, and Toggle a bit of an integer in C++. Before doing so, we need to discuss about how number and bit are interpreted here.
Number and Bit
Given an integer N, when represented in its binary form, the kth bit of its binary value has to set, clear or toggle. to 1 if it is 0 and if it is 1, it will be unchanged.
Suppose,
N = 5
N2 = 1012
Now, if we map the bit of N2 to different value of k:
k | 3 | 2 | 1 |
N2 | 1 | 0 | 1 |
There is another concept of index. As C++ is 0 index based, the value of k and index is not the same. For example, the index of the bit of the binary form of number N will be started from 0, whereas the k is started from 1.
If we map k to index we will get:
k | 3 | 2 | 1 |
Index | 2 | 1 | 0 |
Mapping of k, Index and N2:
k | 3 | 2 | 1 |
Index | 2 | 1 | 0 |
N2 | 1 | 0 | 1 |
From the mapping table, we can identify that for k=1 we have to set, clear or toggle the bit of index=0. If we look at the other cases we will find that always for the kth bit, we have to set, clear or toggle the bit of k-1 index.
Set
Set indicates to setting a bit to 1 if it is 0 and unchanged if it is 1.
For the given example in Number and bit:
N = 5
N2 = 1012
Mapping of k, Index and N2:
k | 3 | 2 | 1 |
Index | 2 | 1 | 0 |
N2 | 1 | 0 | 1 |
If we set the kth bit = 2nd bit of N2, then N2 will be:
N2 | 1 | 1 | 1 |
N2 = 1002
N = 4
Code for Set
We can set the kth bit of N, if we left shift (<<
) bit 1 to the (k-1)th index and do a bitwise logical or (|) with the existing bit in that index of N.
#include <iostream>
using namespace std;
//function to set the k-th bit
//takes n and k as input
//returns the changed n after setting the k-th value
int setBit(int n, int k) {
return (n | 1 << (k-1)); //k-1: index number of the bit to set
}
int main(void) {
int n=5, k=2;
cout << n << " after setting " << k <<"-th value: " << setBit(n, k);
// output will be: 5 after setting 2-th value: 7
}
Clear
Clear indicates to set a bit to 0 if it is 1 and unchanged if it is 0.
For the given example in Number and bit:
N = 5
N2 = 1012
Mapping of k, Index and N2:
k | 3 | 2 | 1 |
Index | 2 | 1 | 0 |
N2 | 1 | 0 | 1 |
If we clear the kth bit = 1st bit of N2, then N2 will be:
N2 | 1 | 0 | 0 |
N2 = 1002
N = 4
Code for Clear
We can clear the kth bit of N, if we left shift (<<) bit 1 to the (k-1)th index, reset (~) it and do a bitwise logical and (&) with the existing bit in that index of N.
#include <iostream>
using namespace std;
//function to clear the k-th bit
//takes n and k as input
//returns the changed n after clearing the k-th value
int clearBit(int n, int k) {
return (n & (~(1 << (k-1)))); //k-1: index number of the bit to set
}
int main(void) {
int n=5, k=1;
cout << n << " after clearing " << k <<"-th value: " << clearBit(n, k);
// output will be: 5 after setting 1-th value: 4
}
Toggle
Toggle indicates to set a bit to 0 if it is 1 and 1 if it is 0.
For the given example in Number and bit:
N = 5
N2 = 1012
Mapping of k, Index and N2:
k | 3 | 2 | 1 |
Index | 2 | 1 | 0 |
N2 | 1 | 0 | 1 |
If we toggle the kth bit = 3rd bit of N2, then N2 will be:
N2 | 0 | 0 | 1 |
N2 = 0012
N = 1
Code for Toggle
We can toggle the kth bit of N, if we left shift (<<) bit 1 to the (k-1)th index and do a bitwise logical XOR (^) with the existing bit in that index of N. If we do a XOR operation gives the following output:
0 ^ 1 = 1
1 ^ 1 = 1
Variable bit | Given bit: 1 | XOR Value |
0 | 1 | 1 |
1 | 1 | 0 |
So, we can see XOR value toggles a bit (when XOR it with bit 1), returns the opposite bit.
#include <iostream>
using namespace std;
//function to toggle the k-th bit
//takes n and k as input
//returns the changed n after toggling the k-th value
int toggleBit(int n, int k) {
return (n ^ (1 << (k-1))); //k-1: index number of the bit to set
}
int main(void) {
int n=5, k=3;
cout << n << " after toggling " << k <<"-th value: " << toggleBit(n, k);
// output will be: 5 after toggling 3-th value: 1
}
Other ways of Implementation
Using Struct
We can hold the bits using struct and set, clear or toggle a specific bit directly.
#include <iostream>
using namespace std;
//declare a struct to hold the bits: value of each bit is 0 initially
struct bits {
unsigned int a:1;
unsigned int b:1;
unsigned int c:1;
unsigned int d:1;
unsigned int e:1;
unsigned int f:1;
unsigned int g:1;
int length;
};
int main(void) {
//create a instance of the struct
struct bits mybits;
//suppose n=11, binary(n)=1011
//declaring length to keep the track of the length of the bits
mybits.length = 4;
//assigning the bits 1011 in mybits
//as it is initialized with 0, I need to just set 3 digits
//to set the bit-value to 1
mybits.a = 1;
//to clear the bit-value to 0
mybits.b = 0;
//to toggle the value
mybits.c ^= 1;
//alternatives
//mybits.c = !mybits.c;
//mybits.c = ~mybits.c;
//setting the last bit
mybits.d = 1;
//now let's look at the changes
cout << "length: " << mybits.length << endl;
cout << "bits: " << mybits.a << mybits.b << mybits.c << mybits.d <<endl;
//output:
//length: 4
//bits: 1011
}
Using "define" keyword
We can use the define keyword, define the functions and use them.
#include <iostream>
//define the functions
#define BIT_SET(n, k) ((n) |= (1<<(k-1))) //set function
#define BIT_CLEAR(n, k) ((n) &= ~(1<<(k-1))) //clear function
#define BIT_TOGGLE(n, k) ((n) ^= (1<<(k-1))) //toggle function
int main(void) {
int n=5, k=2; //binary(n) = 100
printf("%d after setting %d-th value: %d\n", n, k, BIT_SET(n, k)); //n: 111 : 7
printf("%d after setting %d-th value: %d\n", n, k, BIT_CLEAR(n, k)); //n: 101 : 5
printf("%d after setting %d-th value: %d\n", n, k, BIT_TOGGLE(n, k)); //n: 111 : 7
//output
//5 after setting 2-th value: 7
//7 after setting 2-th value: 5
//5 after setting 2-th value: 7
}
Using header file
We can also use header file to store the define functions (macros) and use them by importing the header file in our code.
bits_manipulation.h:
//define the functions
#define BIT_SET(n, k) ((n) |= (1<<(k-1))) //set function
#define BIT_CLEAR(n, k) ((n) &= ~(1<<(k-1))) //clear function
#define BIT_TOGGLE(n, k) ((n) ^= (1<<(k-1))) //toggle function
use_bits_manipulation.cpp:
#include <iostream>
#include "bits_manipulation.h"
int main(void) {
int n=5, k=2; //binary(n) = 100
printf("%d after setting %d-th value: %d\n", n, k, BIT_SET(n, k)); //n: 111 : 7
printf("%d after setting %d-th value: %d\n", n, k, BIT_CLEAR(n, k)); //n: 101 : 5
printf("%d after setting %d-th value: %d\n", n, k, BIT_TOGGLE(n, k)); //n: 111 : 7
//output
//5 after setting 2-th value: 7
//7 after setting 2-th value: 5
//5 after setting 2-th value: 7
}
Using enum
We can also keep the number and our expected shift of values in the enum and use them to set, clear or toggle values. We do this limit the shifting and hide the number.
#include <iostream>
using namespace std;
enum Number_Shift {
Number = 5,
Shift_bit1_to_index1 = 1 << 1, //1 is shifted to the left by 0 position, value of other positions are 0
Shift_bit0_to_index2 = 0 << 2, //1 is shifted to the left by 1 position, value of all positions are 0 here
//index = k - 1 ; kth bit
};
int main() {
//Transfer Number to a variable of relevant datatype
int n = Number;
//set: k = 2; index = 1; binary(n) = 101
n |= Shift_bit1_to_index1; // 111 : 7
cout << n << endl;
//output: 7
//clear: k=2, index = 1; binary(n) = 111
n &= Shift_bit0_to_index2; // 000 : 0
cout << n << endl;
//output: 0
//clear: k=2, index = 1, binary(n) = 000
n ^= Shift_bit1_to_index1; // 010 : 2
cout << n << endl;
//output: 2
return 0;
}
Conclusion
In this article at OpenGenus, we have shown some implementations to set, clear and toggle bits. There are still many other ways like declaring class keeping the functions as methods and using the class and the methods etc. You can search the other methods online according to your need. Hope is that you have learned something new reading this article or it has solved your problem and/or you have enjoyed the reading.