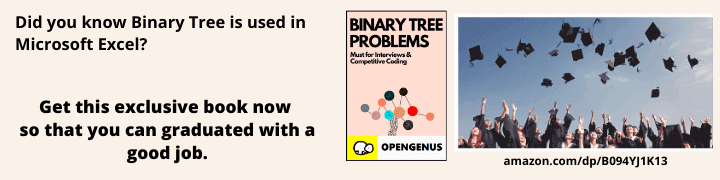
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 5-10 minutes | Coding time: 2 minutes
Introduction
In C++, a stack is a data structure that stores elements following Last-In-First-Out (LIFO) ordering. Before using stack::empty, you must first initialize a stack in your code. To allow their usage, the C++ standard library provides the stack class, which provides a list of functions and operations for working with stacks. In order to use stacks in C++, you must first include the stack header file at the beginning of your code:
#include <stack>
This will provide the contents necessary to use stack containers, and specifically stack::empty. Here is an example of initializing a stack of integers:
std::stack<int> mystack;
Stack::Empty
Among the available functions provided by the C++ standard library is the stack::empty function. Stack::empty is used to determine whether a given stack is empty. The function returns true if the stack is empty and false if it is not empty.
Let's analyze an example where stack::empty will return True:
#include <iostream>
#include <stack>
using namespace std;
int main() {
stack<int> mystack;
if (mystack.empty()){
cout << “True” << endl;
}
else {
cout << “False” << endl;
}
return 0;
}
In the above example, we initialize a stack and do not push any elements onto the stack. Because mystack does not contain any integers, the code will output True to indicate that it is empty.
Let's analyze an example where stack::empty will return False:
#include <iostream>
#include <stack>
using namespace std;
int main() {
stack<int> mystack;
mystack.push(3);
mystack.push(4);
if (mystack.empty()){
cout << “True” << endl;
}
else {
cout << “False” << endl;
}
return 0;
}
In the above example, we initialize a stack and push two elements onto the top of the stack. Since the stack contains the two integers 3 and 4, the code will output False to indicate that it is not empty.
Complexity
- Worst case time complexity:
Θ(1)
- Average case time complexity:
Θ(1)
- Best case time complexity:
Θ(1)
- Space complexity:
Θ(1)
Stack::empty performs a simple check of whether or not the stack is empty. It is not required to iterate over the elements of the stack or perform operations on those elements. Therefore it has O(1), or constant time complexity.
Real-World Applications
-
Webpage Navigation
A real-world example where the stack::empty function is extremely useful is in webpage navigation. On your web browser, there are arrows that allow you to navigate either to your previously visited web page or the next page you navigated to. If there is no webpage to navigate to, the arrow(s) will disabled, indicating that they cannot be used. This is where stack::empty is utilized. Stack::empty can be used to determine whether or not there are pages left that can be visited in either the previous or next webpages visited. If the previous history is empty, this means that the user is already on the earliest visited page. If the next history is empty, this means that there are no web pages the user has visited after the current one. -
Undo/Redo
Another example where stack::empty can be utilized is for undo/redo when typing and/or performing actions while text editing. Stack::empty is perfect for determining whether or not there are any available actions to undo or redo in their respective stacks.