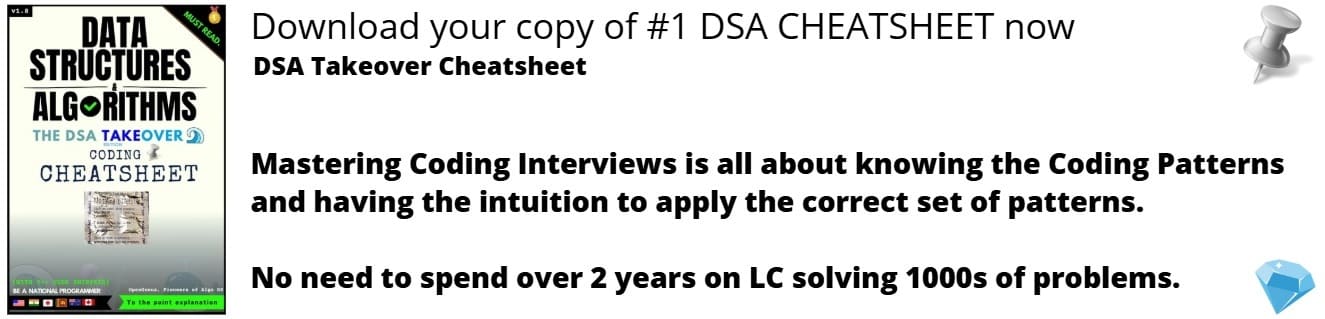
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored about Swapping of 2 variables. We have presented different methods to do so including swapping using third variable, using arithmetic operations, XOR, macro and much more along with their pros and cons.
Table of Contents
- Introduction to Swapping
- Using a third variable
- Using arithmetic operators + and -
- Using arithmetic operators * and /
- Using bitwise XOR operator ^
- Using a macro
- Other Methods
- Using a file as buffer
- Using a command-line-related variable as buffer
Introduction to Swapping
First we should have an idea about swapping.
The basic idea is to transfer the value of one variable to another and take the value of the second variable and put it to first
Example:
a = 6
b = 3
swap(a,b) //using a swap function
print("%d", a) //3
print("%d", b) //6
Note that value of variables a and b are interchanged after swapping.
Using a third variable
We create a third variable temp or temporary variable and we assign it's value as one of the variables to it. (eg. b)
This creates a copy of the b variable.
Now you can assign b to another variable, say a, without worrying about b losing it's value for the assignment of a, as it's already stored in the temp variable.
a = 6
b = 3
temp = b
b = a
a = temp
print("%d", a) //3
print("%d", b) //6
Note:
Many newcomers are confused over why the line
a = b
won't work.
After all a = b is the same as b = a in mathematics. Right?
What may be a valid statement in mathematics doesn't work the same way for variables in computers.
In computers, variables are containers that hold values, they are not the value themselves. A statement like, a = b will make the value stored in container a equal to the value in container b.
But the value stored in container b will be the same.
Using Operators + and -
This method is clever and gives the result without us having to create another variable.
This method might cause arithmetic overflow for large values though (if the first step exceeds the memory assigned)
a = 6
b = 3
a = a + b;
b = a - b;
a = a - b;
print("%d", a) //3
print("%d", b) //6
You can also use the + and - operators to swap the variables in a slightly different
way,
a = b - a;
b = b - a;
a = b + a;
Or you could do this to be compact,
a = (a + b) - (b = a);
Using Operators * and /
This method will not work if one or both variables contain 0.
It is also under the risk of arithmetic overflow for multiplying large numbers.
a = 6
b = 3
a = a * b;
b = a / b;
a = a / b;
printf("%d", a) //3
print("%d", b) //6
Or if you want to do it in a short way,
a = (a * b) / (b = a);
It will show undefined behavious if the first variable (a in the above example) is 0
Using bitwise XOR (^)
To understand this method, think of XOR as something that toggles the bits of numbers 0 and 1 when they are not the same.
We first store the XOR of the two variables in the first variable and then toggle them back for storing the original values in the variables.
a = 6
b = 3
a = a ^ b;
b = a ^ b;
a = a ^ b;
print("%d", a) //3
print("%d", b) //6
This method is especially elegant because we manage to swap the variables with only one operator and don't have to create another variable
You can make the above code a bit more compact by doing this,
a ^= b;
b ^= a;
a ^= b;
Or if you want to go further,
a ^= b ^= a ^= b;
Note:
When using pointers to the variables, the above methods 2, 3 and 4 will fail if both the pointers point to the same variable.
Example:
If a function to swap two variables is accepting the addresses of the two variables, then a call like swap(&a, &a); should make no effect on the value of variable a, but in the above methods (except the third variable method) the variable will store incorrect value.
So, we need to firstly check if both the pointers are exactly the same.
If you want to swap two pointers, a good example of doing that would be:
void swap(int *x, int *y) {
if(a != b) { // Checking that x and y are not pointing to the same location
*a ^= *b;
*b ^= *a;
*a ^= *b;
}
}
Using a macro
A macro is a segment of code which is replaced by the value of macro. Macro is defined by #define directive.
#define SWAP(a, b, Type) Type temp = a; a = b; b = temp;
int main() {
int a1 = 6, b1 = 3;
SWAP(a1, b1, int);
printf("After swapping: a1 = %d, b1 = %d", a1, b1); //After swapping: a1 =3, b1 = 6
return 0;
}
A nice thing about this method is you can use the same SWAP() function for int, char, float etc
In GCC we can make it even more generic,
#define SWAP(x, y) typeof(x) temp = x; x = y; y = temp;
This method is great if it's common for you to use the swap function a lot.
It can also be helpful in Competitive Programming where you have to be quick.
Other methods
These methods are included for purely theoretical purposes.
Using these is not feasible in most of the situations:
Using a file buffer
In this method we will write the value of a in a file (temp.txt) before assigning it the value of b.
Then b scans the value of temp.txt and it gets assigned.
FILE *fp;
fprintf(fp = fopen("temp.txt", "w"), "%d", a);
fclose(fp);
a = b;
fscanf(fp = fopen("temp.txt", "r"), "%d", &b);
fclose(fp);
Using a command-line-related variable as buffer
We use a command line argument to swap the values:
int main(int argc, char **argv) {
int a = 10, b = 5;
argc = a;
a = b;
b = argc;
printf("After swapping: a = %d, b = %d", a, b);
return 0;
}
Conclusion:
Swapping of 2 variables is one of the most common methods used in Algorithms. You may use depending on the situation, according to the situation considering the problem statement, resource constraints etc
Question
Go through this C++ code:
#include <stdio.h>
int main() {
int a = 6;
int b = 3;
a = b;
printf("%d, %d",a,b);
return 0;
}
What will be the output of the above code?
With this article at OpenGenus, you must have a strong idea of swapping two variables and will be able to choose the best technique depending on your use case.