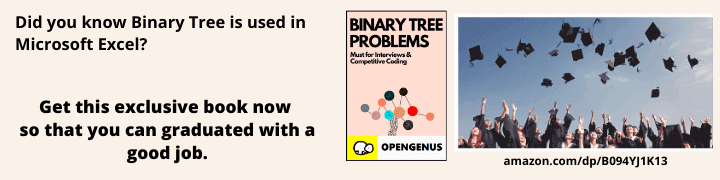
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will explore the algorithm to convert Decimal number to Octal number along with sample implementation.
Table of contents:
- Introduction to Decimal and Octal Numbers
- Steps for Conversion
- Step by Step Examples
- Code Implementation
Introduction to Decimal and Octal Numbers
Firstly we will understand what are decimal and octal numbers.
Decimal Numbers-
All the number having a base ten are called decimal numbers,it has digits from 0-9.
It has both integer and decimal part ,seperated by a decimal(.).
Example: (236.90)10, (52.25)10 etc.
Octal Numbers-
All the number having a base eight are called octal numbers.It has digits from 0-7.
Example: (236)8, (52)8 etc.
Steps for Conversion
Step 1- Write the given decimal number.
Step 2- If the given decimal number < 8 the octal number is the same as decimal number.
Step 3- If the decimal number > 7 then divide the number by 8.
Step 4- Write down the remainder, we get after division.
Step 5- Repeat step 3 and 4 with the quotient till it is < 8.
Step 6- Write the remainders in reverse order (bottom to top)
Step 7- The resultant is the equivalent octal number to the given decimal number.
Step by Step Examples
1. Input: (127) in base 10-
solution-
127/8=15 (Quotient) and 7 (remainder)
15/8=1 (Quotient) and 7 (remainder)
1/8=0(Quotient) and 1(remainder)
Now, as we get 0 as quotient then we can take remainders in reverse order to get the required octal number.
(127)10=(177)8
2. (210) in base 10-
solution-
210/8=26 (Quotient) and 2 (remainder)
26/8=3 (Quotient) and 2 (remainder)
3/8=0(Quotient) and 3(remainder)
Now, as we get 0 as quotient then we can take remainders in reverse order to get the required octal number.
(127)10=(322)8
For the decimal part we have two ways
1. Converting the remainders-
Steps to convert a fractinal decimal number are-
Step 1- Take decimal number as multiplicand.
Step 2- Multiple this number by 8.
Step 3- Store the value of integer part of result in an array.
Step 4- Repeat the above two steps until the number became zero.
Step 5- write the array .
Examples-
1.(0.0146890625)10-
solution-
0.140869140625 x 8=0.12695313 and 1(integer result)
0.12695313 x 8=0.01562504 and 1(integer result)
0.01562504 x 8=0.12500032 and 0(integer result)
0.12500032 x 8=0.0000025 and 1(integer result)
0.00000256 x 8=0.000020544 and 0(integer result)
result= (0.11010)8
2. Converting with division-
Steps to convert with help of division are-
Step 1- Start with decimal number and list the powers of 8.
Step 2- Divide the decimal number by the largest power of eight.
Step 3- Find the remainder and divide the remainder by the next power of 8.
Step 4- Repeat until you've found the full answer.
Example
1.(164)10-
solution-
powers of 8- 2 ,1 and 0
8^2=64
8^1=8
8^0=1
Then,
164/64=2.5625
taking the MSB 2 and remainder= 164- 642=36
36/8=4.5
taking the MSB 4 and remainder= 36- 84=4
4/8=0.5
taking the MSB 0 and remainder= 4- 8*0=4
Now the octal of the above number is (244)8.
Code Implementation
Code in C++
#include <iostream>
using namespace std;
void DToO(int dn) {
int on = 0, placeValue = 1;//octal numberand place value is taken 1
int dNo = dn;//stored in a temporary variable
while (dn != 0) {
on += (dn % 8) * placeValue;
dn /= 8;
placeValue *= 10;
}
cout<<"Octal form of decimal number "<<dNo<<" is "<<octalNum<<endl;//output
}
int main() {
DToO(127);//int num send
return 0;
}
Output-
Octal form of decimal number 127 is 177
With this article at OpenGenus, you must have the complete idea of how to convert decimal number to octal number.