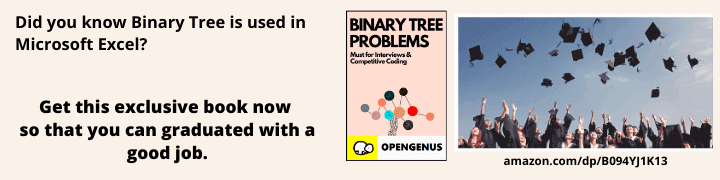
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have presented a method to convert Octal numbers into their Binary equivalents along with Java implementation.
Table of Contents:
- Introduction to Octal and Binary numbers
- Octal to Binary conversion
- Code Implementation
- MCQs on Number conversions
Introduction
The letters or characters typed by a human on a computer is transmitted in the form of numbers to the computer. A computer is capable of understanding only the positional number system that is in the form of symbols and digits. Now everything boils down to the binary number representation, that is quite vital in the computer world. Hence it is quite important to know the procedure behind converting a number of particular number system into a number of another specified number system. The most commonly used number systems are :
- Decimal Number System
- Binary Number System
- Octal Number System
- Hexadecimal Number System
Now in our case, we'll using the first three number systems i.e. Decimal , Binary and Octal. Let's take a quick look at the three number systems one by one
1. Decimal Number System :
The numbers that we use in our day to day activities are actually the decimal numbers. Decimal numbers have base 10 and uses 10 digits(0 to 9). Example : 20 , 100 , 256 etc
2. Binary Number System :
The following numbers comprise only of two digits 0 and 1. It is a base 2 number system. Each position in the binary number corresponds to some power of 2. Example : 11101 , 0101 , 11100 etc
3. Octal Number System:
The following comprise of digits between 0 and 7, hence the base is 8. Each digit in the octal number can be represented in some power of 8, therefore it is called Base 8 number system. Example : 12570 , 123456 etc
Octal to Binary Conversion
For converting a Octal number into binary number, you need to perform the following steps:
1. Convert the given Octal number into the corresponding Decimal number.
Let's understand it using an example, suppose the given octal number is 12570, here the number of digits is 5 so the last power up to which 8 will be raised is 4, therefore the corresponding decimal number will be :
((1x(8^4)) + (2x(8^3)) + (5x(8^2)) + (7x(8^1)) + (0x(8^0)))
Now the above equation boils down to :
(4096 + 1024 + 320 + 56 + 0)
hence the decimal number obtained is 5496
2. Now convert the obtained decimal number into the binary number
For converting a decimal number into the corresponding binary number, perform continuous division of the number by 2 and note the remainders. In our case the decimal number is 5496, so the corresponding binary number will be : 1010101111000
Hence the corresponding binary number for the octal number 12570 is 1010101111000
Now for checking the correctness of the result, you can convert the binary into the octal, if the octal number obtained is similar to the original value then the value is absolutely correct. To convert a binary number into the octal number just multiply each digit of the binary number with the corresponding powers of 8
In our case it will be :
1 x (8^12) + 0 x (8^11) + 1 x (8^10) + 0 x (8^9) + 1 x (8^8) + 0 x (8^7) + 1 x (8^6) + 1 x (8^5) + 1 x (8^4) + 1 x (8^3) + 0 x (8^2) + 0 x (8^1) + 0 x (8^0)
It boils down to 12570, which is exactly similar to the original octal number. Hence the obtained binary number is absolutely correct.
Code implementation
(A) Algorithm :
Step 1: START
Step 2: We will use to functions, toDecimal and toBinary. toDecimal for converting a octal number into decimal equivalent and toBinary to take the decimal output from the toDecimal function and then convert it into the binary equivalent.
Step 3: toDecimal method takes the octal number as the input parameter and then performs respective operation(discussed in Step 1 above) for converting to octal and decimal and then finally return it.
Step 4: toBinary method takes the decimal number from the toDecimal method as input parameter and then converts it into the binary equivalent using the operation(discussed in Step 2 above), then finally returns it in the form of string because a binary number can be very large.
Step 5: STOP
(B) Java Implementation
import java.util.Scanner;
public class opengenus_octal_to_binary {
public long toDecimal(long octal){
// In the following function, we're going to convert a given octal number into its decimal equivalent
// let's first calculate the number of digits in the given octal number
int count = 0;
long n = octal;
while(n > 0){
count++;
n = n / 10;
}
// Now convert it into the decimal equivalent
long decimal = 0;
for(int i = 0 ; i < count ; i++){
long d = octal % 10;
long power = (long)Math.pow(8 , i);
decimal = decimal + power * d;
octal = octal / 10;
}
return decimal;
}
public StringBuffer toBinary(long decimal){
// In the following method, we're goind to convert a given decimal number into its binary equivalent
StringBuffer binary = new StringBuffer();
while(decimal > 0) {
long d = decimal % 2;
if (d == 1)
binary = binary.append('1');
else
binary = binary.append('0');
decimal = decimal / 2;
}
return binary.reverse();
}
public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("Enter the octal number : ");
long octal = sc.nextLong();
opengenus_octal_to_binary obj = new opengenus_octal_to_binary();
StringBuffer binary = obj.toBinary(obj.toDecimal(octal));
System.out.println("Equivalent Binary Number : " + binary);
}
}
Output :
First Execution : Octal = 12570
1010101111000
Second Execution : Octal = 1356
1011101110
MCQs on Number conversions
1. The decimal equivalent of 1001 is :
(A) 9
(B) 8
(C) 7
(D) 10
Ans : A
2. The decimal equivalent of 11011101 :
(A) 121
(B) 221
(C) 441
(D) 256
Ans : B
3. The sum of 1111 + 1111 in binary equals to :
(A) 11110
(B) 10101
(C) 10101
(D) 01010
Ans : A
4. The base of decimal numbers minus binary numbers is :
(A) 8
(B) 2
(C) 5
(D) 6
Ans : A
5. An unknown number system uses 14 numbers (0 to 13) to represent its numbers, what is the base the unknown number system :
(A) 14
(B) 12
(C) 10
(D) 16
Ans : A
Thank You!