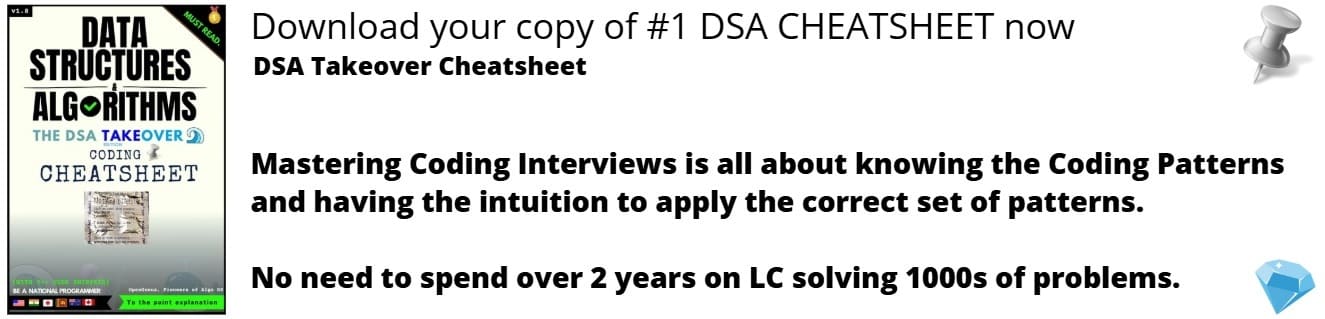
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have present the concept and algorithm to check if a given year is a leap year or not.
Introduction
A leap year is a year that comes after every 4 years. What makes leap years different is that it has 366 days instead of 365 days.
It takes the earth 365.242190 days to orbit the sun, or 365 days 5 hours 48 minutes and 56 seconds. But for simplicity, only the 365 whole days are considered as a calender year. If the remaining part of the year is not accounted for, over a period of centuries, it would start to affect the periodic change in season.
The cumulative of 0.25 days for four consecutive years is taken as one day which is then added to the fourth year, which makes it the leap year. The additional day is added to February as the 29th day of the month.
In a century, we are adding 25 extra days due to a leap year in every four year when in actuality we should add 24.2375 days. Thus, we are adding 0.7625 day extra in every 100-year. So, in span of four centuries, this error will become 3.05 days. Therefore, to avoid this accumulation error of 3.05 days in every four centuries, it was decided that out of four centuries only one will have 25 leap years while other will have 24 leap years. For this reason, not every four years is a leap year. The rule is that if the year is divisible by 100 and not divisible by 400, leap year is skipped.
The aim of this article is to build a program that would determine if the input year is a leap year or not.
Logic
For a year to be a leap year, it has to satisfy the following conditions:
- The year is a multiple of 400.
- The year is a multiple of 4 but not 100.
A century year is a year that is a multiple of 100 and ends with 00. For example, 1500 is a century year. The second condition is used to separate the century years from the leap years. A century year is considered to be a leap year only if it is divisible by 400. For instance, the years 1200, 1600, and 2000 are all century leap years. On the other hand, 1300, 1500, and 1900 are century years which do not qualify as leap year.
Pseudo-code
if year is divisible by 400
the year is leap year
if year is divisible by 4 but not by 100
the year is leap year
else
the year is not leap year
For better understanding of the pseudo-code, refer to the following flowchart
Execution
Python:
year = int(input('Enter year: '))
if(year % 400 == 0 or (year % 4 == 0 and year % 100 != 0)):
print(year,'is a leap year')
else:
print(year,'is not a leap year')
Java:
import java.util.*;
class LeapYear {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("Enter year: ");
int year = sc.nextInt();
if(year % 400 == 0 || (year % 4 == 0 && year % 100 != 0)){
System.out.println("\n"+ year + " is a leap year");
}
else{
System.out.println("\n"+ year + " is not a leap year");
}
}
}
C++:
#include <iostream>
using namespace std;
int main() {
int year;
cout << "Enter year: ";
cin >> year;
if(year % 400 == 0 || (year % 4 == 0 && year % 100 != 0)){
cout << year << " is a leap year";
}
else{
cout << year << " is not a leap year";
}
return 0;
}
Output:
Enter year: 2000
2000 is a leap year
With this article at OpenGenus, you must have the complete idea of how to check if a given year is a leap year or not.