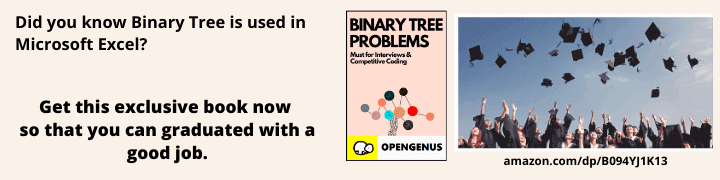
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we are going to discuss some key points of the functional requirements, non-functional requirements and many more technical aspects for the System Desgin of meeting schedule.
Table of contents:
- Functional Requirements
- Non-functional Requirements
- Projected Number of Users
- Database Design
- Low-Level Design and Classes
- Prospective System Design of Meeting Scheduler
- Conclusion
Functional Requirements
- User registration and authentication: Users should be able to register for an account and authenticate themselves to access the meeting scheduler system.
- Meeting scheduling: Users should be able to create, schedule, and manage meetings, including setting meeting details such as title, location, time, and duration.
- Meeting invitations and notifications: Users should be able to invite participants to meetings and send notifications to participants about meeting details, updates, and reminders.
- Calendar integration: The system should allow users to integrate their meeting schedules with their calendars (such as Google Calendar, Outlook Calendar, etc.) for seamless scheduling and reminders.
- Participant management: Users should be able to manage participants, including adding, removing, and updating participant details for each meeting.
- Meeting attendance tracking: The system should allow users to track meeting attendance, including marking participants as present, absent, or tentative.
- Meeting rescheduling and cancellation: Users should be able to reschedule or cancel meetings, with appropriate notifications sent to participants.
- Meeting history and archives: The system should maintain a history of past meetings and provide access to meeting archives for reference and reporting purposes.
- User roles and permissions: The system should support different user roles and permissions, such as administrators, organizers, and participants, with varying levels of access and privileges.
- Reporting and analytics: The system should provide reporting and analytics features, such as meeting attendance reports, meeting productivity metrics, and other relevant insights.
Non-functional Requirements
- Security: The system should ensure the confidentiality, integrity, and availability of meeting data, including user authentication, data encryption, and access control mechanisms.
- Performance: The system should be fast and responsive, with minimal downtime and optimal performance, even with a large number of users and meetings.
- Scalability: The system should be able to handle a growing number of users, meetings, and data, and scale seamlessly without any performance degradation.
- Usability: The system should have a user-friendly interface, with intuitive navigation, clear instructions, and simple interactions, to enhance user experience and adoption.
- Accessibility: The system should be accessible to users with disabilities, conforming to accessibility standards, such as WCAG 2.0, to ensure inclusivity.
- Integration: The system should be able to integrate with other tools, such as calendars, email clients, and collaboration platforms, to enable seamless workflow and productivity.
- Reliability: The system should be reliable, with backup and recovery mechanisms in place to prevent data loss and ensure system availability.
- Maintainability: The system should be easy to maintain, with regular updates, bug fixes, and performance optimizations, and proper documentation and training resources for users and administrators.
Projected Number of Users
The projected number of users for the meeting scheduler system may vary depending on the organization or target audience. For example, it could be used by small teams within an organization, or it could be a public-facing service used by a large number of users. The projected number of users will impact the system design in terms of scalability, performance, and other aspects.
Database Design
The database design for the meeting scheduler system may include the following tables:
-
User Table: This table stores user information, such as user ID, username, password (hashed), email, contact information, and user role.
-
Meeting Table: This table stores meeting information, such as meeting ID, title, description, location, start and end time, and participant list.
-
Participant Table: This table stores participant information for each meeting, such as participant ID, name, email, and attendance status.
-
Calendar Table: This table stores calendar information, such as calendar ID, user ID (foreign key), events, and availability status.
-
Notification Table: This table stores notification information, such as notification ID, recipient ID, notification type, content, and timestamp.
-
Log Table: This table stores system logs, including security events, user activities, and error logs, for auditing and troubleshooting purposes.
Low-Level Design and Classes
Here are some key classes that could be part of the system:
User Class: Represents a user of the system and stores user-related information such as username, password, email, and contact details. It may also have methods for user authentication, registration, and profile management.
Meeting Class: Represents a meeting and stores meeting-related information such as title, description, start time, end time, location, and participants. It may also have methods for scheduling a meeting, updating meeting details, and managing participants.
Participant Class: Represents a participant of a meeting and stores participant-related information such as name, email, and contact details. It may also have methods for adding, removing, and updating participant details.
Calendar Class: Represents a calendar and stores calendar-related information such as events, appointments, and reminders. It may have methods for adding, updating, and retrieving calendar events.
Notification Class: Represents notifications related to meetings and stores information such as recipient, message, and timestamp. It may have methods for sending notifications, managing notification settings, and handling notification events.
Authentication/Authorization Class: Handles user authentication and authorization, including methods for verifying user credentials, managing user roles and permissions, and handling authentication/authorization events.
Database Manager Class: Manages database operations such as CRUD (Create, Read, Update, Delete) operations for storing and retrieving data from the database, including methods for database connection, query execution, and data manipulation.
// User class
public class User {
private String username;
private String password;
private String email;
private String contactNumber;
// Constructors, getters, setters, and other methods for user-related operations
}
// Meeting class
public class Meeting {
private String title;
private String description;
private LocalDateTime startTime;
private LocalDateTime endTime;
private String location;
private List<Participant> participants;
// Constructors, getters, setters, and other methods for meeting-related operations
public void scheduleMeeting() {
// Logic for scheduling a meeting
}
public void updateMeetingDetails() {
// Logic for updating meeting details
}
public void manageParticipants() {
// Logic for managing participants of a meeting
}
}
// Participant class
public class Participant {
private String name;
private String email;
private String contactNumber;
// Constructors, getters, setters, and other methods for participant-related operations
}
// Calendar class
public class Calendar {
private List<Meeting> events;
// Constructors, getters, setters, and other methods for calendar-related operations
public void addEvent(Meeting meeting) {
// Logic for adding a meeting to the calendar
}
public void updateEvent(Meeting meeting) {
// Logic for updating a meeting in the calendar
}
public void retrieveEvents() {
// Logic for retrieving meetings from the calendar
}
}
// Notification class
public class Notification {
private String recipient;
private String message;
private LocalDateTime timestamp;
// Constructors, getters, setters, and other methods for notification-related operations
public void sendNotification() {
// Logic for sending a notification
}
public void manageNotificationSettings() {
// Logic for managing notification settings
}
public void handleNotificationEvents() {
// Logic for handling notification events
}
}
// Authentication/Authorization class
public class AuthenticationAuthorization {
// Methods for user authentication and authorization
public boolean authenticateUser(String username, String password) {
// Logic for authenticating a user
}
public void manageUserRolesAndPermissions() {
// Logic for managing user roles and permissions
}
public void handleAuthAuthorizationEvents() {
// Logic for handling authentication/authorization events
}
}
// Database Manager class
public class DatabaseManager {
// Methods for database operations such as CRUD operations
public void connectToDatabase() {
// Logic for connecting to the database
}
public void executeQuery(String query) {
// Logic for executing database queries
}
public void manipulateData(String query) {
// Logic for manipulating data in the database
}
public void disconnectFromDatabase() {
// Logic for disconnecting from the database
}
}
Prospective System Design
The prospective system design for the meeting scheduler system may involve the following components:
Front-end User Interface: This component includes the user interface components for the system, such as login and registration screens, meeting scheduling forms, participant management screens, and calendar views. It may be implemented using web technologies such as HTML, CSS, and JavaScript, or as a mobile app using native or cross-platform mobile development frameworks.
Back-end Server: This component handles the business logic and data processing of the system. It may be implemented using a server-side programming language such as Java, Python, or C#, and may utilize frameworks such as Spring, Django, or ASP.NET. The server communicates with the front-end UI, performs user authentication and authorization, manages meetings, participants, calendars, notifications, and logs, and interacts with the database.
Database Server: This component stores and manages the data of the system. It may use a relational database management system (RDBMS) such as MySQL, PostgreSQL, or Microsoft SQL Server, or a NoSQL database such as MongoDB or Cassandra, depending on the system's requirements for data storage and retrieval.
Third-party Services: The system may integrate with third-party services for functionalities such as email notifications, SMS notifications, calendar integration, and authentication/authorization. Examples of third-party services that may be used include Google Calendar API, Twilio for SMS notifications, and OAuth providers for authentication/authorization.
Security and Authentication/Authorization: This component handles system security, including user authentication and authorization, data encryption, protection against security vulnerabilities, and logging of security events. It may include components such as firewalls, SSL/TLS encryption, authentication/authorization middleware, and security auditing tools.
Testing and Deployment: This component includes testing and deployment processes for the system, such as unit testing, integration testing, system testing, and user acceptance testing. It may also include continuous integration/continuous deployment (CI/CD) pipelines for automated testing and deployment, and deployment strategies such as cloud-based hosting, containerization, or serverless architecture.
Conclusion
Overall, the system design for the meeting scheduler aims to provide a robust, secure, and user-friendly solution for scheduling and managing meetings, with scalability and flexibility to accommodate a projected number of users, and efficient data management through a well-designed database schema.