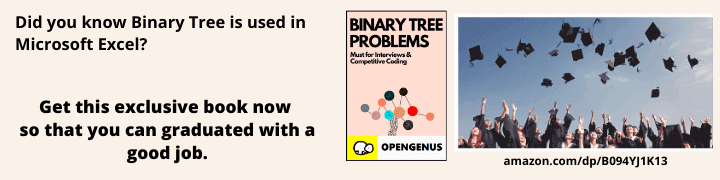
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we will discuss the system design of a train reservation system, covering the functional and non-functional requirements, projected number of users, database design, main class designs, and a prospective system design.
Table of Contents:
1.Functional Requirement
2.Non-functional Requirements
3.Projected Number of Users
4.Database Design
5.Low-Level Design and Classes
6.Tech Stack to be used
7.Prospective System Design
8.Conclusion
Functional Requirements
The functional requirements of a train reservation system typically include the following:
-
User registration: The system must allow users to create accounts and sign in with their credentials.
-
Train search: The system must allow users to search for trains based on various criteria, such as the departure and arrival stations, travel dates, and class of travel.
-
Seat availability: The system must allow users to check the availability of seats on a particular train for a specific date.
-
Ticket booking: The system must allow users to book tickets for their desired train and class.
-
Payment processing: The system must facilitate secure payment processing for ticket bookings.
-
Ticket cancellation: The system must allow users to cancel their booked tickets.
-
Booking history: The system must maintain a history of users' past bookings.
-
Train schedule: The system must display the schedules of trains, including their departure and arrival times.
Non-Functional Requirements
-
The non-functional requirements of a train reservation system are equally important and typically include:
-
Performance: The system must handle a large number of concurrent users without slowing down or crashing.
-
Scalability: The system must be designed to handle an increasing number of users and transactions over time.
-
Availability: The system must be available 24/7, with minimal downtime for maintenance or upgrades.
-
Security: The system must be secure and protect users' personal and financial information.
-
User experience: The system must be intuitive and easy to use, with a simple and responsive user interface.
Projected Number of Users
The projected number of users for a train reservation system will depend on various factors, including the size of the train network, the popularity of the service, and the marketing efforts of the company.
At the top china is served approximately 772.8Billion passanger-kilometer yearly. So it's most impacted transportation system.At the second highest number India is serving 770Billion passanger-kilometer annualy so for this country we required very reliable reseravtion system which can handle huge traffic at hte same time.
Database Design
The database design of a train reservation system must be carefully planned to ensure the efficient storage and retrieval of data. The system will typically require several tables to store information such as train schedules, seat availability, ticket bookings, user details, and payment transactions. The database must be designed to handle large amounts of data and provide fast access to information.
This database design can be used to store all the information needed for a train reservation system, including train schedules, customer information, and booking details. The relationships between the entities ensure that the data is consistent and accurate.
Here are some additional details about the entities and relationships:
The Train entity stores information about each train, such as the train name, number, source and destination stations, departure and arrival times, travel time, fare, and class of service.
The Customer entity stores information about each customer, such as their name, email address, phone number, and address.
The Booking entity stores information about each booking, such as the train, customer, number of tickets, class of service, fare, booking date, and status.
The Ticket entity stores information about each ticket, such as the booking, customer, train, seat number, class of service, fare, and status.
The Train entity has a one-to-many relationship with the Booking entity. This means that each train can have many bookings, but each booking can only be for one train.
The Customer entity also has a one-to-many relationship with the Booking entity. This means that each customer can make many bookings, but each booking can only be for one customer.
The Booking entity has a one-to-one relationship with the Ticket entity. This means that each booking can only have one ticket, and each ticket can only be for one booking.
Low-Level Design and Classes
In the low-level design of a train reservation system, the focus is on the specific implementation details of the system, including the classes and their interactions. Here, we will discuss the classes that are typically involved in a train reservation system and their responsibilities.
Train Class
The Train class represents the trains that are available for reservation. This class is responsible for managing the train's schedule, including its departure and arrival times, the available seats, and their respective prices. It should also be responsible for calculating the fare based on the number of seats booked and their prices. The Train class should also be responsible for updating the available seats after a successful booking.
public class Train {
private int trainId;
private String trainName;
private String source;
private String destination;
private Date departureTime;
private Date arrivalTime;
private int seats;
private double[] prices; // prices for different seat classes
public Train(int trainId, String trainName, String source, String destination, Date departureTime, Date arrivalTime, int seats, double[] prices) {
this.trainId = trainId;
this.trainName = trainName;
this.source = source;
this.destination = destination;
this.departureTime = departureTime;
this.arrivalTime = arrivalTime;
this.seats = seats;
this.prices = prices;
}
public int getAvailableSeats() {
// Returns the number of available seats on the train
return seats;
}
public void updateSeats(int numSeats) {
// Updates the number of available seats on the train
seats -= numSeats;
}
public double calculateFare(int numSeats, int seatClass) {
// Calculates the fare for the given number of seats and seat class
return numSeats * prices[seatClass];
}
}
User Class
The User class represents the users of the train reservation system. This class is responsible for managing user registration, authentication, and profile management. It should also be responsible for managing the user's booking history and any cancellations they make. Additionally, the User class should be responsible for validating the user's payment information before booking a ticket.
public class User {
private int userId;
private String username;
private String password;
private String email;
public User(int userId, String username, String password, String email) {
this.userId = userId;
this.username = username;
this.password = password;
this.email = email;
}
public boolean authenticate(String username, String password) {
// Authenticates the user with the given username and password
return this.username.equals(username) && this.password.equals(password);
}
public void register(String username, String password, String email) {
// Registers a new user with the given credentials
this.username = username;
this.password = password;
this.email = email;
}
public void updateProfile(String username, String password, String email) {
// Updates the user's profile with the given details
this.username = username;
this.password = password;
this.email = email;
}
public ArrayList<Booking> getBookingHistory() {
// Returns the user's booking history
return BookingDB.getBookingsByUser(userId);
}
public void cancelBooking(int bookingId) {
// Cancels the booking with the given ID
BookingDB.cancelBooking(bookingId);
}
public boolean validatePaymentInfo(PaymentInfo paymentInfo) {
// Validates the user's payment information
return PaymentProcessor.validatePayment(paymentInfo);
}
}
Booking Class
The Booking class represents a user's booking transaction. It should be responsible for storing the details of the booking, including the train, user, and seat information. This class should also be responsible for calculating the total fare of the booking and updating the Train class with the number of seats booked.
public class Booking {
private int bookingId;
private int trainId;
private int userId;
private int numSeats;
private int seatClass;
private double fare;
private Date bookingTime;
public Booking(int bookingId, int trainId, int userId, int numSeats, int seatClass, double fare, Date bookingTime) {
this.bookingId = bookingId;
this.trainId = trainId;
this.userId = userId;
this.numSeats = numSeats;
this.seatClass = seatClass;
this.fare = fare;
this.bookingTime = bookingTime;
}
public void saveBooking() {
// Saves the booking to the database
BookingDB.saveBooking(this);
}
public String getBookingDetails() {
String details = "Booking ID: " + bookingId + "\n"+ "Train Name: " +TrainDB.getTrainById(trainId).getTrainName()+ "\n"
+ "Source: " + TrainDB.getTrainById(trainId).getSource() + "\n"
+ "Destination: " + TrainDB.getTrainById(trainId).getDestination() + "\n"
+ "Departure Time: " + TrainDB.getTrainById(trainId).getDepartureTime() + "\n"
+ "Arrival Time: " + TrainDB.getTrainById(trainId).getArrivalTime() + "\n"
+ "Class: " + seatClass + "\n"
+ "Number of Seats: " + numSeats + "\n"
+ "Fare: " + fare + "\n"
+ "Booking Time: " + bookingTime + "\n";
return details;
}
}
In addition to these classes, there may also be additional classes for database access, payment processing, and user interface. The implementation of these classes will depend on the specific requirements of the system.
Tech Stack to be used
-
Server Operating System:
Linux distributions (e.g., Ubuntu, CentOS) are commonly used due to their stability, security, and cost-effectiveness. -
Web Application Framework:
Depending on the development language preference, frameworks such as Django (Python), Ruby on Rails (Ruby), or Laravel (PHP) can be used to streamline development. -
Database Management System (DBMS):
Relational databases like MySQL, PostgreSQL, or Oracle are often used to store reservation data, train schedules, user information, etc. -
Specifications of the Server
We can estimate the resources by following calculations:- The number of users who will be using the system at peak time.
- The average number of actions that each user will perform.
- The amount of memory required for each action.
WE expect 1000 users to be using the system at peak time, and each user will perform an average of 10 actions, and each action requires 1MB of memory, then the total memory requirement would be:
1000 users * 10 actions/user * 1MB/action = 10000MB
If WE expect the system to be able to handle 1000 concurrent users, then the total load at peak time would be:
1000 users * 10 actions/user = 10000 actions
So we required 10000 CPU threads at peak.
Prospective System Design
A prospective system design for a train reservation system could involve a microservices architecture, which allows for the creation of independent services that can communicate with each other through APIs. This architecture can provide greater scalability and flexibility than a monolithic system. Additionally, the system could use cloud infrastructure to improve availability and reduce maintenance costs. The user interface could be designed to be responsive and easy to use, with support for multiple languages and currencies.
Conclusion
A train reservation system is a complex and essential piece of software for the transportation industry. The system design must consider both the functional and non-functional requirements, the projected number of users, the database design, and the main class designs. The system must be scalabel and must be reliable for the users.The system should have several security protocol which wll protect the privacy of the users.