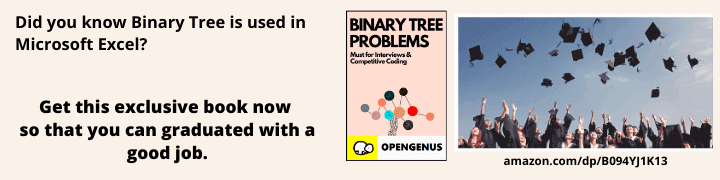
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 45 minutes
In this article, you will learn:
- How to declare variable in Koltin?
- How kotlin infers the type of variables?
- How to work with various data types?
Variables
As it is neccessary to learn about variables and their types before starting to code in any programming language. So, before learning about variable. Let's first understand what they are actually?
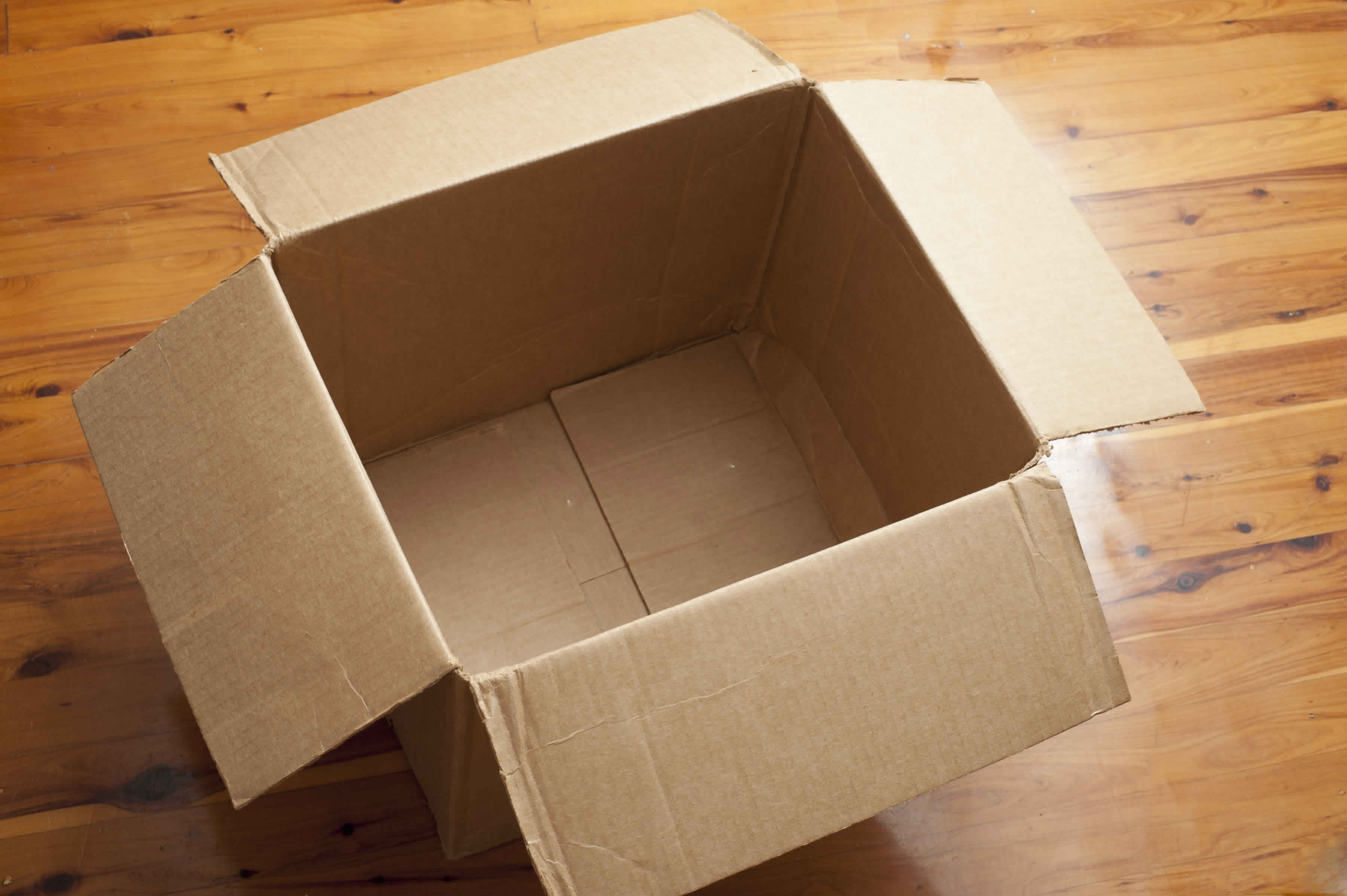
First for simplicity, You can think a variable is an empty box, which stores items or things in it.😋😋😊 That's it this is how variables work.
In programming, a variable is a location in memory to hold data.The type of a variable defines the range of values that the variable can hold, and the operations that can be done on those values.
Now, Question arises:
how to declare a variable in kotlin?
In kotlin we can either use:
- var keyword
- val keyword
Let's learn it by an example how you implement them:-
fun main() {
var IamString = "Hello Learners"
val IamInteger = 4
println("String : $IamString")
println("Number : $IamInteger")
}
Above Code is the presentation of how we can declare Variables in Kotlin. But you might get confused about what is difference Between var
and val
?
val
A variable declared using val keyword is immutable(read-only). It cannot be changed
after we intialized it example.,
Let, you intialize an variable num using val but when you try to change it's value then it shows an error:-
fun main() {
val num = 4
num = 5
println("Number : $num")
//Error: Val cannot be reassigned when you try to run.
}
So, Once val is intialized we can't change it's value.
var
A variable declared using var keyword is mutable. It can be changed after we initialized it.
we try to understand var by above example
Let again, you intialize an variable num using var but when you try to change it's value then it doesn't shows an error:-
fun main() {
var num = 4
num = 5
println("Number : $num")
//Works fine when you try to run.
}
Don't you think, While learning variable declaration we didn't specify the type of variable? Because kotlin implicitly does that for us. The complier knows already by intializer expression. This is called type inference in programming.
It's all on us we can define the type explicitly also.
But for a variable that doesn't intialized at the time of declaration or we want to use it later in our programme then we have to specify its type only then we are able to intialize that later in our programme.
fun main() {
var num
num = 5
println("Number : $num")
/*Error: This variable must either have a type annotation or be initialized*/
}
The above variable declaration fails because koltin has no way to infer the type of the variable without an initializer expression.
So, we can resolve error by giving a type annotation like
fun main() {
var num : Int
num = 5
println("Number : $num") //Works fine.
}
While naming variable remember that an identifiers may consist of letters, digits, and underscores, and may not begin with a digit.
var 1 = "Hello Learners" //shows error
That's all about var
and val
in kotlin.😊
Datatypes
Data Types are used to categorize a set of related values and define the operations that can be done on them.
Just like other languages, Kotlin has predefined types like Int, Double Boolean, Char etc.
Numbers
- Byte - 8 bit
- Short - 16 bit
- Int - 32 bit
- Long - 64 bit
Floating Point Numbers
- Float - 32 bit
- Double - 64 bit
1. Byte
It is used instead of Int or other integer data types to save memory if it's certain that the value of a variable will be within [-128, 127].
fun main() {
var iAmByte : Int = 4
println("$iAmByte")
}
Output:
4
Above code gives error when input exceeds range.
2. Short
It is used instead of other integer data types to save memory if it's certain that the value of a variable will be within [-32768, 32767].
fun main() {
var iAmShort : Short = -10454
println("$iAmShort")
}
Output:
-10454
3. Int
The Int data type can have values from -231 to 231-1 (32-bit signed two's complement integer).
fun main() {
var iAmInt : Int = 104542
println("$iAmInt")
}
Output:
104542
4. Long
The Int data type can have values from -263 to 263-1 (64-bit signed two's complement integer).
fun main() {
var iAmLong : Long = 100000000
println("$iaLong")
}
Output
100000000
You can also define Long as:
var iAmLong = 100000000L
// you can use capital
// letter L to specify that the
// variable is of type Long.
output will be same.
5. Double
The Double type is a double-precision 64-bit floating point.
fun main() {
var iAmDouble : Double = 13343.23
println("iAmDouble")
}
Output:
13343.23
6. Float
The Float data type is a single-precision 32-bit floating point.
fun main() {
var iAmFloat = 1343.23F
println("$iAmFloat")
}
Output:
1343.23
7. char
Characters are represented using the type Char
. Unlike Java, Char types cannot be treated as numbers. They are declared using single quotes like this :
fun main() {
var iAmChar : Char = 'a'
println("$iAmChar")
}
Output:
a
8. Booleans
Used to represent Logical values. It can have only two possible values either true
or false
.
fun main() {
var boolean : Boolean = true
println("$boolean")
}
Output:
true
9. Characters
Characters are represented using the type Char. Char
.
fun main() {
var iAmChar : Char = 'H'
println("$iAmChar")
}
Output:
H
10. Strings
Strings are represented using the class String
. They are immutable, that means you cannot modify a String by changing some of its elements.
fun main() {
var iAmStr : String = "Hello Learners"
println("$iAmStr")
}
Output:
Hello Learners
Few Important Points about Strings :
- Strings are Immutable, Means that you cannot modify a String by changing some of its elements.
- we can access the character at a particular index in a String using
str[index]
.
Some Additional Information:
- We can also use underscore in numeric values to make them more readable -
val hundredThousand = 100_000
val oneMillion = 1_000_000
We can declare hexadecimal and binary values like this -
val myHexa = 0x0A0F
val myBinary = 0b1010
- Hexadecimal values are prefixed with '0x' or '0X'
- Binary values are prefixed with '0b' or '0B'
Important :- Kotlin doesn’t have any representation for octal values.
That's all about Variables and Datatypes in Kotlin. Hope you Learned a Lot !