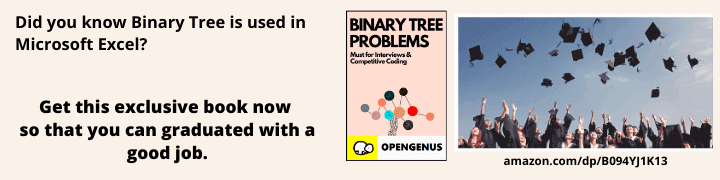
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Java is an Object Oriented Programming language and supports the feature of inheritance. We cannot have Multiple Inheritance in Java directly due to Diamond Problem but it can be implemented using Interfaces. We have explained this in detail starting with basic introduction to inheritance.
Inheritance is inheriting the properties of one class(Parent class) in the another class(child class). This relation can be defined by using the extends keyword as −
public class child extends parent{
}
The class which inherits the properties is known as sub class or child class and the class whose properties are inherited is super class or parent class. In inheritance a copy of parent class members is created in the child class object. Therefore, using the child class object you can access the members of the both classes.The various types of inheritance in java are :-
- Single
- Multilevel
- hierarchical
- Multiple
- Hybrid
Multiple inheritance is inheriting properties of two or more parent classes to one child class.As given in the below diagram class A and class B is being inherited by the child class C.
Sample code of how multiple inheritance works in java is given below. First two classes are made ParentA and ParentB and they both have same signature methods walk() . a child class is made which is inheriting both the classes by using a keyword 'extends'.
//first parent class
class ParentA
{
//same signature method in both class named as walk()
void walk()
{
System.out.println("Parent A is walking");
}
}
//second parent class
class ParentB
{
void walk()
{
System.out.println("Parent B is walking");
}
}
//multiple inheritance in child class results in error
class child extends ParentA, ParentB
{
public static void main(String[] args)
{
child object = new child();
object.walk();
}
}
Output :
Compile time Error
In the above code the compiler will get confuse which method to call when object of child class calls walk() method and results in compile time error.
Hence, Java does not support multiple inheritance because it can lead to increased complexity and ambiguity in case of 'Diamond Problem' which means that when classes with same signature in both the parent classes are made and child class when on calling the method makes the compiler cannot determine which class method to be called and this causes diamond problem and gives the compile time Error.
A picture depicting diamond problem is shown below. Where grandparent class A is being inherited by two class B And class C which further us getting inherited by the child class D.
How diamond problem is coded in java is shown below.
//parent-parent means grandparent class A
class GrandParentA
{
void walk()
{
System.out.println("grandparent is walking ");
}
}
//first parent class
class ParentB extends GrandParentA
{
//same signature method in both class named as walk()
void walk()
{
System.out.println("Parent A is walking");
}
}
//second parent class
class ParentC extends GrandParentA
{
void walk()
{
System.out.println("Parent B is walking");
}
}
//multiple inheritance in child class results in error
class child extends ParentA, ParentB
{
public static void main(String[] args)
{
child object = new child();
object.walk();
}
}
}
Output :
Error :
multipleinheritance.java:25: error: '{' expected
class child extends ParentA, ParentB
^
1 error
in the above code there is GrandParentA class which is inherited by two classes ParentA and ParentB and then both the classes are being inherited by child class and this leads to Diamond problem because compiler will get confuse which method to call when object of child class calls walk() method as both the parent classes have same signature name method and results in compile time error due to diamond problem.
The solution to this problem of Multiple inheritance is using Interface
Interface is a collection of abstract methods(non-defined methods). A class implements an interface, hence inheriting the abstract methods of the interface. An interface may also contain constants, Variables, default methods, static blocks, methods etc.
the syntax of interface is given below :-
// interface
interface Parent {
public void walk(); // interface method (does not have a body)
public void run(); // interface method (does not have a body)
}
as we have studied above to inherit a class in a class we use extends keyword similarily to inherit an interface in a interface we use extends keyword but when we are inheriting the interface in the class we use implements keyword.To easily understand this concept see the below picture.
hence , to perform multiple interfaces in a class we will be using a keyword 'implements'.
Below is an example of where multiple inheritance is achieved with the help of interfaces.
//interface 1
interface ParentA
{
//interfaces method are only declared not defined
public void walk();
}
//interface 2
interface ParentB
{
//any number of methods can be declared in the interface
public void walk();
public void run();
}
//multiple inheritance achieved
class child implements ParentA,ParentB
{
//overidden methods
public void walk()
{
System.out.println("ParentA is walking ");
}
public void run()
{
System.out.println("ParentB is running ");
}
public static void main (String args[])
{
child object = new child();
object.walk();
object.run();
}
}
output:
ParentA is walking
ParentB is running
hence, in the above code we have achieved Multiple inheritance in java with the help of interfaces where we have declared our methods in the interfaces and overridden them in the child class. (override means when a method that has already been declared in parent class is being defined again in the child class having same name, number and type of parameters, and return type as the method that it overrides) so, we have re-implemented the walk() and run() method of parent class in the child class to use them. When the object of child class will call method they will be called without getting ambiguity and will print the output.
Conclusion :-
Java does not support "multiple inheritance" (a class can only inherit from one parent class). However, it can be achieved with help of interfaces, because the class can implement multiple interfaces.
Question
Which problem arises due to multiple inheritance which increases complexity and ambiguity
With this article at OpenGenus, you must have the complete idea of Multiple Inheritance in Java. Enjoy.