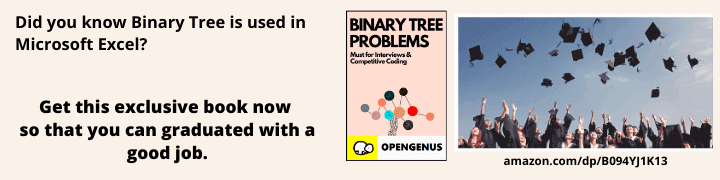
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 15 minutes
In this article, we will explore the types of inheritance that are supported and not supported in Java with code examples.
Inheritance,one of the most important thing in object oriented programming.
Inheritance is the capability of one class of things to inherit properties from another class
Lets take another example to understand this topic. The class Car inherits some of its properties from the class Automobiles, which inherits some of its properties from the class Vehicles.The capability to pass down properties is a powerful one.
Syntax
extends keyword is used in java to inherit classes.
In this example, we class B inherits class A. The object of class B will have access to member variables and functions in class A depending on access specifiers.
class A
{
...
}
class B extends A
{
...
}
Types of Inheritance
In short, Java does not support inheriting multiple classes. This is to avoid some of the undefined behaviour that can arise if such situations are not taken into consideration. To avoid this issue, java does not support this and this is not a limitation.
Hence, Java does not support Multiple inheritance and Multipath inheritance.
Java supports single inheritance, hybrid inheritance, hierarchical inheritance and multilevel inheritance.
Single inheritance
Single inheritance: When a subclass inherits only from one base class, it is known as single inheritance.
Consider the following example:
Class A
{
public void methodA()
{
System.out.println("Base class method");
}
}
Class B extends A
{
public void methodB()
{
System.out.println("Child class method");
}
public static void main(String args[])
{
B obj = new B();
obj.methodA(); //calling super class method
obj.methodB(); //calling local method
}
}
Output:
Base class method
child class method
Multiple inheritance
Multiple inheritance: When a subclass inherits from multiple base classes, it is known as multiple inheritance. Java does not support multiple inheritance i.e we cannot inherit properties from two classes.
Multipath inheritance
Java does not support Multipath inheritance.
This is because in this, we inherit two different classes which is not possible in Java.
Hierarchical inheritance
Hierarchical inheritance: When many subclasses inherit from a single base class, it is known as hierarchical inheritance.
Consider the following example:
class A
{
public void methodA()
{
System.out.println("method of Class A");
}
}
class B extends A
{
public void methodB()
{
System.out.println("method of Class B");
}
}
class C extends A
{
public void methodC()
{
System.out.println("method of Class C");
}
}
class D extends A
{
public void methodD()
{
System.out.println("method of Class D");
}
}
class JavaExample
{
public static void main(String args[])
{
B obj1 = new B();
C obj2 = new C();
D obj3 = new D();
//All classes can access the method of class A
obj1.methodA();
obj2.methodA();
obj3.methodA();
}
}
Output:
method of Class A
method of Class A
method of Class A
Multilevel inheritance
Multilevel inheritance: The transitive nature of inheritance is reflected by this form of inheritance.when subclass inherits from a class that itself inherits from another class, it is known as multilevel inheritance.
Consider the following example:
Class A
{
public void methodA()
{
System.out.println("Class A method");
}
}
Class B extends A
{
public void methodB()
{
System.out.println("class B method");
}
}
Class C extends B
{
public void methodC()
{
System.out.println("class C method");
}
public static void main(String args[])
{
C obj = new C();
obj.methodA(); //calling grand parent class method
obj.methodB(); //calling parent class method
obj.methodC(); //calling local method
}
}
Output
class A method
class B method
class C method
Hybrid inheritance
Hybrid inheritance: When subclass inherits from multiple base classes and all of its base classes inherit from a single base class,then this form of inheritance is known as hybrid inheritance.
Consider the following example:
class C
{
public void disp()
{
System.out.println("C");
}
}
class A extends C
{
public void disp()
{
System.out.println("A");
}
}
class B extends C
{
public void disp()
{
System.out.println("B");
}
}
class D extends A
{
public void disp()
{
System.out.println("D");
}
public static void main(String args[]){
D obj = new D();
obj.disp();
}
}
Output
D