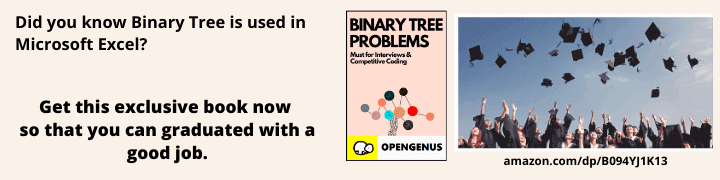
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
We will explore 3D Vectors in C++ in depth.
Vector is used in C++ to store items in consecutive memory locations dynamically. We can resize the vector in between program execution.
Vector is part of C++ Standard template library (STL library).
1D vector contains multiple elements,
2D vector contains multiple 1D vectors as rows and
3D vector contains multiple 2D vectors.
Therefore, we can say that 3D vector is vector of vector of vector.
Initializing 3D Vector
1. Normal Initialization:
Generally we initialize 3D vector using following syntax
Syntax:
vector<vector<vector< data_type >>> vector_name;
Example:
#include<iostream>
#include<vector>
using namespace std;
int main()
{
//initializing 3D Vector
vector<vector<vector<int>>> vector_3d;
return 0;
}
2. Initialization 3D Vector with given size:
For initializing 3D Vector with given size we use following syntax:
vector<vector<vector< data_type >>> vector_name( size );
//size = number of elements (number of 2d vectors that we want)
Example:
#include<iostream>
#include<vector>
using namespace std;
int main()
{
//initializing 3D vector with size 4 (contains 4 2D vectors)
vector<vector<vector<int>>> vector_3d(4);
return 0;
}
3. Initialize 3D vector with given size with same value:
Creating a 3D Vector with given size and initializing each element with given value.
Syntax:
vector<vector<vector<int>>> vector_3d(z, vector<vector<int>>(y, vector<int>(x, value)));
// x = number of elements in 1D vector
// y = number of 1D vectors in 2D vector
// z = number of 2D vectors in 3D vector
// value = value for each element
Example:
#include<iostream>
#include<vector>
using namespace std;
int main()
{
//Initializing a 3D vector (name = vector_3d )with dimensions (2, 3, 4) and initializing each element with 1
vector<vector<vector<int>>> vector_3d(2, vector<vector<int>>(3, vector<int>(4, 1)));
//Printing 3D vector
for(int i=0;i<vector_3d.size();i++)
{
for(int j=0;j<vector_3d[i].size();j++)
{
for(int k=0;k<vector_3d[i][j].size();k++)
{
cout<<vector_3d[i][j][k]<<" ";
}
cout<<endl;
}
cout<<endl;
}
return 0;
}
Output:
1 1 1 1
1 1 1 1
1 1 1 1
1 1 1 1
1 1 1 1
1 1 1 1
Insert elements in 3D vector
1. Insert Elements Using push_back()
:
push_back()
function in vector is used for inserting a element at its end.
For creating 3D vector we need to follow following steps:
- Declaring 3D vector
- Declaring 2D vector
- Declaring 1D vector
- Pushing elements in 1D vector using
push_back()
- Pushing that 1D vectors in 2D vector using
push_back()
- Pushing that 2D vectors in 3D vector using
push_back()
- repeating this to create 3D vector with multiple 2D vector.
Example:
#include<iostream>
#include<vector>
using namespace std;
int main()
{
//initializing 3D vector
vector<vector<vector<int>>> vector_3d;
int x=2, y=3, z=4;
//inserting elements
for(int i=0;i<x;i++)
{
vector<vector<int>> v2d;
for(int j=0;j<y;j++)
{
vector<int> v1d;
for(int k=0;k<z;k++)
{
v1d.push_back(k);
}
v2d.push_back(v1d);
}
vector_3d.push_back(v2d);
}
//printing vector
for(int i=0;i<x;i++)
{
for(int j=0;j<y;j++)
{
for(int k=0;k<z;k++)
{
cout<<vector_3d[i][j][k]<<" ";
}
cout<<endl;
}
cout<<endl;
}
return 0;
}
Output:
0 1 2 3
0 1 2 3
0 1 2 3
0 1 2 3
0 1 2 3
0 1 2 3
2. Insert elements like Arrays:
In C++ arrays we can initialize arrays using curly brackets.
ex:
int arr = {1,2,3};
just like that we can also insert elements 3D Vectors.
Example:
#include<iostream>
#include<vector>
using namespace std;
int main()
{
//initializing 3d vector like array
vector<vector<vector<int>>> vector_3d = {{{1,2}, {2,3}, {3,4}},
{{1,2}, {2,3}, {3,4}},
{{1,2}, {2,3}, {3,4}}};
//printing elements
for(int i=0;i<vector_3d.size();i++)
{
for(int j=0;j<vector_3d[i].size();j++)
{
for(int k=0;k<vector_3d[i][j].size();k++)
{
cout<<vector_3d[i][j][k]<<" ";
}
cout<<endl;
}
cout<<endl;
}
return 0;
}
Output:
1 2
2 3
3 4
1 2
2 3
3 4
1 2
2 3
3 4
Traversing in 3D Vector
Traversing means going through each elements of 3D vector or printing each element.
1. Using normal for
loop :
For printing elements of vector using for
loop we need to execute loop till the counting variable is less then size of vector.
we can get size of vector using size()
.
#include<iostream>
#include<vector>
using namespace std;
int main()
{
//initializing 3d vector
vector<vector<vector<int>>> vector_3d = {{{1,2}, {2,3}, {3,4}},
{{1,2}, {2,3}, {3,4}},
{{1,2}, {2,3}, {3,4}}};
//printing elements of vector
for(int i=0;i<vector_3d.size();i++)
{
for(int j=0;j<vector_3d[i].size();j++)
{
for(int k=0;k<vector_3d[i][j].size();k++)
{
cout<<vector_3d[i][j][k]<<" ";
}
cout<<endl;
}
cout<<endl;
}
return 0;
}
Output:
1 2
2 3
3 4
1 2
2 3
3 4
1 2
2 3
3 4
2. Using for
loop and iterators
:
Iterators are used to point out memory address of STL template class container.
We can use iterators to print each elements of 3D Vectors.
Example:
#include<iostream>
#include<vector>
using namespace std;
int main()
{
//initializing 3d vector
vector<vector<vector<int>>> vector_3d = {{{1,2}, {2,3}, {3,4}},
{{1,2}, {2,3,5}, {3,4}},
{{1,2}, {2,3}, {3,4,6,7}}};
//printing elements of vector
for(auto itr_i=vector_3d.begin(); itr_i!=vector_3d.end(); ++itr_i)
{
for(auto itr_j=(*itr_i).begin(); itr_j!=(*itr_i).end(); ++itr_j)
{
for(auto itr_k=(*itr_j).begin(); itr_k!=(*itr_j).end(); ++itr_k) cout<<*itr_k<<" ";
cout<<endl;
}
cout<<endl;
}
return 0;
}
Output:
1 2
2 3
3 4
1 2
2 3 5
3 4
1 2
2 3
3 4 6 7
We can create 3D vector with internal 2D vectors and 1D vectors with different dimensions
Using enhanced for
loop:
It is nothing but just a little modification to for loop for printing or traversing in 3D vector.
Example:
#include<iostream>
#include<vector>
using namespace std;
int main()
{
//initializing 3d vector
vector<vector<vector<int>>> vector_3d = {{{1,2}, {2,3}, {3,4}},
{{1,2}, {2,3}, {3,4}},
{{1,2}, {2,3}, {3,4}}};
//printing elements
for(vector<vector<int>> i : vector_3d)
{
for(vector<int> j: i)
{
for(int k: j)
{
cout<<k<<" ";
}
cout<<endl;
}
cout<<endl;
}
return 0;
}
Output:
1 2
2 3
3 4
1 2
2 3
3 4
1 2
2 3
3 4
Deleting elements in 3D Vector
1. Deleting elements using pop_back()
:
In C++ vectors pop_back()
method us used for deleting last element of vector.
In same way in our 3D vector we can use that to delete last 2D vector, last 1D vector or last element of 1D vector.
#include<iostream>
#include<vector>
using namespace std;
int main()
{
//initializing 3D vector
vector<vector<vector<int>>> vector_3d = {{{1,2}, {2,3}, {3,4}},
{{1,2}, {2,3}, {3,4}},
{{1,2}, {2,3}, {3,4}}};
vector_3d.pop_back(); //popping last 2D vector
vector_3d[1].pop_back(); //popping last 1D vector (last row) of 2nd 2D vector
vector_3d[1][1].pop_back(); //popping last element of last row of 2nd 2D vector
for(int i=0;i<vector_3d.size();i++)
{
for(int j=0;j<vector_3d[i].size();j++)
{
for(int k=0;k<vector_3d[i][j].size();k++)
{
cout<<vector_3d[i][j][k]<<" ";
}
cout<<endl;
}
cout<<endl;
}
return 0;
}
Output:
1 2
2 3
3 4
1 2
2
2. Deleting elements using erase()
:
In vector C++ erase()
is used to remove specific element or range of elements.
In same way in our 3D vector we can use that for erasing any 2D vector, 1D vector or any element of 1D vector.
#include<iostream>
#include<vector>
using namespace std;
int main()
{
//initializing 3D vector
vector<vector<vector<int>>> vector_3d = {{{1,2}, {2,3}, {3,4}},
{{1,2}, {2,3}, {3,4}},
{{1,2}, {2,3}, {3,4}}};
//erasing 2nd element of 3rd row in 2nd 2D vector
vector_3d[1][2].erase(vector_3d[1][2].begin()+1);
for(int i=0;i<vector_3d.size();i++)
{
for(int j=0;j<vector_3d[i].size();j++)
{
for(int k=0;k<vector_3d[i][j].size();k++)
{
cout<<vector_3d[i][j][k]<<" ";
}
cout<<endl;
}
cout<<endl;
}
return 0;
}
Output:
1 2
2 3
3 4
1 2
2 3
3
1 2
2 3
3 4
3. Deleting using clear()
:
clear()
is used to delete the whole vector or delete all the spaces taken by elements.
It's not mandatory to clear a vector after executing a program, the destructor method of vector template class will automatically clear the vector after program completion.
#include<iostream>
#include<vector>
using namespace std;
int main()
{
//initializing 3D vector
vector<vector<vector<int>>> vector_3d = {{{1,2}, {2,3}, {3,4}},
{{1,2}, {2,3}, {3,4}},
{{1,2}, {2,3}, {3,4}}};
vector_3d.clear(); //clearing 3D vector
//printing each elements
for(int i=0;i<vector_3d.size();i++)
{
for(int j=0;j<vector_3d[i].size();j++)
{
for(int k=0;k<vector_3d[i][j].size();k++)
{
cout<<vector_3d[i][j][k]<<" ";
}
cout<<endl;
}
cout<<endl;
}
vector_3d.clear();
return 0;
}
Output:
Output for above program is empty, since we have deleted our 3D vector using
clear()
.