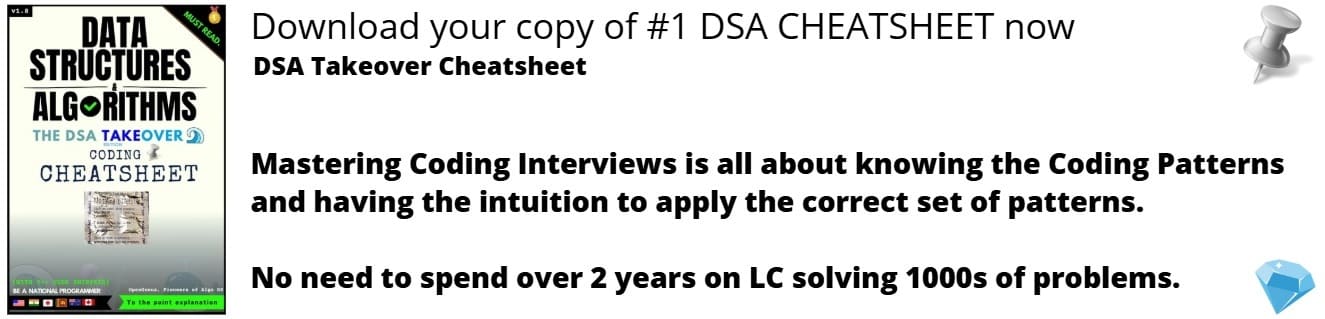
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
A high-level, interpreted programming language that has gained popularity recently is called Python. It is a flexible language that may be used for a range of tasks, including artificial intelligence, data analysis, and web building. Python is a wonderful programming language to start with if you're a beginner. An introduction to Python programming for beginners is provided in this article.
Introduction to Python
The ABC language was replaced by Python, which was developed by Guido van Rossum in the late 1980s. Being open-source, it is possible for anyone to contribute to its development. Python's popularity has increased significantly in recent years, in part due to its ease of use and adaptability.
Installing Python
Python must first be installed on your computer before you can start writing programmes in it. Downloading the Python installer from the official website (https://www.python.org/downloads/) is the simplest way to accomplish this. You can install Python on your computer by following the steps.
Getting started with Python
Python can be installed, and you can begin creating Python code. The simplest method to achieve this is to write your code in a text editor like Notepad or Sublime Text. Your code will be recognised as a Python file if you save it with the.py extension.
Basic Python syntax
Python is straightforward to learn because of its easy-to-read syntax. Here are some fundamental Python syntax guidelines:
- A newline character ends each statement in Python. Statements don't need to be separated by semicolons.
- Coding chunks are identified by indentation. For each level of indentation, four spaces should be used.
- Because of the case-sensitivity of Python, the variables my_var and my_var are distinct.
Data types in Python
Python is capable of working with a wide range of data types, including floats, strings, booleans, and integers. An overview of each data type is provided below:
- Integers: such as 1, 2, 3, etc., are whole numbers.
- Floats: Decimal numbers like 1.5, 2.6, and so forth.
- Text strings, such "hello," "world," etc.
- Boolean values are True or False.
Variables in Python
In Python, data is stored in variables. The = operator can be used to give a value to a variable. For instance:
my_var = 10
my_string = "hello"
my_bool = True
Control flow in Python
If/else, for, and while loops are just a few of the control flow statements that Python offers. By using these statements, you can modify the way your code runs depending on particular circumstances. An if/else statement example is as follows:
x = 10
if x > 5:
print("x is greater than 5")
else:
print("x is less than or equal to 5")
Functions in Python
Code is packaged into reusable units using functions. The def keyword should be used before the function name and any arguments when defining a function. An example of a function that determines the square of an integer is given below:
def square(x):
return x * x
result = square(5)
print(result) # Output: 25
Libraries in Python
There are several libraries in Python that offer extra functionality. The math library, for instance, offers mathematical operations like sin, cos, and tan. The import keyword can be used to import a library. Here is an illustration of how to utilise
the math library in Python:
import math
x = math.sin(1.57)
print(x) # Output: 1.0
Object-oriented programming in Python
Python supports object-oriented programming (OOP) notions like classes and objects because it is an object-oriented language. Objects, which are instances of a class, are defined by classes. Here is an illustration of a Python class definition:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def get_make(self):
return self.make
def get_model(self):
return self.model
def get_year(self):
return self.year
Debugging in Python
Python has a number of tools to assist you in debugging your code, one of which is the print statement, which you can use to display the values of variables at various points throughout your code. Here's an example:
x = 10
y = 5
print("x =", x)
print("y =", y)
if x > y:
print("x is greater than y")
else:
print("x is less than or equal to y")
Resources for learning Python
Python learning materials are widely available, including courses, books, and online tutorials. To help you get started, check out these resources:
- The official documentation for Python: Python's official documentation offers a thorough introduction to the language and all of its available modules and libraries.
- Codecademy: Codecademy provides a free Python course that teaches the fundamentals of Python programming.
- Python classes are available on Udemy at a variety of levels, from introductory to advanced.
- Coursera: The University of Michigan's Introduction to Python course is one of many Python courses available on this platform.
- Al Sweigart's book Automate the Boring Stuff with Python offers a hands-on introduction to Python programming with a focus on automating monotonous operations.
Best practices for programming in Python
It's critical to adhere to recommended practises as you study Python and write code to make sure that it is readable and maintainable. Observe the following best practises:
- Use evocative variable names Instead of using generic names like x and y, give your variables names that accurately describe what they stand for.
- Observe PEP 8: A style manual for Python code is called PEP 8. Your code will be easier to comprehend and more logical if you adhere to its recommendations.
- Comment here: A great method to document your code and explain what it does is to use comments.
- Implement version control: You may collaborate with others and keep track of changes to your code by using a version control system like Git.
- Check the code: Writing tests for your code can help ensure that it works as expected and prevent regressions.
Conclusion
Finally, Python is a robust programming language that is popular in the business and simple to learn. You can begin your path to mastering Python programming by following the advice given in this article at OpenGenus. To continue your learning process, keep practising and look for more materials as necessary.