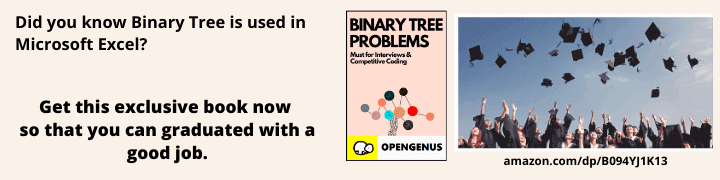
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 15 minutes
In this article, we have explored 7 different methods to convert vector to list in C++ Programming Language.
Table of content
- Introduction to vector and list
- Different methods of converting vector to arrays
- Their implementations
Prerequisite: Vector in C++ STL , List in C++ STL
Vector
Vectors are sequence containers representing C-style dynamic arrays that can change its size dynamically. Sequence containers store elements strictly in linear sequence.
Vector elements are placed in contiguous storage so that they can be accessed and traversed using iterators. It offers fixed time access to elements using at and subscript operator ([]). Vector is part of C++ STL. To use vector in C++, we need to import <vector> library.
List
List in C++ are also sequence container, which allows elements to be stored in non-contiguous memory locations. Also unlike the other standard containers, std::list provides specialized algorithms unique to linked lists, such as splicing, sorting, and in-place reversal.
Traversal up and down the list requires linear time, but inserting and removing elements (or nodes) is done in constant time, regardless of where the change takes place. List are implemented as doubly-linked lists.
Why the need for conversion?:
- Require constant insertion and deletion time
- Traversing list in both directions
You can imagine that you are converting elements of C-style arrays (continuous memory location) to double linked list data structure (stored as non continuous memory location)
Different methods of converting vector to list (7 ways)
- Using a naive solution
- Using range constructor
- Using the std::copy function
- Using the list::insert function
- Using for_each and lambda function
- Using list:: assign function
- Initializing like arrays (=)
1. Using a Naive solution (for loop)
In this naive approach, we are traversing a vector using for loop and one by one inserting elements or objects into the list.
Implementation:
#include <iostream>
#include <vector>
#include <list>
using namespace std;
int main()
{
vector<int> v{1, 2, 3, 4, 5, 7, 8, 9};
list<int> ls;
for (auto i : v)
ls.push_back(i);
for (auto i: ls)
cout << i << " ";
return 0;
}
Output:
1 2 3 4 5 7 8 9
2. Using range constructor
In this, we are passing two input iterators pointing to starting of the vector and the end of the given vector. The range constructor is useful when you want to copy the items of a different type of container, or don't want to copy a full range.
Implementation:
#include <iostream>
#include <vector>
#include <list>
using namespace std;
int main()
{
vector<int> src({1, 2, 3, 4, 5});
list<int> dest(src.begin(), src.end());
for (auto i: dest)
std::cout << i << " ";
}
Output:
1 2 3 4 5
3. Using std::copy function
copy() function exists in C++ STL that allows performing the copy operations in different ways. It is defined in header <algorithm>.
copy(begin_iter1, end_iter1, begin_iter2) : The copy function is used to copy a range of elements from one container to another. It takes 3 arguments:
begin_iter1: The pointer to the begin of the source container, from where elements have to be copied.
end_iter1: The pointer to the end of source container, till where elements have to be copied.
begin_iter2: The pointer to the beginning of destination container, to where elements have to be started copying. In our case it is list.begin().
Implementation
#include <iostream>
#include <vector>
#include <list>
using namespace std;
int main()
{
vector<int> src({1, 2, 3, 4, 5});
list<int> dest;
/* copy(begin_iter1, end_iter1, begin_iter2) :
It is used to copy a range of elements from one container to another.
Iterator types
---------------
back_inserter returns std::back_insert_iterator that uses Container::push_back().
*/
copy(src.begin(), src.end(), back_inserter(dest));
for (auto i: dest)
std::cout << i << " ";
}
Output:
1 2 3 4 5
4. Using list::insert function
list::insert() is used to insert new elements before the element at the specified position. Iterators specify a range of elements. Copies of the elements in the range [first, last)
are inserted at position (in the same order).
Implementation
#include <iostream>
#include <vector>
#include <list>
using namespace std;
int main()
{
vector<int> src({1, 2, 3, 4, 5});
list<int> dest;
// syntax:
// list::insert (iterator position, InputIterator first, InputIterator last);
dest.insert(dest.begin(), src.begin(), src.end());
for (auto i: dest)
std::cout << i << " ";
}
Output:
1 2 3 4 5
5. Using for_each and lambda function
To iterate over all elements of the vector and insert them one by one into the list. For iteration over all elements of the vector, use the for_each() algorithm and pass a lambda function as an argument.
for_each() will apply this lambda function on each of the elements in vector.
Implementation
#include <bits/stdc++.h>
using namespace std;
int main()
{
vector<int> v{1, 2, 3, 4, 5, 7, 8, 9};
list<int> ls;
for_each(v.begin(),
v.end(),
[&](const auto &elem)
{ ls.push_back(elem); });
for (auto i : ls)
cout << i << " ";
return 0;
}
Output:
1 2 3 4 5 7 8 9
6. Using assign function
The list::assign() is a built-in function in C++ STL used to assign new contents to the list, replacing its current elements, and changing its size accordingly.
In the range version, the new contents are elements constructed from each of the elements in the range between first and last, in the same order.
Implementation
#include <iostream>
#include <vector>
#include <list>
using namespace std;
int main()
{
vector<int> src({1, 2, 3, 4, 5});
list<int> dest;
dest.assign(src.begin(), src.end());
for (auto itr = dest.begin(); itr != dest.end(); ++itr)
{
cout << *itr << " ";
}
}
Output:
1 2 3 4 5
7. Initializing like arrays = {..}
In this, we are using the idea of a C-style array initialization method using the = assignment operator.
Implementation
#include <iostream>
#include <vector>
#include <list>
using namespace std;
int main()
{
std::vector<int> src({ 1, 2, 3, 4, 5 });
std::list<int> dest= {src.begin(), src.end()};
//dest.assign(src.begin(), src.end());
for (auto itr = dest.begin(); itr != dest.end(); ++itr)
{
cout << *itr << " ";
}
}
Output:
1 2 3 4 5
Above are the different methods for converting vector to list in C++ STL.