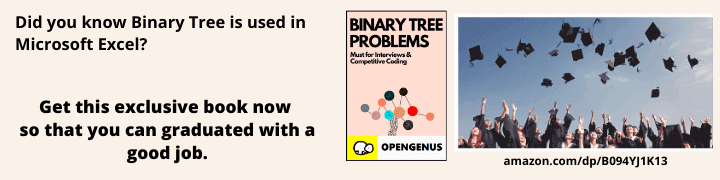
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored the concept of typedef struct in C Programming Language which brings in two core concepts. We have explained the ideas with code examples and differences between typedef struct and struct.
In C language struct is a great way to group several related variables of different data type all at one place. typdef is yet another way used for declaring the type of structure in C language.
More so the fact that, typedef gives freedom to the users by allowing them to create their own data types.
Table of Contents :
- Introduction to typedef
- Typedef struct (concept and implemented code)
- typedef and its uses
- Differences between "typedef struct" and "struct"
- Advantages of Typedef
- Conclusion
Point | typedef struct in C |
---|---|
What is it? | An implementation approach for defining structure (struct) |
Why it is used? | Coding convention and style |
Concepts involved | typedef, structure |
Programming Languages | C, C++ |
Code Snippet |
|
Introduction to typedef
typedef is a keyword that gives a new name to an existing data type.
For example, you can give a new name to an existing data type unsigned int type and program using uint in its place. At this time, uint will also be treated exactly as unsigned int.
The point is with typedef you can create a new name, but you don't create a new type itself.
typedef is for using the original data type with a different name, and the original type always exists.
Basic Syntax :
typedef <existing_data_type> <new_data_type>
Typedef struct
Concept:
When writing a code we certainly at most times have felt the need to define our own data type as per the requirement of the problem. In C language this can be achieved using two keywords: struct and typedef. These keywords help us to group non-homogeneous data types into a single group.
The question remains why we need typedef if we still have struct?
Putting it simply, typedef means we no longer have to write struct every time in our code. Hence it makes our code look clean and readable. It also makes the code portable and easily manageable.
This can be shown by the following implemented code:
Implementation of code
#include <stdio.h>
typedef struct {
char name[20];
char sex;
int age;
} person_t;
main()
{
person_t p = {"Tom", 'M', 19};
printf("%s %c %d\n", p.name, p.sex, p.age);
return 0;
}
}
Output :
Tom M 19
Code Explanation :
- First we have to use the keyword typedef before struct.
- Then we can mention variables of different data types to be grouped under that struct.
- Then we have written "person_t" as the name of this group.
- Here, comes the real roleplay of typedef where we do not have to mention "struct" again in our program and simply write person_t.
- At the same time that you declare a variable of structure type, you can set the initial value of each of its members. The initial value of each member is enclosed in { } and becomes the value of each member in the order in which the structure is defined.
typedef and its uses
Hence, the typedef keyword helps user to provide meaningful names to the already existing data types in the C language and make it more understandable for others as well.
For example , if you want to name int newly as MyInt , write as follows.
typedef int MyInt;
So, now instead of using int we can also use MyInt as a substitute name for this data type. The same can be shown in the below implemented code.
Example:
#include <stdio.h>
typedef unsigned int MyInt;
MyInt add(MyInt, MyInt);
MyInt add(MyInt a, MyInt b) {
return a + b;
}
int main(void) {
MyInt a, b, c; //Variable declaration
char d = 72;
a = (MyInt)d; //typecast
b = 245;
c = add(a, b);
printf("%u\n", c);
printf("%u\n", sizeof(MyInt)); //sizeof get size by
return 0;
}
Output
317
4
Code Explanation :
- First we have to specify this keyword typedef before struct.
- Then the existing data type and then the new data type.
- Finally this existing data type will get replaced by this new data type.
- In the above code we have substituted int data type by giving it another name MyInt and performed basic operations like add, typecasting, variable declaration using it. This shows that aliases made with typedef execute the same behavior as their original data type.
From the above examples we understand that we can accomplish the following by using typedef:
- Variable declaration
- typecast
- function arguments
- function return value
- sizeof get size by
A compilation error may arise in the following cases :
- ; missing at the end
- 1st and 2nd designations are reversed
- first type name does not exist
- The second type name specifies a type name that already exists
- Invalid characters are used in the second type name
- You can't use characters that probably aren't allowed in variable names
- no space
Differences between "typedef struct" and "struct"
When defining structs, it is more convenient to use typedefs.
When declaring a struct variable, you must prefix the struct name with the keyword "struct".
Writing a struct every time you use a struct is a bit of a hassle.
Example of struct without using typedef
#include <stdio.h>
struct Person
{
char name[50];
int age;
char gender;
};
int main()
{
struct Person person = { "John", 22, 0 }; //we will have to write struct Person every time
printf("name: %s\n"
"age: %d\n"
"gender: %d\n",
person.name, person.age, person.gender);
getchar();
}
Example of struct using typedef:
#include <stdio.h>
typedef struct Person
{
char name[50];
int age;
char gender;
} Person;
int main()
{
Person person = { "John", 22, 0 };///we will now not have to write struct Person every time
printf("name: %s\n"
"age: %d\n"
"gender: %d\n",
person.name, person.age, person.gender);
getchar();
}
The output we will get the same in both the above programs:
name: John
age: 22
gender: 0
The following points of difference have also been discussed in the following table :
struct without using typedef | struct using typedef | |
---|---|---|
We are calling struct Person every time and it is defined in our main function. | Now, struct Person is the old data type and Person becomes the new data type. | |
struct is used to define a structure. | typedef is used to give an alias name to a data type and the data type can be a predefined data type (like int,float, char) or a user defined data type. | |
After the second declaration we will have to refer to that structure as "struct StructName" throughout the program. | typedef helps us to avoid the use of word 'struct' all over the program. | |
We cannot do forward declaration here. | The concept of forward declaration comes in because the name can be referenced before it is declared. | |
The compiler doesn't consider the structure defined until it gets to the semicolon at the very end, so it's just gets declared before that point. | Because we have given the alias name just after typedef struct alias name, so now it can be still be referenced in start. |
Note : In C++ programming language, there is no difference between 'typedef struct' and 'struct'.
Advantages of Typedef
- Easy to read source code - With typedef You can make the source code easier to read by creating a type that is easy to understand.
- Easier to write types - typedef by shortening the new type name, it becomes easier to write that type.
Conclusion
In this article at OpenGenus, we learned about typedef and how we can use it to create an alias of an existing data type. Also we learned how to use typedef in struct in C programming language and how it works to make our code look cleaner and more readable.