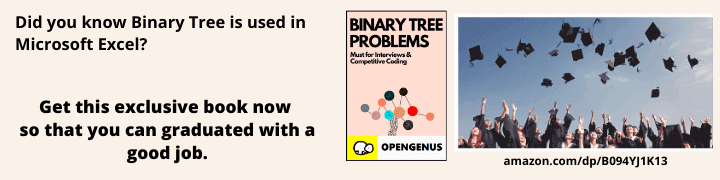
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Introduction
In this article, I shall be explaining negative indexes in Python. But first, we have to know these prerequisites: iterables and indexing. Having a fair knowledge on these two are paramount to understanding how negative indexes works.
So we shall be looking at the following:
- Iterable
- Indexing
- Negative indexes
- Pictorial illustration of negative indexes
- Code examples of the use of neagtive index in a
list
iterable - Benefits of negative indexes
Iterable
An iterable is an object that can be traversed over. Meaning you can go through all the elements contained in the object. Iterables are also capable of returning each element they contain one-by-one and the amount of elements they can contain is only limited by the memory size of the computer. Examples of iterables are:
- Lists
- Sets
- Tuples
- Dicts (Dictionaries)
- Strings
Although Sets and Dicts are iterables, they do not have indices. Meaning that thier elements cannot be indexed unlike Lists, Tuples and Strings
Indexing
Indexing in Python is performed on iterables. It is a means of getting individual elements within an iterable by the element's position. This enables you to get or remove a specific element within a selected iterable if that elements postion is known.
Elements in an iterable are normally positively indexed in Python, starting from zero (0) and going up to the tail or last element of the iterable.
Negative Index
Earlier, we mentioned that elements in a Python iterable are positively indexed by default. The reverse is also possible here as they can be negatively indexed as well, starting from -1.
Negative indexes in Python works completely differently from positive (or zero-based) indexes. With negative indexes, elements are returned from the end of the iterable, with the last element having a value of -1. As negative indexes are used to get or remove an element starting from the back of the iterable, they are very useful in slicing.
Slicing in Python is the means of accessing parts of an iterable like strings, lists and tuples.
Illustration
Code Examples
In the code examples, we shall be using the list
iterable as a preferred choice. The same example also works for tuple
and str
(string) iterables.
1. Returning elements in a list
iterable with negative index
#list definition
test_list = [0, 1, 2, 3, 4, 5, 6]
#returns the 'last' element in the list
print(test_list[-1])
#returns the 'second to last' element in the list
print(test_list[-2])
#returns the 'third to last' element in the list
print(test_list[-3])
Output
6
5
4
In this example, we define and initialize test_list
and using the negative index to get the last 3 values, 6, 5, 4
was printed vertically on the screen.
2.Removing elements in a list
iterable with negative index
Using the builtin function pop()
, we can remove any element from the list to get a new list.
This will not work for the
tuple
iterable since they are immutable. meaning that thier values cannot be changed or removed.
#list definition
test_list = [0, 1, 2, 3, 4, 5, 6]
#removes the 'last' element in the list
test_list.pop(-1)
#removes the 'second to last' element in the list
test_list.pop(-2)
#removes the 'third to last' element in the list
test_list.pop(-3)
print(test_list)
Output
[0, 1, 2, 3]
In this example, we used pop()
to access and remove the last 3 elements in the list which resulted in a new list.
3. Slicing a list
iterable with negative index
#list definition
test_list = [0, 1, 2, 3, 4, 5, 6]
#Slicing the list with negative index
#Assigning the new list to a variable
sliced_list = test_list [-5:-1]
#Returns the new list
print(sliced_list)
Output
[2, 3, 4, 5]
In this example, we defined a list and sliced it to create a new list. The slicing was done from the last element (exclsuive) to the fifth to last element (inclusive).
The syntax for slicing in Python is
item[start:stop:step]
, with all of them being optional values if you just want the original list back after slicing (which is redundant and probably best to avoid).
4. Reversing the order of a list
iterable with negative index
#list definition
test_list = [0, 1, 2, 3, 4, 5, 6]
#reversing the list
#assigning the reversed list to a variable
reversed_list = test_list[::-1]
#returns the reversed list
print(reversed_list)
Output
[6, 5, 4, 3, 2, 1, 0]
In this example, we reversed the list to display the last element first and the first element last using a negative index step of -1. This is required in order to traverse through the list from the back in steps of 1.
Benefits of Negative index
Negative indexing has a lot of advantages in programming. Some of these benefits include:
- Reverses an iterable in just a single line of code which enhances productivity.
- Enables access to the last element of an iterable in case the size is not known.
- Helps in negative slicing.
- Reduces the overall complexity of performed operations on an iterable.