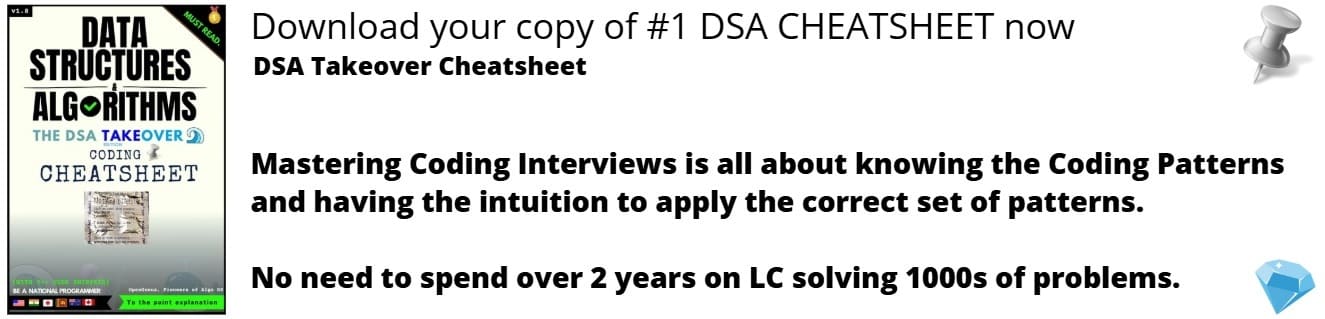
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 25 minutes | Coding time: 10 minutes
We have been handling exceptions that causes compile time errors and run time errors. At times, we may also require to throw our own exceptions. Such exceptions are known as User-defined exceptions or Custom exceptions.
Exceptions are any contradiction or unexpected situation that causes run time error in the program or disrupts the normal flow of the program.
Custom Exceptions: These exceptions are not defined in Java librairies, but can be defined by us to handle customized error situations. Example: Suppose, our banking transaction program should raise exception for debit from an account with zero balance
Types of Exceptions in Java
Exceptions in Java can be broadly classified under two categories:
- Built-in Exceptions: These exceptions are already defined in Java libraries and are helpful for some error situations.
- User-defined Exceptions (Custom Exceptions): These exceptions are not defined in Java librairies, but can be defined by us to handle cutomized error situations. Example: Suppose, our banking transaction program should raise exception for debit from an account with zero balance but Java does not have any built-in exceptions class to do that.
Note: Exception is a subclass of Throwable and thus all custom exceptions are also subclass of Throwable class.
We can throw our own exceptions using the keyword throw as :
throw new Throwable_subclass;
Examples:
throw new ArithmeticException();
throw new ArrayIndexOutOfBoundsException();
The examples shown above throws exceptions already defined in java library. We will be soon looking into throwing user-defined exceptions.
Steps to create user-defined exception:
- Create a subclass of the Exception class. All exceptions are subclasses of Exception class.
class MyException extends Exception
- Inside MyException class, let's define our own default constructor as:
MyException(){ }
- Define a parameterized constructor with string as parameter if you want to store exception details. Now we need to send this string to the superclass Exception. So, let's call it inside our constructor using the super keyword, just like this:
MyException(String str) {
super(str);
}
- Now, we are ready to call our user-defined exception MyException in our program. We do it as:
MyException ex = new MyException();
throw ex;
- Now from the knowledge about exceptions, we know that we need a try-catch block to handle exceptions. So, we do it as:
try {
MyException ex = new MyException();
throw ex;
}
catch(MyException e) {
System.out.println("Caught the user-defined exception");
}
Let us now look at the custom exception implementation in Java:
import java.lang.Exception;
// Custom exception class definition...
class MyException extends Exception {
MyException(String message) {
super(message);
}
}
// Main class that will use the custom exception...
class TestMyException {
public static void main(String args[]) {
int x = 5, y = 1000;
// try-catch-finally block to handle exceptions...
try {
float z = (float) x / (float) y;
if(z < 0.01) {
throw new MyException("Number is too small"); // Exception is hit and thrown
}
}
catch(MyException e) { // Exception matches the catch block and is caught
System.out.println("Caught my Exception");
System.out.println(e.getMessage());
}
// Code fragment inside finally block runs irrespective of exception occurence
finally {
System.out.println("Execute me always");
}
}
}
Explanation:
First line indicates, importing necessary libaries. By default, java.lang
package is imported. The line is optional and may be used for demonstration.
import java.lang.Exception;
Next, we define our custom exception, MyException class as:
class MyException extends Exception {
MyException(String message) {
super(message);
}
}
The lines following are definition of class TestMyException
which will use our custom exception class MyException to raise exceptions.