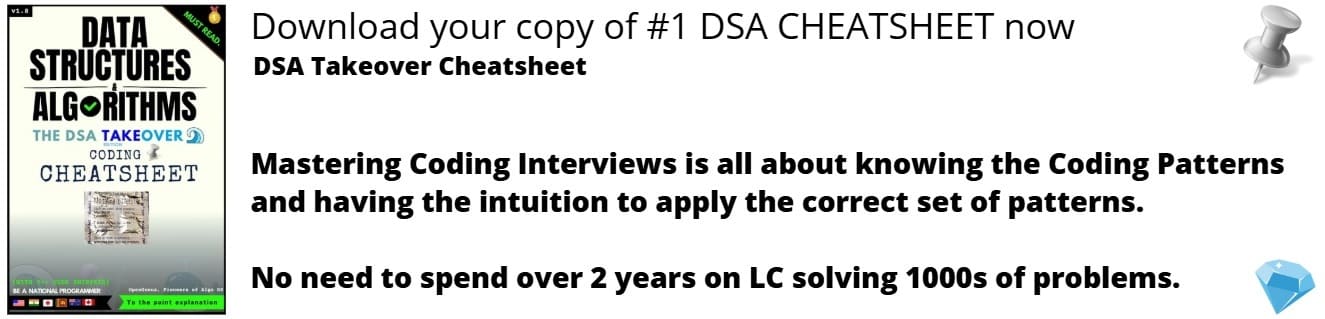
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will explore the concept of Data Sampling and Data Splitting in Machine Learning.
Table of contents:
- Introduction
- Data Sampling
- Program for performing Data Sampling in ML
- Advantages and Disadvantages of Data Sampling in ML
- Applications of Data Sampling in ML
- Data Splitting
- Program for performing Data Splitting in ML
- Advantages and Disadvantages of Data Splitting in ML
- Applications of Data Splitting in ML
- Conclusion
Introduction
Machine learning (ML) is an approach to artificial intelligence (AI) that involves training algorithms to learn patterns in data. One of the most important steps in building an ML model is preparing and splitting the data into training and testing sets. This process is known as data sampling and splitting. In this article, we will discuss data sampling and splitting in ML and the different techniques used for it.
Data Sampling
Data sampling is the process of selecting a subset of data from a larger dataset to use in a machine learning model. The sampling technique used depends on the type of data and the problem at hand. There are two main types of sampling techniques:
-
Random Sampling: In random sampling, each data point in the dataset has an equal chance of being selected. This technique is used when the data is uniformly distributed and representative of the entire population.
-
Stratified Sampling: Stratified sampling is used when the dataset has an uneven distribution of data points across different categories or classes. In stratified sampling, the dataset is divided into subgroups or strata based on these categories, and samples are taken from each stratum.
Code for performing Data Sampling in python:
import pandas as pd
from sklearn.model_selection import train_test_split
#Load the dataset
data = pd.read_csv('data.csv')
# Random sampling
sampled_data = data.sample(n=100, replace=False)
# Stratified sampling
stratified_sampled_data = data.groupby('class').apply(lambda x: x.sample(n=50))
# Split the data into training and testing sets
train_data, test_data = train_test_split(data, test_size=0.2, random_state=42)
In this code, we first load the dataset using the pandas library. We then perform random sampling using the sample() method, where we randomly select 100 data points from the dataset without replacement. We also perform stratified sampling using the groupby() method, where we group the data by the class column and select 50 data points from each group.
Finally, we split the original dataset into training and testing sets using the train_test_split() method from the sklearn library. We specify a test size of 0.2, which means that 20% of the data will be used for testing, and the rest will be used for training. We also set a random state of 42 to ensure that the splitting is reproducible.
Note that the sampling and splitting techniques used in this code are just examples, and different techniques may be more appropriate depending on the dataset and the problem at hand.
Advantages and Disadvantages of Data Sampling
Here are some advantages and disadvantages of data sampling in machine learning:
- Advantages:
-
Reduces training time and computation: Data sampling reduces the size of the training dataset, which can reduce the time and computational resources required for training a model.
-
Helps to balance class distribution: Data sampling can be used to address class imbalance, where one class is much more prevalent than another in the dataset. By oversampling the minority class or undersampling the majority class, data sampling can help to balance the class distribution, which can improve model performance.
-
Can improve model performance: Data sampling can help to reduce overfitting, which occurs when a model learns the training data too well and performs poorly on new data. By reducing the size of the training dataset or balancing the class distribution, data sampling can improve model performance and reduce overfitting.
- Disadvantages:
-
Can introduce bias: Data sampling can introduce bias if the selected subset is not representative of the underlying population. For example, if a biased sample is selected, the resulting model may not generalize well to new data.
-
May discard useful information: Data sampling can result in the loss of useful information, particularly if the sample is too small or not representative of the underlying population. This can lead to an underfit model that performs poorly on new data.
-
Can be sensitive to the sampling method: The effectiveness of data sampling can depend on the sampling method used, and different methods may be more or less effective depending on the specific dataset and problem. Choosing an appropriate sampling method requires careful consideration and experimentation.
Applications of Data Sampling in ML
Data sampling is a commonly used technique in machine learning with various applications, some of which include:
-
Class imbalance: In many real-world datasets, one class may be much more prevalent than another, which can lead to biased model performance. Data sampling can be used to address class imbalance by oversampling the minority class or undersampling the majority class, which can improve model performance.
-
Large datasets: When working with large datasets, training a model on the entire dataset can be computationally expensive and time-consuming. Data sampling can be used to select a smaller subset of the data for training, which can reduce the time and computational resources required for training a model.
-
Anomaly detection: In datasets where anomalies are rare, data sampling can be used to increase the prevalence of anomalies in the training dataset, which can help the model to detect and classify anomalies more effectively.
-
Quality control: In manufacturing and industrial applications, data sampling can be used to select a representative subset of data for quality control analysis. This can help to identify defects or anomalies in the production process and improve product quality.
-
Fraud detection: In financial applications, data sampling can be used to identify fraudulent transactions by oversampling the minority class (fraudulent transactions) and undersampling the majority class (legitimate transactions).
Data Splitting
Data splitting is the process of dividing the dataset into two or more sets for training and testing the ML model. The most common splitting technique is the 80-20 rule, where 80% of the data is used for training the model, and the remaining 20% is used for testing the model's accuracy. Other techniques include:
- K-Fold Cross-Validation: In K-fold cross-validation, the dataset is divided into K subsets or folds. The model is trained on K-1 folds and validated on the remaining fold. This process is repeated K times, with each fold serving as the validation set once.
2.Leave-One-Out Cross-Validation: In leave-one-out cross-validation, the model is trained on all data points except one and validated on the single omitted data point. This process is repeated for all data points, and the model's performance is evaluated by averaging the results.
3.Time-Based Splitting: Time-based splitting is used when the dataset has a time-series structure, and the order of the data points is essential. In this technique, the data is split into training and testing sets based on a specific time point. The model is trained on the data before the time point and tested on the data after the time point.
Code for performing data splitting in Python
# Stratified splitting based on a specific column
train_data, test_data = train_test_split(data, test_size=0.2, stratify=data['class'], random_state=42)
# Splitting for time-series data
train_data = data.loc[data['timestamp'] < '2022-01-01']
test_data = data.loc[data['timestamp'] >= '2022-01-01']
In the first example, we perform stratified splitting based on the class column by setting the stratify parameter to data['class']. This ensures that the proportion of each class is the same in both the training and testing sets.
In the second example, we perform splitting for time-series data by selecting all data points before a specific timestamp for the training set and all data points after or on that timestamp for the testing set. This ensures that the model is trained on past data and tested on future data.
Advantages and Disadvantages of Data Splitting
Here are some advantages and disadvantages of data splitting in machine learning:
- Advantages:
-
Helps to estimate model performance: By using a separate testing dataset, data splitting allows us to evaluate the performance of our model on new and unseen data. This helps to estimate how well the model is likely to perform on real-world data.
-
Reduces overfitting: By testing our model on a separate dataset, we can avoid overfitting, which occurs when a model is too complex and learns the training data too well, resulting in poor performance on new data.
-
Enables hyperparameter tuning: By splitting the data into training and validation subsets, we can use the validation set to tune hyperparameters such as the learning rate, regularization strength, and other model parameters, which can improve the performance of the model.
- Disadvantages:
-
Can introduce bias: Data splitting can introduce bias if the training and testing subsets are not representative of the underlying population. For example, if a random sample of the data is used for training and testing, it may not be representative of the population, which can lead to inaccurate estimates of model performance.
-
Can lead to unstable models: The performance of a model can vary depending on the specific samples used for training and testing. If the samples are too small or not representative, the resulting model may be unstable and unreliable.
-
May not reflect real-world data: The testing data used in data splitting may not accurately reflect the real-world data that the model will encounter in production. This can lead to over-optimistic estimates of model performance and poor generalization to new data.
Applications of Data Splitting in ML
Here are some applications of data splitting in machine learning:
-
Model selection: Data splitting is commonly used to select the best model among different algorithms or hyperparameters. The training dataset is used to train and fit the model, the validation dataset is used to evaluate and compare the performance of different models, and the testing dataset is used to evaluate the performance of the final model.
-
Avoiding overfitting: Overfitting occurs when a model performs well on the training data but poorly on new data. Data splitting can be used to avoid overfitting by evaluating the model's performance on a separate testing dataset that the model has not seen during training.
-
Tuning hyperparameters: In machine learning, hyperparameters are the configuration settings that are set before training a model. Data splitting can be used to tune hyperparameters by using the validation dataset to evaluate the performance of different hyperparameter settings.
-
Early stopping: Early stopping is a technique used to stop the training process when the model's performance on the validation dataset starts to degrade. Data splitting is used to monitor the model's performance on the validation dataset and stop the training process when the performance starts to degrade.
-
Ensemble models: Ensemble models are a combination of multiple models to improve performance. Data splitting is used to train different models on different subsets of the training dataset, and then the models are combined to create an ensemble model.
Conclusion
With this article at OpenGenus, you must have the complete idea of Data Sampling and Data Splitting in ML.
Data sampling and splitting are crucial steps in building an ML model. Proper sampling and splitting techniques can help avoid overfitting, where the model performs well on the training data but poorly on new data, and underfitting, where the model is too simple to capture the underlying patterns in the data. Random and stratified sampling, along with techniques like K-fold cross-validation, leave-one-out cross-validation, and time-based splitting, can help build accurate and robust ML models.