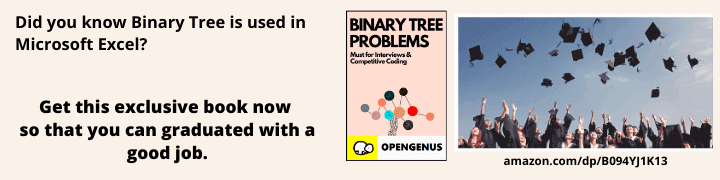
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Data in layman's terms is the information that is stored in a computer. Data Type is the type of data that can be stored in a particular variable.
Data Types define the operations that can be performed on a variable and the amount of memory allocated for a variable.
Dart Programming Language is a type inferred language which means the compiler automatically gets to know the data type based on the values we assign.
Here are the Data Types which are built-in Dart
- Numbers (Integer and Double)
- Boolean
- String
- Maps
- Lists
- Symbols
Now we will discuss in detail about each Data Type present in Dart
1. Numbers
Number Data type can hold the numeric values. Dart supports both Integer and Double numeric values.
Integer Data Type
Integer Data Type is used when whole numbers need to be stored in a variable. It can store either unsigned value(only positive numbers) or signed(both positive and negative numbers).
The value range that Integer data type can hold is from -2^63 to 2^63
Integers in Dart can be declared using the int keyword.
Example
int age = 18 ;
Here ,
int is the data type.
age is the variable name used to store the integer value.
18 is the integer value that is stored in the variable age.
Double Data Type
Double is used to store double precision(numbers with large decimal points) also called as floating point numbers.
The keyword used to declare double is double
Example
double weight = 72.56 ;
Here , double is the data type .
weight is the variable name used to store the value.
72.56 is the value of the double stored in variable weight.
2. Boolean
Boolean Data Type is used to store or represnt boolean values(0 and 1). 1 indicates True and 0 indicates False. Boolean Data type is widely used in decision making statements.
The keyword used to declare Boolean values is bool
Example
bool value = false ;
Here , bool is the data type.
value is the variable name.
false is the boolean value(0)
3. Strings
A String can be defined as sequence of characters,numbers,special characters. It can be represented by using either single quotes or double quotes in Dart.
String data type can hold sequence of characters, special characters and numbers.
String data type can be declared in Dart using the string keyword.
Example
string name = "OpenGenus Foundation";
Here , string is the data type.
name is the variable name.
OpenGenus Foundation is the string name.
4. Maps
Map is used to hold a set of values as key or value pairs which can be of any type. But each key can occur only once but the value of that key can be used multiple times.
Maps can be declared using {} and the values can be accessed using []
Example:
void main()
{
var record = {'Name':'Sughosh' , 'Age':'18'} ;
record['number'] = 's002' ;
print(record) ;
}
The Code will output the following
{Name : Sughosh , Age : 18 , number: s002}
5. Lists
Dart represents arrays in form of list objects. A List is a data type used to store ordered collection of objects.
A list can be declared by using [] brackets in which values must be seperated by commas , .
Example
var numbers = [2,4,6,8];
Here , numbers is the list name.
2,4,6,8 are list objects.
6. Symbols
Symbols are used to refer to an identifier declared in a Dart program. Symbols are commonly used in API's that refer to the identifers by name.
Symbol can be declared by using # and the identifer name.
Example
#name
here name is the identifer
Variables in DART
Variables are identifiers that are used to refer to the computer memory that holds the variable value.
The value of the variable is not constant. They can be changed during the execution.
There are some rules which have to be followed before we name a variable in Dart.
- Special characters are not allowed(@,&,#). There is an exception for dollar sign($) and underscore (_)
- A keyword cannot be used as a variable name for example int , double cannot be used as a variable name.
- Variables are case sensitive
- First character of a variable should not be a digit and must always be an alphabet.
- Blank spaces cannot be used
- A combination of numbers and alphabet can be used to name a variable for example , name123 can be used as a variable name.
Declaring a variable
Variables must be declared before they are assigned a value or used. Variables can be declared using the keyword var
Syntax
var variable_name ;
Example
var age ;
Multiple Variable Declaration
Multiple variables can be declared in a single statement. But they should be seperated by commas.
Syntax
var variable1, variable2, variable3 ;
Example
var name,age,date_of_birth ;
Variable initialization
In Dart, a variable can be decalred and a value can be assigned to the variable.
Syntax
var variablename = value ;
Example
var age = 18;
Variable Assignment Operation
Values can be assigned to variables using the assignment operator (=).
Syntax
var variable name ;
variable name = value ;
Example
var name ;
name = Sughosh ;
Here , name is the variable name and Sughosh is the value assigned to the variable name using the assignment operator (=)