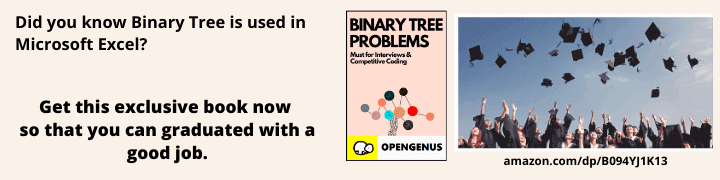
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Authentication is a common process in the world of web and mobile app development. In a global system where different users can have access to a particular software, it is important to identify (authenticate) all users and grant privileges (authorize) to each one.
The different types of User Authentication Techniques are:
- Session-based Authentication
- Cookie-based Authentication
- Token-based Authentication
- Claims-based Authentication
- HTTP Authentication
- Bearer authentication
- Digest authentication
- Form-based authentication
Simply defined, Authentication is a means of verifying who a user is, while Authorization is a means of determining what a user can see and have access to when logged on. Authentication answers the question of "who you are?" to an application, while Authorization answers the question of "what you have access to as a user?"
There are various ways a user can be authenticated - some of which include, Session-based authentication, Cookie-based authentication, Token-based authentication, Claims-based authentication and HyperText Transfer Protocol (HTTP) authentication mechanisms. In this article, we'd be taking a dive into each methods listed above, and understand the roles they play in protecting a software application.
Session-based Authentication
In a session-based authentication, when a user logs in, the server stores the session information (client state) in the server memory and a session id is sent to the client browser - which is then stored using (most likely) cookies on the browser. On subsequent requests to server, the server compares the session id with the session information stored in its memory, and sends the corresponding response. Once a user logs out, the session is destroyed from both client and server side. Sessions are typically stateful on the server side (i.e. they are stored on the server).
Cookie-based Authentication
Cookie-based authentication is basically a type of session-based authentication, in which session data are stored using cookies. Considering HTTP is a stateless protocol, cookies are used to store information concerning the user on the browser - incase of subsequent requests to server. Adapting a cookie-based authentication in web applications can prevent your site against XSS (Cross Site Scripting) attacks, as there some security flags available using cookies to protect user data. Additional flags can also be used to prevent a site against CSRF (Cross Site Request Forgery) attacks.
Token-based Authentication
There's much preference for token based authentication in web applications, due to its scalability and compatibility with mobile applications. In a token based authentication, the client data is encrypted in a JSON Web Token (JWT) by the server, and sent back to the client. The JWT is usually stored in local storage, but can also be stored in a cookie. On subsequent requests, the web token is first verified by the server, followed by a corresponding response from the server. One interesting thing about token based authentication is its statelessness (client state NOT stored on server), compared to a stateful (client state stored on server) approach - like in session based authentication. Once the user logs out, token is destroyed from both client and server side.
Claims-based Authentication
This type of authentication is similar to a token-based authentication. Here users are authenticated on external systems, called identity providers. These identity providers then issue a security token - which contains information about authenticated user. These informations are referred to as claims. Claim is a piece of information that describes a given identity (user) in regards to authorization. When a user requests access to an application, the application redirects him/her to the authentication page of chosen identity provider. After successful authentication, the user is redirected back with some information. The application then requests the user to be validated by external system, and upon successful validation, the user is granted access to the application.
HTTP Authentication
This is one of the simplest ways to identify users logged into a system. Common types of HTTP authentication include: Basic, Bearer, Digest and Form Based.
In Basic access authentication, the client provides a username and password when making a request, which is sent in an Authorization header. The username and password are concatenated into a single string username:password which is encoded in Base64, and written with the Basic keyword before the encoded string "Authorization: Basic am9objpzZWNyZXQ=" example.com
.
Although, this method doesn't require any saved sessions or tokens, it is not as secure and can be prone to attacks. In Python, this request can be made using the requests library as shown below.
# Open OpenGenus discourse to test authentication
from requests.auth import HTTPBasicAuth
requests.get('https://discourse.opengenus.org/u/user/', auth=HTTPBasicAuth('userName', 'password'))
# Alternative method
requests.get('https://discourse.opengenus.org/u/user/', auth=('user', 'pass'))
Bearer authentication
Bearer authentication, also called token authentication is a HTTP authentication mechanism that makes use of cryptic strings (called Bearer Tokens). Bearer Tokens are generated by the server during a login request, and similarly to basic authentication, the token is sent in an Authorization header - with the Bearer keyword. The bearer authentication mechanism was initially created to access OAuth 2.0-protected resources, but is sometimes used on its own "Authorization: Bearer <token>"
.
Digest authentication
In Digest authentication, all the user credentials (usernames and passwords), HTTP methods and requested URIs are encrypted using MD5 (message-digest algorithm) hashing before being sent to the server. However, the digest access authentication mechanism is still vunerable to man-in-the-middle attacks.
from requests.auth import HTTPDigestAuth
response = reqs.get('https://postman-echo.com/digest-auth', auth=HTTPDigestAuth('postman',
'password'))
Form-based authentication
In Form-based authentication, the user credentials (username and password) are sent in a plain text (like Basic authentication) using forms, which can lead to exposure of usernames and passwords except connecting via HTTPS/SSL. One benefit is that, the developer is allowed to customize the authentication and error pages sent by the browser.
Key Differences
- Token-based authentication: stateless mechanism with tokens (usually JWT) used for authentication.
- Session-based authentication: stateful mechanism. sessions can be managed using cookies, JSON web tokens etc.
- Claims-based authentication: users are authenticated on external systems (called identity providers), and claims are sent back to target application for validation. Claim is a piece of information that describes a given identity.
- Cookie-based authentication: cookies are used for client/server requests.
In conclusion, there are other user authentication methods beyond the scope of this article, but these are the most commonly used types of authentication. When deciding on which method is best for a web application, you should always consider the use case. For instance, a session-based authentication is useful in the development of web platforms - however, if the web platform would also require a mobile platform, it is recommended to use a token-based authentication as this would be dealing with APIs.