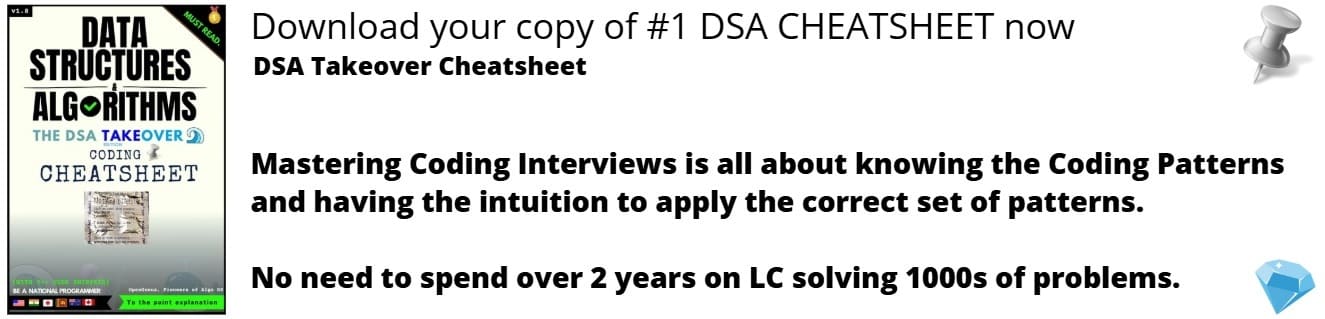
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Tables are a fundamental part of HTML and CSS is used to format tables nicely. Bootstrap being a CSS framework provides several classes to design HTML tables easily.
The different types of Bootstrap tables are:
- Default Table
- Bordered Table
- Striped Table
- Contextual Classes Table
- Dark Table
- Borderless Table
Before going into Bootstrap tables, we will go through the basics of bootstrap and get to know how to use it.
What is Boostrap?
Bootstrap is a free and open-source CSS framework directed at responsive, mobile-first front-end web development.
Where to Get Bootstrap?
There are two ways to start using Bootstrap on your own web site.
-
Download Bootstrap from https://getbootstrap.com
If you want to download and host Bootstrap yourself, go to getbootstrap.com, and follow the instructions there. -
Include Bootstrap from a CDN
MaxCDN provides CDN support for Bootstrap's CSS and JavaScript. You can include these links in your header section of the code and get started with Bootstrap. You need to include jQuery too.
Latest compiled and minified CSS
<link rel="stylesheet"
href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
jQuery library
<script
src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js">
</script>
Latest compiled JavaScript
<script
src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js">
</script>
It will look like-
<!DOCTYPE html>
<html lang="en">
<head>
<title>Bootstrap Tables</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
</head>
What is Bootstrap table?
Bootstrap contains CSS and JavaScript-based design templates for different UI components like typography, forms, buttons, navigation etc. Among these components, table is one of the most used components.
In this post, we will learn to create different tables using Bootrap. It provides a clean layout for building tables. Bootstrap has a number of default classes using which we can easily and quicky build various kind of tables with different style and functionality. It also makes the tables responsive and mobile-friendly. Utilizing default Bootstrap classes can style our table within a wide variety of formats. Let's see few examples of these tables.
Different Bootstrap tables
1. Default Table:
The .table class adds basic styling to the table with no padding and horizontal dividers. This builds a very basic table layout.
<body>
<div class="container">
<h2>Default Table</h2>
<table class="table">
<thead>
<tr>
<th>First name</th>
<th>Last name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
<tr>
<td>Jon</td>
<td>Doe</td>
<td>21</td>
</tr>
<tr>
<td>Jon</td>
<td>Smith</td>
<td>22</td>
</tr>
</tbody>
</table>
</div>
</body>
Output:
2. Bordered Table:
The .table-bordered class adds borders on all four sides of the table and around each cell.
<body>
<div class="container">
<h2>Bordered Table</h2>
<table class="table-bordered" style="width:100%">
<thead>
<tr>
<th>First name</th>
<th>Last name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
<tr>
<td>Jon</td>
<td>Doe</td>
<td>21</td>
</tr>
<tr>
<td>Jon</td>
<td>Smith</td>
<td>22</td>
</tr>
</tbody>
</table>
</div>
</body>
Output:
3. Striped Table:
The .table-striped class adds stripes to the rows of table.
<body>
<div class="container">
<h2>Bordered Table</h2>
<table class="table-striped" style="width:100%">
<thead>
<tr>
<th>First name</th>
<th>Last name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
<tr>
<td>Jon</td>
<td>Doe</td>
<td>21</td>
</tr>
<tr>
<td>Jon</td>
<td>Smith</td>
<td>22</td>
</tr>
</tbody>
</table>
</div>
</body>
Output:
4. Contextual Classes Table:
Contextual classes can be used to color table rows and cells.
The contextual classes that can be used are:
.active - Applies the hover color to the table row or cell.
.success - Indicates a successful or positive action with color green.
.info - Indicates a neutral informative action with color blue.
.warning - Indicates a warning action with color yellow.
.danger - Indicates a negative action with color red.
<body>
<div class="container">
<h2>Contextual Classes</h2>
<table class="table">
<thead>
<tr>
<th>Indication</th>
<th>Name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
<tr>
<td>Default</td>
<td>Jon Doe</td>
<td>22</td>
</tr>
<tr class="success">
<td>Success</td>
<td>Jon Doe</td>
<td>24</td>
</tr>
<tr class="danger">
<td>Danger</td>
<td>Jon Smith</td>
<td>20</td>
</tr>
<tr class="info">
<td>Info</td>
<td>Jon Edwards</td>
<td>29</td>
</tr>
<tr class="warning">
<td>Warning</td>
<td>Jon Doe</td>
<td>25</td>
</tr>
<tr class="active">
<td>Active</td>
<td>Jon Doe</td>
<td>27</td>
</tr>
</tbody>
</table>
</div>
</body>
Output:
5. Dark Table:
We can use the modifier classes like .thead-light or .thead-dark to make table heads appear light or dark gray.
<table class="table">
<thead class="thead-dark">
<tr>
<th scope="col">Firstname</th>
<th scope="col">Lastname</th>
<th scope="col">Age</th>
</tr>
</thead>
<tbody>
<tr>
<td>Jon</td>
<td>Doe</td>
<td>21</td>
</tr>
<tr>
<td>Jon</td>
<td>Smith</td>
<td>22</td>
</tr>
</tbody>
</table>
Output:
6. Borderless Table:
The .table-borderless class removes the borders on all four sides of the table and around each cell.
<body>
<div class="container">
<h2>Bordered Table</h2>
<table class="table-bordered" style="width:100%">
<thead>
<tr>
<th>First name</th>
<th>Last name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
<tr>
<td>Jon</td>
<td>Doe</td>
<td>21</td>
</tr>
<tr>
<td>Jon</td>
<td>Smith</td>
<td>22</td>
</tr>
</tbody>
</table>
</div>
</body>