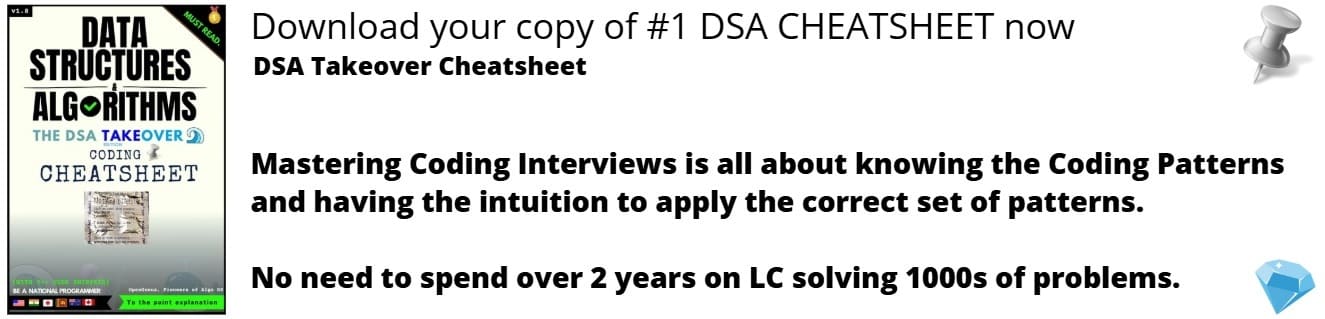
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article we are going to discuss about Digit Separators in C++. This topic is introduced in C++14. Using digit separators in the code make it easy for human readers to parse large numeric values.
Problem Detection
As we can see, everyone around us is in fond of comfort so when it comes to read a number having many digits, it seems sometimes difficult.
For example : Reading a number like 1000 is easy but when the number is like 1000000 it becomes little difficult and uncomfortable to read it and what if more zeros are added to it ?
Let's have a look at some problems below :
- Pronounce 345682384908
- Add 123456789 and 987654321
- Compare 34576807908 and 34576867908
We can solve it easily using programs but can we easily read it by our own?
The answer is 'NO'! This problem can be solved by using of Digit separators.
Solution
To solve this problem in our daily life we use commas (,) to separate digits.
Then the number 1000000 looks like 10,00,000 and can be easily read as 'Ten Lakhs'.
In a programming language we cannot use this commas so the concept of Digit Separators is introduced. Here a simple quotation mark (') is used to separate the digits. Now it becomes easier for us to read the number.
Implementation
A program to show that single quote marks are ignored while determining the value :
#include <iostream>
using namespace std;
int main()
{
long long int a = 10 '00' 000;
cout << a;
return 0;
}
Output : 1000000
A program to show that single quote marks are just for user's convenience and value of given number is not affected by there position :
#include <iostream>
using namespace std;
int main()
{
long long int a = 23'4'589;
long long int b = 23'45'89;
long long int c = 2345'89;
cout<<a<<" "<<b<<" "<<c;
return 0;
}
Output : 234589 234589 234589
From the above program it is clear that the use of digit separators does not affect the value of original number.
We can say that the numbers 23'456'76 , 2345'676 and 2'3456'7'6 represents the same value which can be written manually as 23,45,676 (twenty three lakhs forty five thousand six hundred and seventy six).
We can also use Digit separators in declaration of hexadecimal, octal and binary numbers in C++.
long long hexadecimal_no = 0xFFFA'FBFF;
long long octal_no = 00'43'02;
long long binary_no = 0b1000'1101;
Why not something else ?
One might be thinking that why only single quotation mark is used and why not space character or underscore?
- Underscore ( _ ) character is already taken in C++11 by user defined literals. So it will be confusing if we use it as a digit separator also.
- In case of comma (,) If we are thing a number like 1,23,456 and assign it to integer then compiler may not decide that you are passing three integers separately or only one integer in which digit separator is used. So again this is a bad idea.
- Space character ( ) i.e. white space also indicates that a new line is there. We know that C++ treats all white spaces equally. So again this is not sufficient to fulfill the condition why digit separators are used.
Due to this and many more different reasons a single quotation mark (') is selected as a digit separator to smoothen our work flow.