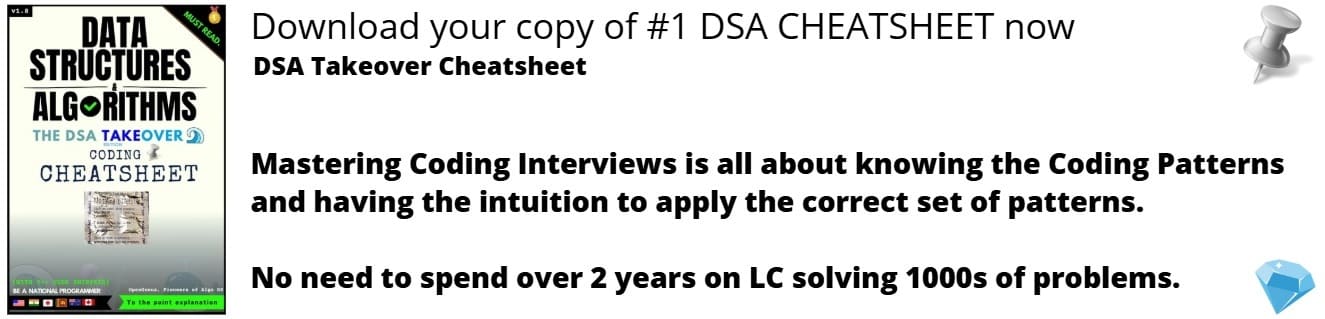
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we have explored different ways to dot product in Python Programming Language with and without Numpy.
Table of contents:
- Introduction to dot product
- Introduction to Matrix in Python and Numpy
- Different methods to do dot product
- Python: Naive approach in dot product
- Dot product in python without NumPy
- Dot product in Python with NumPy
Introduction to dot product
Mathematical process called the "dot product" produces a scalar from two vectors. The NumPy library, a well-known one for numerical computation, makes it simple to compute the dot product in Python.
The dot() function in NumPy may be utilised to calculate the dot product of two vectors. Here is an illustration:
import numpy as np
# define two vectors
a = np.array([1, 2, 3])
b = np.array([4, 5, 6])
# compute dot product
dot_product = np.dot(a, b)
print(dot_product)
To begin, we import NumPy and define the two vectors a and b in our example. The result is then saved in the dot_product variable after computing the dot product of a and b using the np.dot() method. The value of dot_product, which should be 32 (i.e., the dot product of a and b), is printed last.
Keep in mind that a pair of vectors' dot product can only be defined if they are the same dimensions. You will need to reshape the vectors or use a different method to compute the dot product if the vectors have different dimensions.
The scalar product and inner product are other names for the dot product. It accepts two vectors and gives back a scalar number that indicates how similar or aligned the two vectors are.
Two vectors a and b's mathematical dot product may be calculated as follows:
a . b = |a| |b| cos(theta)
where the vectors' magnitudes are |a| and |b| and the angle between them is theta.
The dot product may be used to quantify how much of one vector points in the same direction as the other in practical terms. The dot product is equal to the product of the vectors' magnitudes if the vectors are completely aligned (i.e., their angle is zero). The dot product is zero if the vectors are perfectly perpendicular, or if their angle is exactly 90 degrees.
Introduction to Matrix in Python and Numpy
In a matrix, which has two dimensions, integers are organised in rows and columns. For instance:
A matrix is a rectangular array of letters, numbers, or expressions in mathematics that are organised in rows and columns. The numpy package, which offers a variety of matrix functions and methods, is frequently used in Python to represent matrices.
The numpy.array() method, which accepts a list of lists as input, may be used to generate a matrix. A row of the matrix is represented by each inner list. Here's an example:
import numpy as np
# create a matrix with 2 rows and 3 columns
matrix = np.array([[1, 2, 3], [4, 5, 6]])
print(matrix)
Output :-
array([[1, 2, 3],
[4, 5, 6]])
A matrix is a rectangular array of numbers, symbols, or expressions that are organised in rows and columns in linear algebra. Linear equations, linear transformations, and many other mathematical ideas are represented and manipulated using matrices. The numpy package, which offers a variety of matrix functions and methods, is frequently used in Python to represent matrices.
The notation below can be used to express a matrix:
A = [ a11 a12 ... a1n ]
[ a21 a22 ... a2n ]
[ ... ... ... ... ]
[ am1 am2 ... amn ]
Different methods to do dot product
Dot products between two vectors can be calculated using a variety of techniques. Here are a few typical examples:
1.Standard Method
By multiplying the relevant elements of two vectors, the dot product is typically calculated. The results are then added. Consider two vectors with n elements each, a and b. The following formula may be used to get the dot product of a and b:
a · b = (a₁ * b₁) + (a₂ * b₂) + ... + (aₙ * bₙ)
where the elements of vector a, a1, a2,..., an are, and the elements of vector b, b1, b2,..., bn are.
In this case, if the vectors a and b are equal to [2, 4] and [3, 1], we may get their dot product as follows:
a · b = (2 * 3) + (4 * 1) + (1 * 5) = 6 + 4 + 5 = 15
A and B's dot product thus equals 15.
2.Geometric Method
Using the magnitudes of the two vectors and the angle between them, the dot product may be calculated geometrically. If is the angle between two vectors a and b, then we have two vectors a and b. The formula below may be used to determine the dot product of a and b:
a · b = ||a|| ||b|| cos(θ)
For instance, using the geometric technique, we can determine the dot product of two vectors a = [2, 4, 1] and b = [3, 1, 5] as follows:
||a|| = sqrt(2² + 4² + 1²) = sqrt(21)
||b|| = sqrt(3² + 1² + 5²) = sqrt(35)
Using the dot product formula, we can get the angle between the two vectors:
a · b = (2 * 3) + (4 * 1) + (1 * 5) = 6 + 4 + 5 = 15
The formula can then be changed such that cos() can be solved:
cos(θ) = (a · b) / (||a|| ||b||) = 15 / (sqrt(21) * sqrt(35)) = 0.591
Finally, the formula for the dot product may be rewritten by replacing the values of ||a||, ||b||, and cos():
a · b = ||a|| ||b|| cos(θ) = sqrt(21) * sqrt(35) * 0.591 = 15
Thus, the dot product of a and b is 15, matching the outcome of the conventional technique.
3.Matrix Method
Calculating the dot product of two vectors using the matrix method is a handy method. By multiplying the respective elements of the vectors and then adding the products, it is possible to determine the dot product of two vectors. The two vectors may be represented as matrices using the matrix technique, and the dot product can then be calculated by multiplying the matrices.
Consider the following two vectors:
a = [a1, a2, ..., an]
b = [b1, b2, ..., bn]
These vectors can be written as row matrices in order to be represented as matrices:
A = [a1, a2, ..., an]
B = [b1, b2, ..., bn]
In order to determine the dot product of a and b, we may now utilise matrix multiplication:
a · b = a1b1 + a2b2 + ... + anbn
= [a1, a2, ..., an][b1]
[b2]
[...]
[bn]
= A × B^T
where matrix B's transposition, BT, is. A 1 1 matrix that contains the dot product of the two vectors is the outcome.
Python: Naive approach in dot product
The dot product, sometimes referred to as the scalar product, is a method used in linear algebra to multiply two vectors to produce a scalar. One simplistic method for calculating the dot product of two vectors would be to run over each member of the two vectors in a for loop, multiply the corresponding elements, and then sum the results.
Here is some Python code that uses this strategy:
def dot_product(v1, v2):
"""
Computes the dot product of two vectors of equal length.
"""
if len(v1) != len(v2):
raise ValueError("Vectors must have the same length.")
result = 0
for i in range(len(v1)):
result += v1[i] * v2[i]
return result
Although this method works, it is ineffective for huge vectors. It is impracticable for use in machine learning or other applications where huge vectors are often used since as the size of the vectors rises, the computing time likewise climbs linearly.
More sophisticated algorithms, like the BLAS (Basic Linear Algebra Subprograms) library, are used to get around this inefficiency since they can do dot products significantly quicker by utilising specialised hardware and methods.
Dot product in python without NumPy
A mathematical procedure called the dot product takes two vectors of equal size and produces a single scalar result. The appropriate elements of each vector are multiplied to determine the dot product of two vectors, which is then determined by adding the answers.
The dot product may be computed in Python without the use of external libraries like NumPy by utilising loops and simple arithmetic operations. Here is how to accomplish it:
1.The first thing we must do is make sure the two vectors have the same size. We are unable to compute the dot product if the vectors have different widths.
2.Set up a variable to hold the value of the dot product. Set this variable to 0 to make it zero.
3.Multiply the appropriate elements of each of the two vectors as you iterate through each element, then add the result to the dot product variable.
4.Get the dotted product value back.
Here is an example of how to use Python to implement the dot product without NumPy :
def dot_product(vector1, vector2):
"""
Calculates the dot product of two vectors.
Args:
vector1 (list): First vector.
vector2 (list): Second vector.
Returns:
dot_product (float): The dot product of the two vectors.
"""
# Ensure the two vectors are of the same length
if len(vector1) != len(vector2):
raise ValueError("Vectors must have the same length")
# Initialize the dot product to zero
dot_product = 0
# Calculate the dot product
for i in range(len(vector1)):
dot_product += vector1[i] * vector2[i]
return dot_product
Dot product in Python with NumPy
The mathematical operation known as the dot product of two vectors in linear algebra takes two equal-length sequences of integers and produces a single number. It is also referred to as the scalar product or inner product of two vectors. The dot product is described as the product of the equivalent items in the two sequences added together.
Using the numpy.dot()
method in NumPy, you may get the dot product of two arrays. This function accepts two input arrays and outputs a scalar value. The following is how to compute the dot product:
dot_product = a[0]*b[0] + a[1]*b[1] + ... + a[n-1]*b[n-1]
where n is their combined length, and a and b are the two arrays.
The @ operator is another option for calculating the dot product. The numpy.dot() method is similar to using this operator, which was introduced in Python 3.5.
Here is an example using NumPy showing how to compute the dot product of two arrays:
import numpy as np
#define two arrays
a = np.array([1, 2, 3])
b = np.array([4, 5, 6])
#calculate the dot product using numpy.dot()
dot_product = np.dot(a, b)
#calculate the dot product using the @ operator
dot_product2 = a @ b
print(dot_product) # output: 32
print(dot_product2) # output: 32
In this example, numpy.dot() and the @ operator are used to compute the dot product of the two arrays [1, 2, 3] and [4, 5, 6]. 32 is the final dot product.
Comparison With NumPy and Without NumPy of Dot product in python
With NumPy | Without NumPy |
---|---|
The sum of the products of related items in two vectors or matrices is computed using the mathematical operation known as the dot product in Python. Both using and without the numpy module, Python may implement the dot product. | Without Numpy we build two Python lists, a and b, and then use a for loop and the sum() method to compute their dot product. |
Conclusion
In Python, a vector is usually referred to as two numpy arrays. The behaviour of numpy.dot() and the '*' operator varies on them. It's important to be aware of this, especially if you're working on a topic requiring data science or competitive programming.