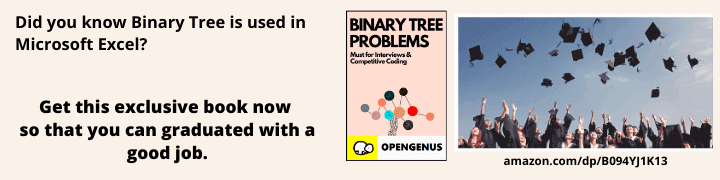
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we are going to learn about the Fastest and Smallest types of Fixed width integer types in C++ such as int_least8_t, int_fast8_t and more.
The basic data types in C or C++ are char, short, int, and long, each of which allows 1, 2, or 4,8 bytes in memory, respectively. However, this byte can vary depending on the compiler, operating system, or hardware used.
On a 32-bit compiler, the Long data type allocates 4 bits in memory, whereas, on a 64-bit compiler, it allocates 8 bytes in memory.In other words, the data type's size depends on the compiler.
To overcome this problem, C has provided a fixed width integer type which allocates a certain amount of memory's bytes.
Fixed width integer type :
Integral data types with a fixed number of bits are called fixed-width integers.As the name suggests, it is useful to allocate a fixed number of bytes in memory .
To define fixed-width integers, a programmer must add the cstdint> header file within the std namespace.
For data types like short, int, and long, C++ specifies the least byte. whereas Fixed-width integers ensure a certain size, hence they are not portable because not every platform supports them.
Example : int32_t - integer with a fixed memory size of 32 bytes.
There are two types of fixed type integers.
1. Singed :
intX t : singed integer with X bytes in memory.
2. Unsinged :
uintX t: unsigned integer containing X bytes in memory.
Where x is the number of bytes stored in memory (4,8,16,32).
Advantage:
Provides a fixed byte size in memory.
Disadvantage:
It is not portable.
Types of Fixed-width integer
C++ supports three Fixed-width integer types .
- Fixed size of Integer : the size you user select
- Smallest Fixed size of integer : whose size is at least the size useruser specify
- Fastest Fixed size of integer : whose size is at least the size you specify.
Fixed size of Integer
It contain fixed size of bit.They were further divided into two groups.
1. Singed fixed integer
signed integers usually defined by int(no_of_byte)_t types .Here is List of the fixed signed integer types is:
- std::int8_t define 1 byte signed data type.
- std::int16_t define 16 byte signed data type.
- std::int32_t define 32 byte signed data type.
- std::int64_t define 64 byte signed data type.
2. Unsigned fixed integer
unsigned integers are defined by uint(no_of_byte)_t . Here is List of the fixed unsigned integer types is:
- std::uint8_t define 1 byte unsigned data type.
- std::uint16_t define 16 byte unsigned data type.
- std::uint32_t define 32 byte unsigned data type.
- std::uint64_t define 64 byte unsigned data type.
From above table you will get idea that signed integers usually defined by int(no_of_byte)_t types or unsigned integers are defined by uint(no_of_byte)_t .
Example :
#include <cstdint>
#include <iostream>
int main()
{
std::int16_t i{16};
std::cout << "data :"<<i;
return 0;
}
Output :
data : 5
Smallest Fixed width integer
This type provide the Smallest signed or unsigned integer type with a width of at least bit .This type include 8, 16, 32, or 64 byte.
They were further divided into two groups.
- Smallest Signed Fixed width integer
- Smallest Unsigned Fixed width integer
Smallest Signed Integer
Smallest signed integer type include width of at least 8, 16, 32 and 64 bits.
here is a list of the Smallest signed types is:
- int_least8_t
- int_least16_t
- int_least32_t
- int_least64_t
Smallest Unsigned Integer
Smallest unsigned integer type include width of at least 8, 16, 32 and 64 bits .
here is a list of the Smallest Unsigned types is:
- uint_least8_t
- uint_least16_t
- uint_least32_t
- uint_least64_t
Example :
#include <cstdint>
#include <iostream>
//main code
int main()
{
std::cout << "Smallest signed integer in C++ ";
std::cout << '\n';
std::cout << "least 8 bit : " << sizeof(std::int_least8_t) * 8 << " bits\n";
std::cout << "least 16 bit: " << sizeof(std::int_least16_t) * 8 << " bits\n";
std::cout << "least 32 bit: " << sizeof(std::int_least32_t) * 8 << " bits\n";
std::cout << "least 64 bit: " << sizeof(std::int_least64_t) * 8 << " bits\n";
std::cout << '\n';
std::cout << "Smallest unsigned integer types in C++ ";
std::cout << '\n';
std::cout << "least 8 bit : " << sizeof(std::uint_least8_t) * 8 << " bits\n";
std::cout << "least 16 bit: " << sizeof(std::uint_least16_t) * 8 << " bits\n";
std::cout << "least 32 bit: " << sizeof(std::uint_least32_t) * 8 << " bits\n";
std::cout << "least 64 bit: " << sizeof(std::uint_least64_t) * 8 << " bits\n";
return 0;
}
Output :
Smallest signed integer in C++
least 8 bit : 8 bits
least 16 bit: 16 bits
least 32 bit: 32 bits
least 64 bit: 64 bits
Smallest unsigned integer types in C++
least 8 bit : 8 bits
least 16 bit: 16 bits
least 32 bit: 32 bits
least 64 bit: 64 bits
Fastest Fixed width integer
This type provide the fastest signed or unsigned integer type with a width of at least bit .which include 8, 16, 32, or 64 bit memory .
They were further divided into two groups.
- Fastest signed integer
- Fastest Unsigned integer
Fastest Signed Integer
This Fastest signed integer type inlcude width of at least 8, 16, 32 and 64 bits.
List of the Fastest signed types is:
- int_fast8_t
- int_fast16_t
- int_fast32_t
- int_fast64_t
Fastest Unsigned Integer
This Fastest unsigned integer type inlcude width of at least 8, 16, 32 and 64 bits.
List of the Fastest Unsigned types is:
- uint_fast8_t
- uint_fast16_t
- uint_fast32_t
- uint_fast64_t
Example :
#include <cstdint>
#include <iostream>
//main code
int main()
{
std::cout << "Fastest Signed Integer of Fixed width integer types in C++ ";
std::cout << '\n';
std::cout << "Fastest 8: " << sizeof(std::int_fast8_t) * 8 << " bits\n";
std::cout << "Fastest 16: " << sizeof(std::int_fast16_t) * 8 << " bits\n";
std::cout << "Fastest 32: " << sizeof(std::int_fast32_t) * 8 << " bits\n";
std::cout << "Fastest 64: " << sizeof(std::int_fast64_t) * 8 << " bits\n";
std::cout << '\n';
std::cout << "Fastest Unsigned Integer of Fixed width integer types in C++ ";
std::cout << '\n';
std::cout << "Fastest 8: " << sizeof(std::uint_fast8_t) * 8 << " bits\n";
std::cout << "Fastest 16: " << sizeof(std::uint_fast16_t) * 8 << " bits\n";
std::cout << "Fastest 32: " << sizeof(std::uint_fast32_t) * 8 << " bits\n";
std::cout << "Fastest 64: " << sizeof(std::uint_fast64_t) * 8 << " bits\n";
return 0;
}
Output :
Fastest Signed Integer of Fixed width integer types in C++
Fastest 8: 8 bits
Fastest 16: 64 bits
Fastest 32: 64 bits
Fastest 64: 64 bits
Fastest Unsigned Integer of Fixed width integer types in C++
Fastest 8: 8 bits
Fastest 16: 64 bits
Fastest 32: 64 bits
Fastest 64: 64 bits
let us take one more another example .
Example :
#include <cstdint>
#include <iostream>
//main code
int main()
{
std::cout << "Smaller types of Fixed width integer types in C++ ";
std::cout << '\n';
std::cout << "least 8 bit : " << sizeof(std::int_least8_t) * 8 << " bits\n";
std::cout << "least 16 bit: " << sizeof(std::int_least16_t) * 8 << " bits\n";
std::cout << "least 32 bit: " << sizeof(std::int_least32_t) * 8 << " bits\n";
std::cout << "least 64 bit: " << sizeof(std::int_least64_t) * 8 << " bits\n";
std::cout << '\n';
std::cout << "Fastest types of Fixed width integer types in C++ ";
std::cout << '\n';
std::cout << "Fastest 8: " << sizeof(std::int_fast8_t) * 8 << " bits\n";
std::cout << "Fastest 16: " << sizeof(std::int_fast16_t) * 8 << " bits\n";
std::cout << "Fastest 32: " << sizeof(std::int_fast32_t) * 8 << " bits\n";
std::cout << "Fastest 64: " << sizeof(std::int_fast64_t) * 8 << " bits\n";
return 0;
}
Output :
Smaller types of Fixed width integer types in C++
least 8 bit : 8 bits
least 16 bit: 16 bits
least 32 bit: 32 bits
least 64 bit: 64 bits
Fastest types of Fixed width integer types in C++
Fastest 8: 8 bits
Fastest 16: 64 bits
Fastest 32: 64 bits
Fastest 64: 64 bits
Clarification:
This output will vary from system to system. The value of std::fast16_t is 16 bits, whereas the value of std::int_fast32_t is 64 bits. Because 32-bit integers are faster to process than 16-bit integers.
Conclusion :
In real-world problems, fixed-width integer types are helpful in writing portable and efficient code.