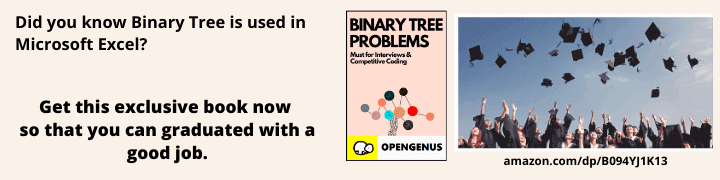
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored why a C++ code using fscanf cannot read next line and how to fix it by using getline() and sscanf() in C++ by keeping the syntax same. We have demonstrated this with C++ code examples.
Table of contents:
- Understanding the problem and fix
- fscanf not reading next line
- Fix with C++ code
Understanding the problem and fix
This is the major problem with using fscanf to read data from a text file. The issue is fscanf does not recognize new line character (\0
).
The fix is to:
- Read each line one by one using getline()
- Extract data from each line using sscanf() just like fscanf() (same syntax)
fscanf not reading next line
Let the file (opengenus.txt) be of the following format:
10, 53, 101
-9, 33, 10
44, 2, 1
1, 2, 3
5, 0, 5
...
#include <string.h>
#include <cstdlib>
int main(int argc, char** argv) {
FILE* my_file = fopen("opengenus.txt", "r");
int data[100][3];
for(int count = 0; count < 100; count++) {
int got = fscanf(my_file, "%d,%d,%d", data[count][0],
data[count][2], data[count][2]);
}
}
The issue with the above C++ code is that fscanf cannot read the next line and reads only the first line of the file.
Fix with C++ code
Following is the correct C++ code:
#include <string.h>
#include <cstdlib>
int main(int argc, char** argv) {
FILE* my_file = fopen("opengenus.txt", "r");
char* line = NULL;
size_t len = 0;
int data[100][3];
for(int count = 0; count < 100; count++) {
size_t read = getline(&line, &len, my_file);
int got = sscanf(line, "%d,%d,%d", data[count][0],
data[count][2], data[count][2]);
}
}
With this article at OpenGenus, you must have the complete idea of how to fix the problem of fscanf not reading next line.