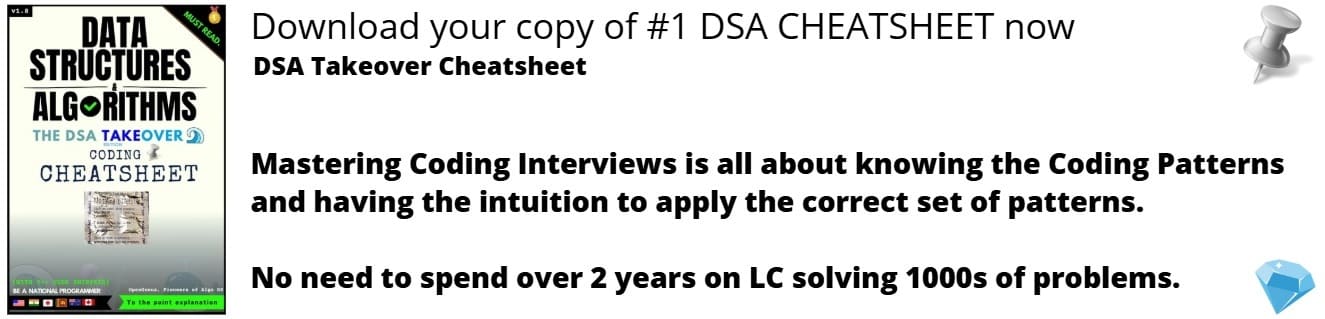
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored how to initialize struct in C Programming Language using different techniques such as designated initializer, dot operator and more.
Only a single data type can be stored in an array (if you declare a char type, you cannot store anything other than char type), but by using the structure in C language, we can combine variables with different data types into one.
Table of Contents :
- Introduction
- USE A Struct
- Structure initialization
- Initialization at Declaration
- Initialization using Designated Initializer
- Initialized at compile time using the dot(.) operator
- When should the structure be initialized?
- Conclusion
INTRODUCTION
A structure is a function that allows you to collectively manage multiple values.
It can also be said that it is a function that creates a new data type by gathering multiple data types.
struct in simpler terms can be said to be the keyword for "I'm going to define a structure now."
Arrays are also a function that can handle multiple values collectively, but whereas arrays are collections of the same data type, structures can collectively manage different data types.
A variable defined in a structure is called a member variable .
e.g.:
struct Subject
{
char name[20];
int totalMarks;
float marks;
};
Basic steps to write a struct in C :
- Write the structure name following struct. You can decide the structure name as you like.
- Next, create a block with curly braces {}.
- Inside this block, define one or more variables that you want to use in your structure.
- In the sample code, name is defined as a char type array, marks is defined as an int type, and a structure is created to store Subject information.
- Don't forget to end the block with a semicolon (;).
- A function definition didn't need a semicolon, so it's easy to forget it in a struct definition.
USE A Struct
#include <stdio.h>
#include <string.h>
struct Person
{
char name[30];
int age;
char gender;
};
int main()
{
struct Person person = { "John", 25, 0 };
printf(
"name: %s\n"
"age: %d\n"
"gender: %d\n",
person.name, person.age, person.gender);
getchar();
}
//Output
name: John
age: 25
gender: Male
We use the dot operator to access member variables from structure variables.
Write "." (dot, period) after the structure variable, and then write the name of the member variable you want to use.
Structure initialization in C
C language offers us many ways to initialize a structure in our program. We can use the following initialization method to initialize struct:
- Initialization at Declaration
- Initialization using Designated Initializer
- Initialized at compile time using the dot(.) operator
Initialization at Declaration
Structure variables can be initialized at the same time they are declared, just like normal variables and arrays.
General form:
structure type structure variable = { value1, value2, ... };
struct Book
{
char name[80];
int price;
int publishYear;
};
struct Patient p1 = { "AdventureOfTom" , 400, 2020 };
Initialization using Designated Initializer
Following C code demonstrates Initialization of struct using Designated Initializer:
struct pet {
char name [20];
int age;
double weight;
};
int main(void) {
struct pet dog = {
.name="Matty",
.age = 4,
.weight = 8.2,
};
return 0;
}
Note : Order of assigning the variables do not matter.
Initialized at compile time using the dot(.) operator
Structure variable can be initialized at compile time using the dot(.) operator.
Initialization that directly assigns values to members of structure variables.
Example :
struct student {
char name [20];
int age;
int marks;
};
int main(void) {
struct student S1;
S1.name = John; //initialization of each member separately
S1.marks = 93;
S1.age = 13;
}
When should the structure be initialized?
First of all, the structure may be initialized when the structure variable is created.
By initializing the structure variables by clearing them to 0, bugs related to the variables can be reduced.
If the structure has a pointer it may contain a random value unless it is initialized.
In the code after that, it is not possible to judge whether the value of the pointer is a proper memory address or a random value that has not been initialized.
Therefore, if you clear the structure variable to 0 and set the pointer to NULL when creating the structure variable for the first time, you can reduce the bug errors.
Conclusion
We have seen the initialization of structures in C language in this article at OpenGenus. We found out that there are a multiple ways to initialize the structure like below:
- Initialization at Declaration
- Initialization using Designated Initializer
- Initialized at compile time using the dot(.) operator
Which one to use solely depends on the judgment of the programmer.