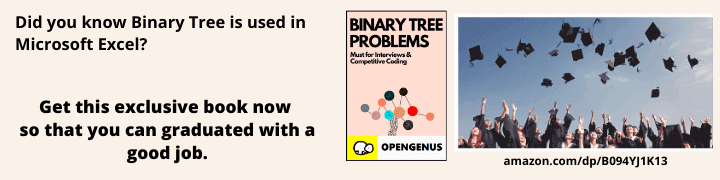
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 45 minutes
Inheritance is the process by which objects of one class acquire the properties of objects of another class. It supports the concept of hierarchical classification. The principle behind this sort of division is that each derived class shares common characteristics with the class from which it is derived.
Inheritance enforces a relationship between various code components and gives it a structure and a good opportunity for code reuse. Consider that you have a large class A and you need another class B which will have only one member function different. In this case, class B can be built by inheriting from class A and adding/ overriding the member function. This shows that class A and B are related and there is no code duplication.
In this case, class A is called base class and class B is called derived class.
Consider the following example, in which, 'Flying bird' and Non-Flying Bird' class are the subclass of 'Bird' class. They inherit some attributes from 'Bird' class.
Now,'Robin' and 'Swallow' class are subclass of 'Flying Bird'. Similarly 'Penguin' and 'Kiwi' are subclass of 'Non-Flying Bird'.
- The principle behind this sort of division is that each derived class shares common characteristics with the class from which it is derived.
Purpose of inheritance
In OOP, the concept of inheritance provides the idea of reusability. This means that we can add additional features to an existing class without modifying it. This is possible by deriving a new class from the existing one.
Different types of inheritance
The different types of inheritance are:
- Single inheritance
- Multilevel inheritance
- Hierarchical inheritance
- Hybrid inheritance
- Multipath inheritance
- Multiple inheritance
1. Single Inheritance
- In single inheritance, a class is allowed to inherit from only one class. i.e. one sub class is inherited by one base class only.
-
In the above diagram, Class B is derived from Class A. Class A is base class and Class B is a derived class.
-
Example-
In the above figure, 'Non-Flying Bird' is the subclass of class 'Bird'. Since 'Non-Flying Bird' inherits some attributes of 'Bird' class.
2. Multilevel Inheritance
- In Multilevel inheritance, one class is derived from a class which is also derived from another class.
- In the above diagram, Class C is derived from class B which is derived from Class A. So, class A is called base class and class B is intermediate base class.
- Example-
In the above figure, 'Non-Flying Bird' is a subclass of 'Bird' and 'Kiwi' is a subclass of 'Non-Flying Bird'.
Here the 'Bird' class serves as a base class for the derived class 'Non-Flying Bird' ,which in turn serves as a base class for the derived class 'Kiwi'. The class 'Non-Flying Bird' is known as intermediate base class since it provides a link for the inheritance between 'Bird' and 'Kiwi'.
3. Hierarchical Inheritance
- In hierarchical inheritance, there are multiple classes derived from one class.
- In this case, multiple derived classes allow to access the members of one base class. Several features of one level can be shared by many others below that level.
- In the above diagram, Class B and Class C are derived from the base class A.
- Example-
In the above figure, a hierarchical classification of birds is shown. Here there are two derived classes 'Non-Flying Bird' and 'Flying Bird' accesses the members of one base class 'Bird'.
4. Hybrid Inheritance
- In hybrid inheritance, there could be some situations where we need to apply two or more types of inheritance to design a program.
- In the above figure,there a combination of hierarchical and mulltilevel inheritance. Classes B and C are derived from base class A, further classes D and E are derived from Class B and C.
- Example-
In the above figure, There are two types of inheritance hierarchical and multiple inheritance. Here, 'Non-Flying Bird' and 'Flying Bird' classes are derived from base class 'Bird'. 'Kiwi' and 'Robin' classes are derived from the intermediate base classes 'Non-Flying Bird' and 'Flying Bird' respectively. - In this type of inheritance, derived class have multiple paths to a base class, a diamond problem occurs. It will result in duplicate inherited members of the base class.
- To avoid this problem easily use Virtual Inheritance.
5. Multipath Inheritance
- In multipath inheritance, a class is derived from other derived classes which are derived from the same base class.
- In this type of inheritance, other types of inheritance are involved like multiple, multilevel, hierarchical etc.
- It is famously known as diamond problem in computer programming.
- In the above figure, Class B and C are derived from base class A. Further, class D is derived from class B and C.
- Example-
In the above figure, class 'Exam' and 'Sport' are derived from class 'Student'. 'Result' class is derived from the two derived classes 'Exam' and 'Sports'. - Both derived classes inherits the features of base class. Hence when we derive a new class by inheriting features from these two classes derived from first base is inherited to the finally derived class from two paths.
6. Multiple Inheritance
- In multiple inheritence, a class can be derived from more than one parents.
In the above figure, Class A is derived from class B and C. - Example-
In the above figure, a class 'Bat' is derived from base classes 'Mammal' and 'WingedAnimal'. It makes sense because bat is mammal as well as a winged animal.
Advantages and Disadvantages of using Inheritance
1. Advantages
- Reusability-The capability to utilize methods relating to a base class with no need of re-scripting the similar.
- Data hiding-The base class can decide to make some data private with the purpose it is not changed via the derived class.
- Overriding-With inheritance, we will be able to override the methods of the base class so that meaningful implementation of the base class method can be designed in the derived class.
- Extensibility-extending the base class logic as per business logic of the derived class.
Disadvantages
- Improper use of inheritance may lead to wrong solutions.
- Often,data members in the base class are left unused which may lead to memory wastage.
- Inherited functions works slower than the normal function as there is indirection.