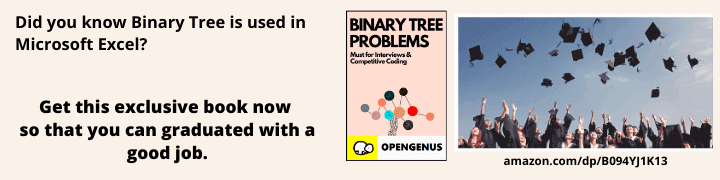
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 25 minutes | Coding time: 10 minutes
Operator overloading is an important concept in C++
. It is a type of polymorphism
in which an operator is overloaded to give user defined meaning to it. Overloaded operator is used to perform operation on user-defined data type. For example '+' operator can be overloaded to perform addition on various data types, like for Integer, String(concatenation) etc.
In simple terms, Operator overloading is a compile-time polymorphism in which the operator is overloaded to provide the special meaning to the user-defined data type. Operator overloading is used to overload or redefines most of the operators available in C++. It is used to perform the operation on the user-defined data type. For example, C++ provides the ability to add the variables of the user-defined data type that is applied to the built-in data types.
That is, Overloaded operators are functions with special names: the keyword operator
followed by the symbol for the operator being defined. Like any other function, an overloaded operator has a return type and a parameter list.
The advantage of Operators overloading is to perform different operations on the same operand.
Almost any operator can be overloaded in C++. However there are few operator which can not be overloaded.
Operator that are not overloaded are follows :-
- scope operator -
::
sizeof
- member selector -
.
- member pointer selector -
*
- ternary operator -
?:
Syntax of Operator Overloading
return_type class_name : : operator op(argument_list)
{
// body of the function.
}
- Where the
return type
is the type of value returned by the function. class_name
is the name of the class.- operator op is an operator function where op is the operator being overloaded, and the operator is the keyword.
Implementing Operator Overloading in C++
Operator overloading can be done by implementing a function which can be :
- Member Function
- Non-Member Function
- Friend Function
Operator overloading function can be a member function if the Left operand is an Object of that class, but if the Left operand is different, then Operator overloading function must be a non-member function.
Operator overloading function can be made friend function if it needs access to the private
and protected
members of class.
Rules for Operator Overloading
- Existing operators can only be overloaded, but the new operators cannot be overloaded.
- The overloaded operator contains atleast one operand of the user-defined data type.
- We cannot use friend function to overload certain operators. However, the member function can be used to overload those operators.
- When unary operators are overloaded through a member function take no explicit arguments, but, if they are overloaded by a friend function, takes one argument.
- When binary operators are overloaded through a member function takes one explicit argument, and if they are overloaded through a friend function takes two explicit arguments.
- Precedence and Associativity of an operator cannot be changed.
- Arity (numbers of Operands) cannot be changed. Unary operator remains unary, binary remains binary etc.
- Cannot redefine the meaning of a procedure. You cannot change how integers are added.
C++ Operators Overloading Example: unary operator ++
Example - 1 :-
Let's see the simple example of operator overloading in C++. In this example, void operator ++ () operator function is defined (inside Test class).
Program to overload the unary operator ++ :-
# include<iostream>
using namespace std;
class Test
{
private:
int num;
public:
Test(): num(8){}
void operator ++() {
num = num+2;
}
void Print() {
cout<<"The Count is: "<<num;
}
};
int main()
{
Test tt;
++tt; // calling of a function "void operator ++()"
tt.Print();
return 0;
}
Output :-
The Count is: 10
C++ Operators Overloading Example: binary operator +
Example - 2 :-
Let's see a simple example of overloading the binary operators.
Program to overload the binary operators:-
# include<iostream>
using namespace std;
class A
{ int x;
public:
A(){}
A(int i)
{
x=i;
}
void operator+(A);
void display();
};
void A :: operator+(A a)
{
int m = x+a.x;
cout<<"The result of the addition of two objects is : "<<m;
}
int main()
{
A a1(5);
A a2(4);
a1+a2;
return 0;
}
Output :-
The result of the addition of two objects is : 9
C++ Operators Overloading Example: Arithmetic Operator +
Example - 3 :-
Arithmetic operator are most commonly used operator in C++. Almost all arithmetic operator can be overloaded to perform arithmetic operation on user-defined data type.
In the below example we have overridden the +
operator, to add to Time(hh:mm:ss) objects.
# include<iostream>
using namespace std;
class Time
{ int h,m,s;
public:
Time()
{
h=0, m=0; s=0;
}
void setTime();
void show()
{
cout<< h << ":"<< m<< ":"<< s;
}
//overloading '+' operator
Time operator+(time);
};
Time Time::operator+(Time t1) //operator function
{
Time t;
int a,b;
a = s+t1.s;
t.s = a%60;
b = (a/60)+m+t1.m;
t.m = b%60;
t.h = (b/60)+h+t1.h;
t.h = t.h%12;
return t;
}
void time::setTime()
{
cout << "\n Enter the hour(0-11) ";
cin >> h;
cout << "\n Enter the minute(0-59) ";
cin >> m;
cout << "\n Enter the second(0-59) ";
cin >> s;
}
void main()
{
Time t1,t2,t3;
cout << "\n Enter the first time ";
t1.setTime();
cout << "\n Enter the second time ";
t2.setTime();
t3 = t1 + t2; //adding of two time object using '+' operator
cout << "\n First time ";
t1.show();
cout << "\n Second time ";
t2.show();
cout << "\n Sum of times ";
t3.show();
getch();
}
While normal addition of two numbers return the sumation result. In the case above we have overloaded the +
operator, to perform addition of two Time
class objects. We add the seconds, minutes and hour values separately to return the new value of time.
In the setTime()
funtion we are separately asking the user to enter the values for hour, minute and second, and then we are setting those values to the Time
class object.
First two are values of t1
and t2
and the third is the result of their addition.
Output :-
1:20:30
2:15:25
3:35:55
Important points about operator overloading
- For operator overloading to work, at leas one of the operands must be a user defined class object.
- Assignment Operator: Compiler automatically creates a default assignment operator with every class. The default assignment operator does assign all members of right side to the left side and works fine most of the cases (this behavior is same as copy constructor). See this for more details.
- Conversion Operator: We can also write conversion operators that can be used to convert one type to another type.
- Overloaded conversion operators must be a member method. Other operators can either be member method or global method.
- Any constructor that can be called with a single argument works as a conversion constructor, means it can also be used for implicit conversion to the class being constructed.