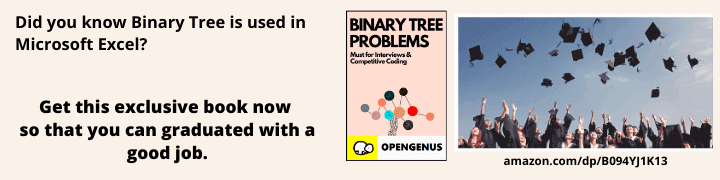
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 35 minutes
The central idea of encapsulation and data hiding concept is that any non-member function has no access permission to the private data of the class. The private members of the class are accessed only from member functions of that class.
C++ allows a mechanism, in which a non-member function has access permission to the private members of the class. This can be done by declaring a non-member function friend to the class whose private data is to be accessed. The friend is a keyword. Consider the following example.
class ac
{
private:
char name [15];
int acno;
float bal;
public:
void read();
friend void showbal();
};
The keyword friend must precede the function declaration, whereas function declarator must not. The function can be defined at any place in the program like normal function. The function can be declared as friend function in one or more classes. The keyword friend
or scope access operator must not precede the definition of the friend function. The declaration of friend function is one inside the class in private or public part and a function can be declared as friend function in any number of classes. These functions use objects as arguments. Thus the following statement is wrong.
friend void::showbal (ac a) //Wrong function definition
{
statement 1;
statement 2;
}
The above declaration of function is wrong because the function declarator precedes the keyword friend.
The friend functions have the following properties:
-
There is no scope restriction for the friend function; hence, they can be called directly withot using objects.
-
Unlike member functions of class, the friend cannot access the member directly. On the other hand it uses object and dot operator to access the private and public member variables of the class.
-
By default, friendship is not shared(mutual). For example, if class X is declared as friend of Y, this does not meant that Y has privileges to access private members of class X.
-
Use of friend functions is rare, since it violates the rule of encapsulation and data hiding.
-
The function can be declared in public or private sections without changing its meaning.
Sample Program
#include <iostream>
class ac
{
private:
char name[15];
int accno;
float bal;
public:
void read()
{
std::cout << "\nName :";
std::cin >> name;
std::cout << "\nA/c No. :";
std::cin >> accno;
std::cout << "\nBalance :";
std::cin >> bal;
}
// Friend function declaration
friend void showbal (ac);
};
void showbal (ac a)
{
std:: cout << "\n Balance of A/c no. " << a.accno << "is Rs. " << a.bal;
}
int main()
{
ac k;
k.read();
showbal(k); // call to friend function
return 0;
}
Output
Name:Shreya
A/c No. : 911
Balance : 87695
Balance of A/c no. 911 is Rs.87695
EXPLANATION
In the above program, class ac is declared. It has three member variables and one member function.
Also, inside the class ac, showbal()
is a function, which is declared as friend of the class ac. Once the outside function is declared as friend to any class, it gets an authority to access the private data of that class. The fucntion read()
reads the data through the keyboard such as name, accoount number, and balance. Th friend function showbal()
display the balance and acno.
friend class
A friend class is a class that cn access the private and protected members of a class in which it it declared as friend. This is needed when we want to allow a particular class to access the private and protected members of a class. Declaring class A to be friend of class B does not meant class B a friend of class A; that is, friendship is not exchageable.
Sample Program
#include <iostream>
class XYZ {
private:
char ch='A';
int num = 11;
public:
/* This statement would make class ABC
* a friend class of XYZ, this means that
* ABC can access the private and protected
* members of XYZ class.
*/
friend class ABC;
};
class ABC {
public:
void disp(XYZ obj){
std::cout<<obj.ch<<endl;
std::cout<<obj.num<<endl;
}
};
int main() {
ABC obj;
XYZ obj2;
obj.disp(obj2);
return 0;
}
Output
A
11
Merits and Demerits of friend function
Merits:
- Using friend function is generally syntatic, i.e, both member function and friend function are equally privileged.
- A friend function can be called like f(obj), where a member is called like obj.f().
- friend fucntion are used when two or more classes are designed to be more tightly coupled than you want for.
Demerits:
- They don't bind dynamically, i.e, they don't respond to polymorphism.
- A derived class does not inherit friend function.
- Friend functions can not have a storage class specifier i.e they can not be declared as static or extern.
Limitations of friend function
- In C++, friendship is not inherited. If a base class has a friend function, then the function doesn’t become a friend of the derived class(es).
For example, following program prints error because show() which is a friend of base class A tries to access private data of derived class B.
#include <iostream>
class A
{
protected:
int x;
public:
A() { x = 0;}
friend void show();
};
class B: public A
{
public:
B() : y (0) {}
private:
int y;
};
void show()
{
B b;
std::cout << "The default value of A::x = " << b.x;
// Can't access private member declared in class 'B'
std::cout << "The default value of B::y = " << b.y;
}
int main()
{
show();
getchar();
return 0;
}