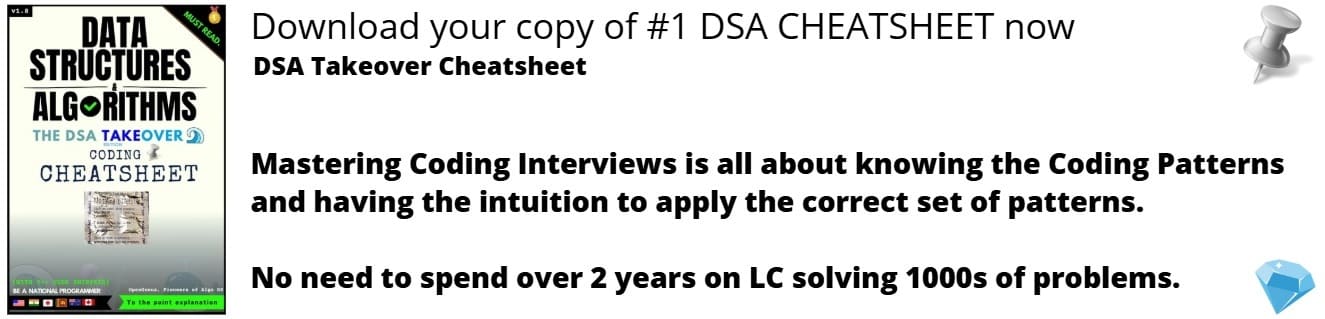
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of Content
- Summary
- What i used to create language translation program in Python
- Example
- My Program
- Other available APIs and approaches
Summary:
In this article at OpenGenus, we will be talking about how I create a language translation program using the python 3 programming language. This program is able to translate user input from english to arabic. I used the Google Translation API to translate languages and in order to add more functionality to my program I used functions and if statement to create a restart option so that once the program has finished executing the user will have an option to run it again.
What I used to create language translation program in python:
In order to create a language translator program in python I used imported two modules which is the Translator and Constants from the googletrans package which is from the Google Translation API.
The Translator module provides a way to use the Google Translate API to translate text from one language to another. It allows you to specify the input text, the source language, and the target language for the translation.
The constants module contains constants that are used by the Translator module, such as language codes and error messages.
Together, these modules allow you to easily integrate Google Translate functionality into a Python program.
Example:
In this sample code we can see the input text being in spanish "Hola Mundo" and the output shows the original text as well as the translation into english. In order to run this code you just have to click triangle shaped button at the top right. The way the code works is basically we are importing our package which is the Translator package from the google translate API and then we do translator = Translator()
to initialize the package and translation = translator.translate("Hola Munda")
which translates the given spanish text to english by default. After that in order to print all this information we do print(f"{translation.origin} ({translation.src}) --> {translation.text} ({translation.dest})")
which creates a string that prints the original text and the translated text in the terminal.
My Program:
from googletrans import Translator, constants
from pprint import pprint
def translator():
# init the Google API translator
translator = Translator()
# translate a spanish text to english text (by default)
translation = translator.translate(input("type here: "), src="en", dest="ar")
output = (f"{translation.origin} ({translation.src}) --> {translation.text} ({translation.dest})")
print(output)
restart()
def restart():
option = input("do u wanna repeat, type y for yes and n for no: ")
if (option == "y"):
translator()
else:
print("bye")
translator()
This code is a Python script that utilizes the Google Translate API to translate a user's input from english to arabic and output the translation to the console. Here is a breakdown of what each part of the code does:
We imports the Translator and constants classes from the googletrans module by doing from googletrans import Translator
and from pprint import pprint
imports the pprint function from the pprint module, which is used to print the output of the translator()
function. def translator():
defines a function called translator()
. The translator = Translator()
initializes a Translator object from the googletrans module.
translation = translator.translate(input("type here: "), src="en", dest="ar")
translates the user's input from English to Arabic and stores the result in a translation object. output = (f"{translation.origin} ({translation.src}) --> {translation.text} ({translation.dest})")
creates a string that displays the original text and the translated text and assigns it to the output variable.
The restart()
calls the restart()
function, which prompts the user to either repeat the translation process or exit the program. The def restart():
defines a function called restart()
. The option = input("do u wanna repeat, type y for yes and n for no: ")
prompts the user to enter either "y" or "n" to indicate whether they want to repeat the translation process or exit the program. if (option == "y"):
checks if the user entered "y" the translator()
calls the translator()
function to restart the translation process if the user entered "y" and if not then this will be executed else: print("bye")
to the console and exits the program if the user entered "n".
Other available APIs and approaches:
There are other APIs and approaches you can use to create a language translation program in Python such as the ones listed below:
Microsoft Translator Text API - Microsoft offers a cloud-based Translator Text API that provides translation for over 70 languages. You can use the translate() method from the microsofttranslator Python package to interact with this API.
Amazon Translate - Amazon provides a cloud-based machine translation service called Amazon Translate, which can translate text from one language to another. You can use the boto3 Python package to interact with this API.
OpenNMT - OpenNMT is an open-source neural machine translation toolkit that you can use to train your own translation models. You can use the OpenNMT-py package in Python to build and train a model.
Here is the link to my github repository: https://github.com/N00rAhmed/python-translator