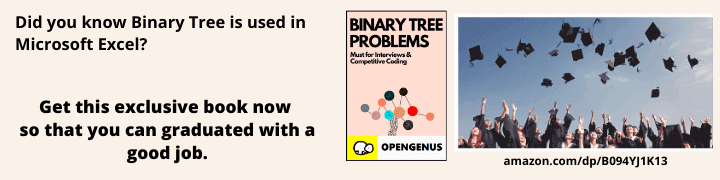
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will be discussing list and dictionary comprehension and it's relevance.
Table of Contents:
- Lists
- Dictionaries
- For loops
- Conditional statements
- List and Dictionary Comprehension
Lists
Lists are important data structures in Python used for containing elements. These elements can be in the form of numbers, letters and alphabets. Elements can be appended, removed and inserted into these lists. Each element within the list can be accessed using the index of that element. They are mainly used as storage devices for these elements so we can refer back to them later in the code.
Python example for implementing a list
fruits = ["Apples","Bananas","Grapes","Pears"] #creating a list
fruits.remove("Apples") #removes the first occurence of Apples
fruits.insert("Guava") #inserts the element guava into the list
fruits.append("Melon") #appends melon to the list
print(fruits)
Dictionaries
Dictionaries are also data structures in Python. Its pythons native implementation of a hash map. It stores key value pairs. Instead of using [], it uses {} to denote itself. Each value in the dictionary can be accessed using the key. New keys and values can also be inserted into a dictionary and you can change previous key values to something else.
Python example for implementing a dictionary
countries = {
"France": Paris,
"Germany":"Berlin",
"Russia":"Moscow",
"United Kingdom": "London"} #creating a dictionary
country = countries["France"] #getting the value associated with the key France
countries["France"] = "Stockholm" #changing the value of France
countries["Switzerland"] = "Bern" #adding a new country to the list with an associated value
countries.pop(France) #using the method pop to delete France from the dictionary
print(countries)
For Loops
For loops are used for repetitive tasks. Like going through a list or dictionary to find an element or key:value pair or even adding new values to a list. It's used for incrementation and can be used to save a lot of time when coding because we can avoid repetition. There is another loop in python known as the while loop. But for this article, I'll be using for loops for my examples
Python example for using a for loop
fruits = ["Apples","Bananas","Grapes","Pears"]
for i in fruits:
print (i) #prints every fruit in the list
In loops, you must indent the next statement after calling the loop.
Conditional Statements
Conditional statements are used to control the flow of your program. These are statements in your code used for making decisions. They consist of if, else and elif statements. They check the particular condition assigned to it and execute the function assigned to it.
Python example for using conditional statements
fruits = ["Apples","Bananas","Grapes","Pears"]
for i in fruits:
if i = "Apples":
print("Best fruits")
elif i = "Grapes":
print("Better than Apples")
else:
print("Wish I had Oranges")
For else statements, you don't assign a condition to it. Because it's a catch all conditional statement. Like loops, anything after the conditional statement should be indented.
List and Dictionary Comprehension
This brings us to list and dictionary comprehension. Using this, we can save lines of code and still execute the code. And it's easier to read. With list and dictionary comprehension, you can create new lists and dictionaries and add elements prior to them without having to implement a new dictionary and append.
Python example after using list comprehension
fruits = ["Apples","Bananas","Grapes","Pears"]
new_fruit = [i for i in fruits if "r" in i] #we only want fruits that have the letter r
print(new_fruit)