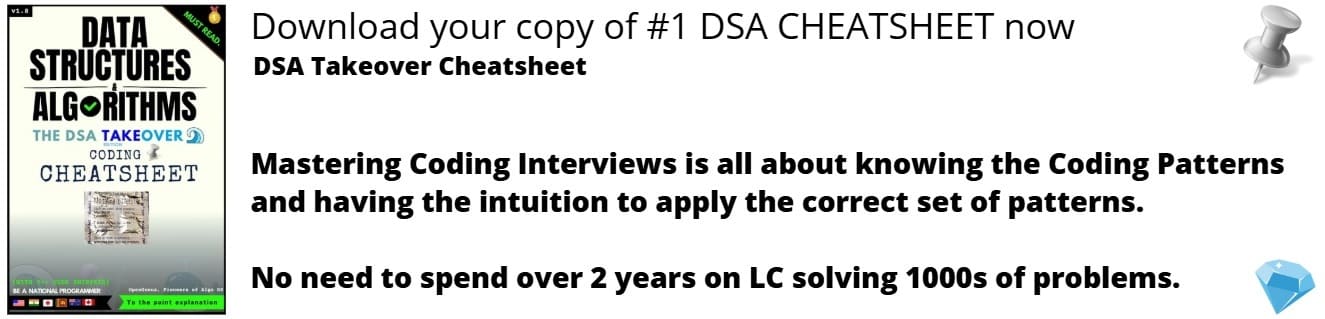
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes
Intel's Math Kernel Library (Intel MKL) is a Basic Linear Algebra Subprograms (BLAS) Library that optimizes code with minimal effort for future generations of Intel processors. It is compatible with your choice of compilers, languages, operating systems, and linking and threading models.
Intel's MKL is not open source.
Download MKL
Download the Intel's MKL library from Intel's download page
Usage
Include the header file mkl.h in your source code and link the MKL library while compiling and executing.
Example of matrix multiplication using MKL library
#include <mkl.h>
#include <stdio.h>
#include <sys/time.h>
#include <stdlib.h>
#include <math.h>
void init_matrix(double* A, int dim1 , int dim2 )
{
int mod = 100003, prod = 7 , e = 1 , i = 0, j = 0;
for ( i = 0; i < dim1; ++i )
{
for ( j = 0; j < dim2; ++j )
{
e = (e*prod + 1)%mod; // random
A[i*dim2 + j] = e * .91739210437;
}
}
}
int main(int argc, char** argv)
{
int m , n , k , i , j , u , nrep = 1 , cnt = 0;
double *A , *B , *C;
if ( argc != 4 )
{
puts("Format: ./a.out dimension_1 dimension_2 dimension_3");
exit(0);
}
if ( argc == 4 )
{
sscanf ( argv[1] , "%d" , &m );
sscanf ( argv[2] , "%d" , &k );
sscanf ( argv[3] , "%d" , &n );
}
A = (double *) malloc( sizeof(double) * m * k );
B = (double *) malloc( sizeof(double) * k * n );
C = (double *) malloc( sizeof(double) * m * n );
init_matrix ( A , m , k );
init_matrix ( B , k , n );
cblas_dgemm ( CblasRowMajor, CblasNoTrans, CblasNoTrans , m , n , k , 1.0 , A , k , B , n , 0.0 , C , n );
return 0;
}
Compile and execute the above code:
gcc matrix_multiplication_mkl.c
./a.out 100 100 100