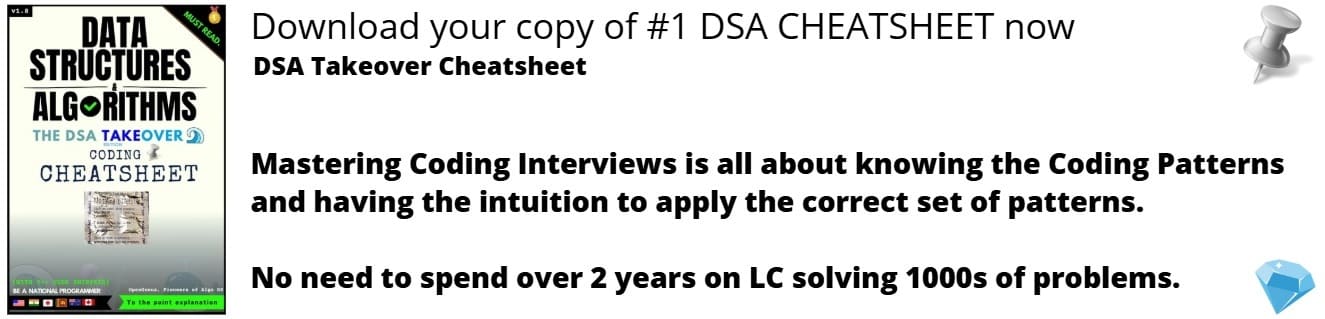
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes
OpenBLAS is an open source optimized BLAS (Basic Linear Algebra Subprograms) library based on GotoBLAS2 1.13 BSD version.
Install OpenBLAS from source
- Step 1: Clone the source of OpenBLAS
git clone https://github.com/xianyi/OpenBLAS.git
cd OpenBLAS
- Step 2: Build the source
make -j4
Usage
Statically link with libopenblas.a or dynamically link with -lopenblas if OpenBLAS was compiled as a shared library.
Example of Matrix Multiplication in OpenBLAS
#include <cblas.h>
#include <stdio.h>
#include <sys/time.h>
#include <stdlib.h>
#include <math.h>
void init_matrix(double* A, int dim1 , int dim2 )
{
int mod = 100003, prod = 7 , e = 1 , i = 0, j = 0;
for ( i = 0; i < dim1; ++i )
{
for ( j = 0; j < dim2; ++j )
{
e = (e*prod + 1)%mod; // random
A[i*dim2 + j] = e * .91739210437;
}
}
}
int main(int argc, char** argv)
{
int m , n , k , i , j , u , nrep = 1 , cnt = 0;
double *A , *B , *C;
if ( argc != 5 )
{
puts("Format: ./a.out number_of_iteration dimension_1
dimension_2 dimension_3");
exit(0);
}
if ( argc == 5 )
{
sscanf ( argv[1] , "%d" , &m );
sscanf ( argv[2] , "%d" , &k );
sscanf ( argv[3] , "%d" , &n );
}
A = (double *) malloc( sizeof(double) * m * k );
B = (double *) malloc( sizeof(double) * k * n );
C = (double *) malloc( sizeof(double) * m * n );
init_matrix ( A , m , k );
init_matrix ( B , k , n );
cblas_dgemm ( CblasRowMajor, CblasNoTrans, CblasNoTrans ,
m , n , k , 1.0 , A , k , B , n , 0.0 , C , n );
return 0;
}
Compile and run the above code:
gcc matrix_multiplication_openblas.c
./a.out 100 100 100