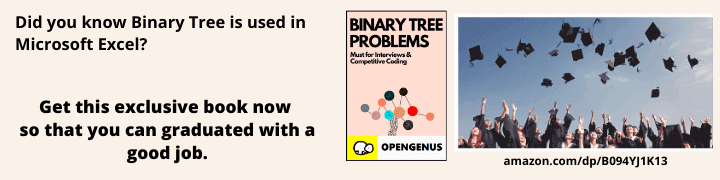
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained the difference between * and ** pointer in C and C++.
Table of contents:
1. What are Pointers?
2. Examples of Pointer
3. Implementation of Pointers using C
4. *p
vs **p
pointer
5. Implementation of *p
and **p
using C
What are Pointers?
Pointer is a variable used to store the address in memory of another variable.
The two operators used in the pointers:
- Operator & :- Gives the address of a variable
- Operator * :- Gives the values at location
Some Points:
- Address cannot be Negative.
- Pointer variable takes same bytes of memory irrespective of it’s data type.
- Pointer variable size differs from system to system depending on operating system.
- Pointer variable can dereference no. of bytes depending on it’s data type.
* Integer pointer can dereference two bytes of data.
* Character pointer can access one byte of data.
* Float pointer can access four bytes of data. - Pointer variable will be declared by using ‘*’(asterisk).
Examples of Pointer:
int * p ; // Declaration of pointer ‘p’
int a = 10 ;
p = &a ; //Assigning
//now pointer ‘p’ is pointing at variable ‘a’.
Here we are Declaring and Assigning memory block 'a' to a pointer 'p'.
- ‘p’ is the pointer variable of type integer where we can store address of any integer variable.
- ‘a’ is an integer variable storing value 10.
int * p ;
int a = 10 , b ;
b = &a ; //ERROR
Here we see an error because we are trying to store the address of an integer 'a' in an another integer 'b'
int a = 10 ;
printf(" a = %d " , * &a ); // output will be a = 10
Here we are printing value of 'a' by representing it as a pointer. Simply say, a=``*&a
.
int * p ;
int a = 10 ;
p = &a ;
printf(" %d " , a * p ) ; // ERROR
printf(" %d " , a * * p ); // No ERROR
Here we see an ERROR, because 'a' is having a value(=10) and *p
is also having a value(=10) but compiler will get confuse that what do we want to do because compiler see it as printf(" %d ", (a) (*p
) );, there is no arithmetic operators for compiler.
But in next printf() statement we don't see any ERROR because Now compiler will give output as 100 as we are doing Multiplication of 'a' and *p
and they both are containing value(=10) in them.
Implementation of Pointers using C
#include<stdio.h>
int main(){
int a=10;
int * p = &a ;
printf(" a = %d \n " , * p ) ; // It gives output a = 10
printf(" Address of a = %p \n ", p ) ; // It gives output Address of a
printf(" Address of p = %p \n ", & p ) ; // It gives output Address of p
return 0;
}
Here we printing the value of 'a' and address of 'a' and 'p'.
Output:
a = 10
Address of a = 000000000061FE1C
Address of p = 000000000061FE10
*p
vs **p
pointer
*p
is a pointer, means it holds an address of a value or a block of reserved memory, It can access upto one level.
It is used if you want to retain a block of memory where your values are in.
**p
is a pointer to a pointer, means it holds an address that holds an address of a value or a block of reserved memory, It can access upto two levels.
It is used if you want to pass a pointer that gets its memory allocated in a different function.
#include<stdio.h>
int main(){
int a=10;
int * p = & a ; // b is a single pointer or a pointer
int ** c = &p; // c is a double pointer or pointer to a pointer
printf("using p \n ");
printf(" value of a = %d \n " , * p ) ; // It gives output value of a = 10
printf(" Address of a = %p \n ", p ) ; // It gives output Address of a
printf(" Address of p = %p \n", & p ) ; // It gives output Address of p
printf("using c \n ");
printf(" value of a = %d \n ", ** c ) ; // It gives output value of a
printf(" Address of a = %p \n ", * c ) ; // It gives output Address of a
printf(" Address of c = %p \n ", &c ) ; // It gives output Address of c
printf(" Address of p = %p \n ", c ) ; // It gives output Address of p
return 0;
}
Here we are printing the values and addresses using a pointer and a double pointer.
Output:
using p
value of a = 10
Address of a = 000000000061FE1C
Address of p = 000000000061FE10
using c
value of a = 10
Address of a = 000000000061FE1C
Address of c = 000000000061FE08
Address of p = 000000000061FE10
Implementation of *p
and **p
using C
#include<stdio.h>
void swap(int * x ,int * y ){
int t = * x ;
* x = * y ;
* y = t ;
}
int main(){
int a = 10 , b = 20 ;
printf(" Value of 'a' before swap = %d \n", a );
printf(" Value of 'b' before swap = %d \n", b );
swap( & a , & b ) ; //function call
printf(" Value of 'a' after swap = %d \n", a );
printf(" Value of 'b' after swap = %d \n", b );
return 0;
}
Here we are Swapping two numbers using pointers.
While calling the function swap(), we are sending the addresses of memory blocks (i.e. a, b) and to take those addresses, we are taking integer pointers as the arguments in swap() (i.e. x, y).
In function swap(), we are swapping the values on the addresses.
Output:
Value of 'a' before swap = 10
Value of 'b' before swap = 20
Value of 'a' after swap = 20
Value of 'b' after swap = 10
#include<stdio.h>
void func(int ** p1 )
{
int b = 2;
* p1 = & b;
}
int main()
{
int a = 10;
int * p = &a;
printf("Before the function, value of the p : %d \n", * p) ;
func(&p); //function Call
printf("After the function, new value of the p : %d \n", * p) ;
return 0;
}
Here we first take an integer 'a' and assign value 10 to it,
then taking an integer pointer 'p' and assigning the address of 'a' to 'p'.
Now In function call func(), we are giving the address of 'p' which is a pointer, to take the address of a pointer, function func() should have an integer pointer to a pointer as an argument (i.e. p1 in func(p1) ).
In func() we are changing the value from 10 to 2.
Output:
Before the function, value of the p : 10
After the function, new value of the p : 2
With this article at OpenGenus, you must have the complete idea of * vs ** pointer in C.