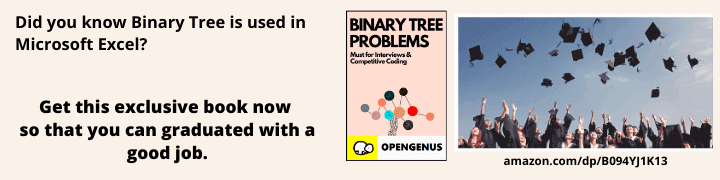
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 15 minutes | Coding time: 2 minutes
i++ is known as postfix increment operation while ++i is known as prefix increment operation. We compare these two operations based on:
- Use/ Program flow
- Compiler instruction
- Benchmark
We demonstrate that ++i is significantly faster than i++ in Java and should be kept into consideration.
Use/ Program flow
Consider the operation ++i:
- Step 1: Value of i is incremented by 1
- Step 2: Value of i is returned / used in the expression or statement
Consider the operation i++:
- Step 1: Value of i is returned / used in the expression or statement
- Step 2: Value of i is incremented by 1
Hence, in ++i, directly the value of i is changed while in i++, the value of i is copied into a temporary variable which is used in the expression and the value of i is incremented.
Note: i++ has an extra operation of copying an element i into another variable.
Demonstration using Java code:
class benchmark_opengenus
{
public static void main (String[] args)
{
int i = 1;
System.out.println(++i);
i = 1;
System.out.println(i++);
}
}
Output:
2
1
Compiler Instruction
Both i++ and ++i use iinc instruction
The exact command depends on the type of compiler being used.
Benchmark
To measure the performance of the two similar statements, we used the following Java code:
class benchmark_opengenus
{
public static void main (String[] args)
{
int i=0, limit = 1000000;
long t1_1 = System.nanoTime();
for(int j=0; j<=limit; j++)
{
i++;
}
long t1_2 = System.nanoTime();
long t2_1 = System.nanoTime();
for(int j=0; j<=limit; j++)
{
++i;
}
long t2_2 = System.nanoTime();
System.out.println("i++ took "+(t1_2-t1_1)+ " nanoseconds");
System.out.println("++i took "+(t2_2-t2_1)+ " nanoseconds");
System.out.println("++i took "+ String.format("%.2f",(double)(t2_2-t2_1)*100/(t1_2-t1_1))+" % of time taken by i++");
}
}
Output:
i++ took 7113590 nanoseconds
++i took 3814360 nanoseconds
++i took 53.62 % of time taken by i++
Hence, we see ++i is nearly 50% faster than i++.