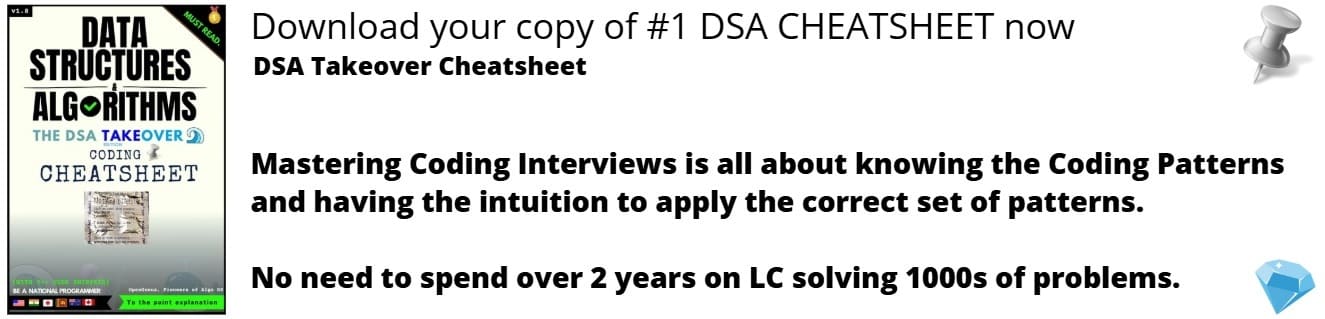
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will be discussing about Setprecision in C++ in detail along with C++ code examples.
Contents:
- Introduction to Setprecision
- Properties
- Syntax
- Parameter
- Return Value
- Program Code
- Explanation
- Comparision with other function
- Quiz
Introduction
The setprecision() method is present in the iomanip library.It allows the user to obtain a float or double data type's accurate value
With the help of this function, the user can get the precise value of a float or double data type.
The significant digit of N will be obtained if the N parameter is supplied to the setprecision() function without any data being lost.
Properties:
- It is an inbuilt function.
- Useful to prevent loss of data.
- easy to use
- Return requird value instead of long value.
Syntax
setprecision (int n);
Parameter:
This method accepts N numbers of arguments.
where N represents the required floating-point precision as a single integer argument.
Return Value
Unspecified.
Program
#include <iomanip>
#include <ios>
#include <iostream>
using namespace std;
int main()
{
double num = 5.147;
cout << "Original Number=\n"<< num << endl;
cout << "Number After Setprecision=\n"<< setprecision(3);
cout << num << endl;
}
output
Original Number=
5.147
Number After Setprecision=
5.15
Explanation:
Here, we are using the setprecision() function to set the precision to the original number, 5.147, and noting the value after performing precision.
Program 2
#include <iomanip>
#include <ios>
#include <iostream>
using namespace std;
int main()
{
float num = 2.716;
cout << "Original Number=\n"<< num << endl;
cout << "Number After Setprecision(1)=\n"<< setprecision(1);
cout << num << endl;
cout << "Number After Setprecision(2)=\n"<< setprecision(2);
cout << num << endl;
cout << "Number After Setprecision(3)=\n"<< setprecision(3);
cout << num << endl;
}
output
Original Number=2.716
Number After Setprecision(1)=3
Number After Setprecision(2)=2.7
Number After Setprecision(3)=2.72
Example
Here, we are using the setprecision() function to set the precision to the N (1, 2, 3) number to see the original number's precision value.
Program 3
#include <iomanip>
#include <ios>
#include <iostream>
using namespace std;
int main()
{
float num1 = 2.12;
float num2 = 2.17;
cout << "Original Number=\n"<< num1 << endl;
cout << "Original Number=\n"<< num2 << endl;
cout <<num1 << " After Setprecision=\n"<< setprecision(2);
cout << num1 << endl;
cout <<num2 << " After Setprecision=\n"<< setprecision(2);
cout << num2 << endl;
}
output
Original Number 1=2.12
Original Number 2=2.17
Number 1 After Setprecision=2.1
Number 2 After Setprecision=2.2
Explanation:
In the above example, we are using the setprecision() function on two different values to see how setprecision works.
Now,let us compare setprecision() with other function.
Floor Vs Setprecision
The floor function is a mathematical function that rounds a number down to the nearest integer.
For example, floor(4.6) would return 4, and floor(-2.8) would return -3.
On the other hand, setprecision() function is used in C++ programming language and it is used to set the precision of the floating point numbers.
It is used in conjunction with the fixed function and the scientific function.
For example, setprecision(3) will return only 3 digits after the decimal point.
In short, floor function is used to round down a decimal number to the nearest whole number, while setprecision is used to control the number of digits displayed after the decimal point in a floating-point value.
precision Vs Setprecision
In C++, "precision" and "setprecision" is that "precision" is a property of a floating-point number that refers to the number of digits in the number after the decimal point, while "setprecision" is a manipulator function that is used to control the number of digits displayed after the decimal point when outputting floating-point numbers.
The "precision" property of a floating-point number is determined by the type of the number (e.g. float, double, long double) and the specific implementation of the C++ compiler being used. For example, a float typically has a precision of 6-9 decimal digits, while a double typically has a precision of 15-17 decimal digits.
setw and setprecision
setw is a manipulator in C++ that sets the width of the output field for a stream. It determines the minimum number of characters to be written in the output field.
For example, if you use setw(8) and try to output a 5-character string, it will be padded with whitespace to fill the 8-character field.
setprecision is a manipulator that sets the number of decimal places to be written for floating-point values.
For example, if you use setprecision(2) and try to output the value 3.14159, it will be written as 3.14.
In summary, setw controls the width of the output field, while setprecision controls the precision of floating-point values in the output.
fixed and setprecision
fixed and setprecision are both manipulators in C++ that control the output formatting of floating-point values.
fixed is used to set the floating-point output format to fixed-point notation, which means that the decimal point is always present and the number of digits after the decimal point is fixed.
For example, if you use fixed and setprecision(2) to output the value 3.14159, it will be written as 3.14.
On the other hand, setprecision is used to set the number of decimal places to be written for floating-point values.
It works with both fixed and scientific formatting. For example, if you use setprecision(3) to output the value 123456, it will be written as 123456.000
In summary, fixed sets the floating-point output format to fixed-point notation, while setprecision sets the number of decimal places to be written for floating-point values. setprecision can be used with both fixed and scientific formatting.