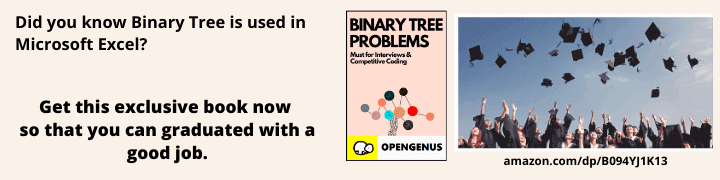
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we are going to build a random password generator in C Programming Language.
Define the Problem
Write a function in C that takes an integer as input and provide a string of certain length of pseudorandom characters as the output. The goal is to let the output meet some basic security requirement, which includes a certain length and the character set. To achieve this, we first want the output to be "random" and unique each time. we also want the function to have as many output as possible to generate so that the password will not be easily break by brute force.
Helpful Pre-requisites
- Final outputs would be string that includes lowercase and uppercase letters, numbers, and special characters.
- for loops to construct the output.
srand()
,rand()
, andtime()
function in C for the randomness.4.srand()
,rand()
, andtime()
function in C for the randomness.
Introduction to srand()
, rand()
and time()
function srand()
Input: int seed
- the integer value be used as seed for the pseudo-random number generator algorithm
Output: None
- initializes a random number generator that can be used later on to generate random numbers
function rand()
Input: None
Output: returns a pseudo-random number in the range of 0 to RAND_MAX
(a default value that depends on implementation)
If srand()
is not initialized and rand()
is called, the rand()
function will default the seed value to 1 which will generate the same "random" number every time.
function time()
Input: time_t *second
- set the time_t object which store the time
Output: returns current calendar time from 00:00:00 UTC, January 1, 1970 in seconds to input if provided. If the input is null, it will return the value like a normal function.
Solve the Problem
- Including the necessary headers -
stdio.h
,stdlib.h
, andtime.h
.stdio.h
andstdlib.h
are standard libraries that provide input/output and general purpose functions respectively, andtime.h
is used to seed the random number generator with the current time.
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
- Define two constants:
LENGTH
andCHARS
.LENGTH
is used to specify the desired length of the password, andCHARS
is a string of characters that will be used to generate the password.
#define LENGTH 8 // password length
#define CHARS "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789!@#$%^&*()_+-=[]{};':\"\\|,.<>/?`~" // characters to use in the password
- In the
main()
function, seed the random number generator with the current time using thesrand(time(NULL))
function. This ensures that the random number generated each time will be different each time the program runs.
int main() {
srand(time(NULL)); // seed the random number generator
- Declare an empty character array
password
with a length ofLENGTH + 1
. This array will be used to store the generated password. Since we want the password to beLENGTH
long, we initialize the array to beLENGTH + 1
to account for the null terminator in the end we need to add.
char password[LENGTH + 1]; // password array
- A for loop is used to generate the password. The loop iterates
LENGTH
times, and in each iteration, therand()
function is used to generate a random number within the length of theCHARS
string.
In order to limit the range of the random number, we use the %
operator. Since the value of the modulo operator is the remainder when the left-hand operand is divided by the right-hand operand, it will be guaranteed to be smaller than the right-hand operand, which in this case sizeof(CHARS) - 1
. Since arrays are 0-indexed, we want it to be sizeof(CHARS) - 1
instead of sizeof(CHARS)
.
The character at the position specified by this number is then selected as the next character in the password.
// loop to generate the password
for (int i = 0; i < LENGTH; i++) {
password[i] = CHARS[rand() % (sizeof(CHARS) - 1)]; // select a random character from the character set
}
- After the for loop completes, the password is terminated by setting the last character to
\0
. This is an important step because it indicates the end of the string, allowing the program to know where the password ends.
password[LENGTH] = '\0'; // null-terminate the string
- The password is then printed to the console using the
printf()
function, formatting it as a string.
printf("Password: %s\n", password); // print the password
return 0;
}
The full code is attached below.
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define LENGTH 8 // password length
#define CHARS "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789!@#$%^&*()_+-=[]{};':\"\\|,.<>/?`~" // characters to use in the password
int main() {
srand(time(NULL)); // seed the random number generator
char password[LENGTH + 1]; // password array
// loop to generate the password
for (int i = 0; i < LENGTH; i++) {
password[i] = CHARS[rand() % (sizeof(CHARS) - 1)]; // select a random character from the character set
}
password[LENGTH] = '\0'; // null-terminate the string
printf("Password: %s\n", password); // print the password
return 0;
}
Time and Space Complexity
Time Complexity: The for loop runs LENGTH
times. rand()
is constant time, and therefore each iteration of for loop is constant time. Rest of the operations outside of for loop are all constant, and therefore the runtime will depend on the LENGTH
of the password, thus O(LENGTH
).
Space Complexity: We defined password
string to print the output, and size of password
depends on LENGTH
. Therefore, the space complexity is O(LENGTH
).
Further Improvements
There are further improvements to this program that makes it more complex and close to real life. For example, we can prompt the user to input the length of the password they want. We can also take in 2 numbers that specify the range of the password to make it more random.
It's important to note that while the rand()
function is a quick and simple way to generate random numbers, it is not a cryptographically secure random number generator since it is only pseudorandom. Therefore, it should not be used for real-life cryptographic applications such as password generation. Instead, it is recommended to use a cryptographically secure random number generator, such as the ones provided by OpenSSL or libsodium, for security purposes.