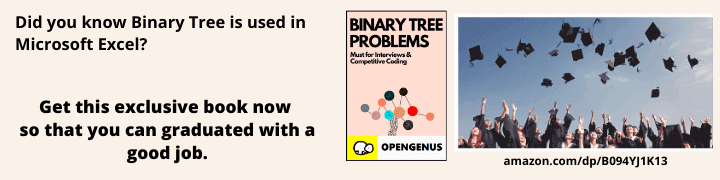
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 15 minutes | coding time: 2 minutes
Inheritance is the process of inheriting properties of objects of one class by objects of another class. The class which inherits the properties of another class is called Derived or Child or Sub class and the class whose properties are inherited is called Base or Parent or Super class.
Single Level Inheritance or Single Inheritance is the mechanism of deriving a class from only one single base class.
It is the simplest of all inheritance.
For example:
1.Animal is derived from living things
2.Car is derived from vehicle
- In the above diagram, Class B is derived from Class A. Class A is base class and Class B is a derived class.
Single Inheritance
Syntax
class base_classname
{
datatype variable name;
methods;
};
class derived_classname : visibility_mode base_classname
{
datatype variable_name;
methods;
};
Ambiguity in Single Inheritance in C++
If parent and child classes have same named method, parent name and scope resolution operator (::) is used. This is done to distinguish the method of child and parent class since both have same name.
Example
class staff
{
private:
char name[50];
int code;
public:
void getdata();
void display();
};
class typist: public staff
{
private:
int speed;
public:
void getdata();
void display();
};
void staff::getdata()
{
cout<<"Name:";
gets(name);
cout<<"Code:";
cin>>code;
}
void staff::display()
{
cout<<"Name:"<<name<<endl;
cout<<"Code:"<<code<<endl;
}
void typist::getdata()
{
cout<<"Speed:";
cin>>speed;
}
void typist::display()
{
cout<<"Speed:"<<speed<<endl;
}
int main()
{
typist t;
cout<<"Enter data"<<endl;
t.staff::getdata();
t.getdata();
cout<<endl<<"Display data"<<endl;
t.staff::display();
t.display();
getch();
return 0;
}
Output
Enter data
Name:Roger Taylor
Code:13
Speed:46
Display data
Name:Roger Taylor
Code:13
Speed:46
Note: In this example, typist class is derived and staff class is the base class. Public members of class staff such as staff::getdata() and staff::display() are inherited to class typist. Since the child is derived from a single parent class, it is single inheritance.