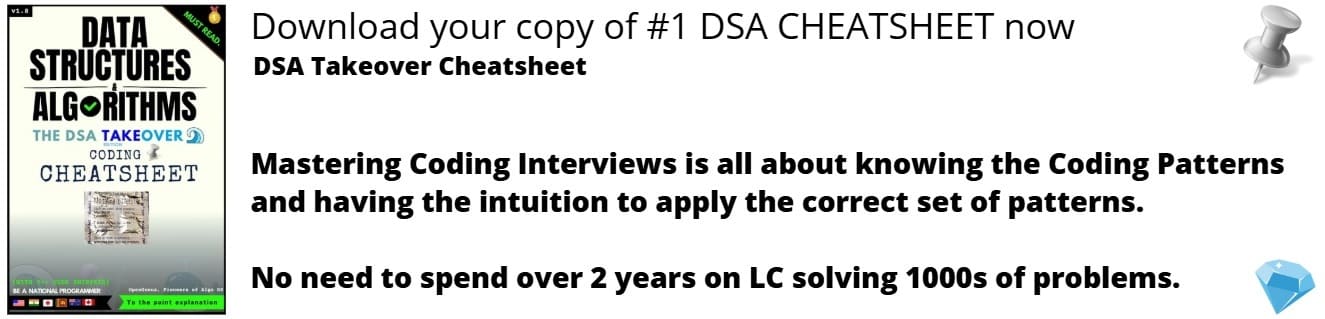
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will learn how to get size of 2D vector in C++. This topic has a wide applications such as in graphs, or even in machine learning. Getting used to handling 2D vectors in C++ helps us approach our problems easier.
Table of contents:
- Size of 1D vector in C++
- Size of 2D vector MxN in C++
- What if our 2D vector has different column size?
Prerequisite: 2D vector in C++
Let us get started with Size of 2D vector in C++. We have also covered how to get total number of elements in 2D vector in C++.
Size of 1D vector in C++
Let's get started with a 1D vector. Imagine a 1D vector is a single row in excel or google sheets, that is a table with only one row, and in that one row you have a number of elements, for example:
5 | 6 | 3 | 5 |
The table above has 1 row and 4 columns (or elements). To generalize it, let's call m is the number of rows and n is the number of columns. In this case m is 1, n is 4.
There are many ways to define a 1D vector, however for simplicity, we will define it like below:
vector<int> vect{5, 6, 3, 5};
To get the size of the vector all we need to do is to use .size()
function:
vector<int> vect{5, 6, 3, 5};
int n;
n = vect.size(); // n = 4
Size of 2D vector MxM in C++
Similarly for 2D vector, imagine a 2 dimensional table just like in google sheets, or excel! That is how our 2D vector should looks like:
A 2D vector has m rows and n columns. For example:
5 | 6 | 3 |
1 | 2 | 4 |
The above table has m = 2 rows and n = 3 columns.
We can define a 2D Vector just like in this link.
To speed up the process let's say we have a vector vect
and we are going to define it like this:
vector<vector<int>> vect{{ 5, 6, 3 }, { 1, 2, 4 }};
And this is how the table above is represented in a 2D vector. Thinking it like a large vector is containing two other small vectors inside. The outer vector has the length of 2, and the two smaller vectors have the size of 3 each.
To get the size of the vector all we need to do is the following:
Let's say we have 2 variables m and n, and we are going to store the size of the vector into it.
Example:
#include <iostream>
#include <vector>
using namespace std;
int main() {
int m, n;
vector<vector<int>> vect{{ 5, 6, 3 }, { 1, 2, 4 }};
m = vect.size(); // size() function is use to return the size of the vector
n = vect[0].size();
cout << "The dimension of the vector is " << m << " x " << n << endl;
// Now m and n will have the values m = 2, n = 3
cout << "The vector has " << m*n << " elements";
}
Output
The dimension of the vector is 2 x 3
The vector has 6 elements
Since our vector has the same number of columns, therefore we only need to access one of the row to get the size of it. To get the total number of elements in this vector all we need to do is to take m * n, simple right?
What if our 2D vector has different column size?
Like row 0 has 4 elements, and row 1 has 5 elements? The code below will generalize it. The idea is to store the number of elements of a row in an array or a vector, so we can easily get the number of elements of our desire row. To get the total number of elements in this vector, we need to get the sum of the size of all small vectors inside the large vector. The code below will better explain!
Example
#include <iostream>
#include <vector>
using namespace std;
int main(){
vector<vector<int>> vect{{ 5, 6, 3, 3 }, { 1, 2, 4, 10, 2 }};
// here our row 0 has 4 elements of 5, 6, 3, 3
// row 1 has 5 elements of 1, 2, 4, 10, 2
int totalElements = 0; // total number of elements
int m = vector.size(); // number of rows
vector<int> numElements(m, 0); // vector of m rows
for(int i = 0; i < m; i++){
numElements[i] = vect[i].size();
totalElements += vect[i].size();
}
for(int i = 0; i < m; i++) {
cout << "Row " << i << " has " << numElements[i] << " elements\n";
}
cout << "The vector has " << totalElements << " elements";
}
// at the end we will have numElments of all rows
// the total element is stored in totalElements
Output
Row 0 has 4 elements
Row 1 has 5 elements
The vector has 9 elements
And that is it with this article at OpenGenus! Thanks for reading!