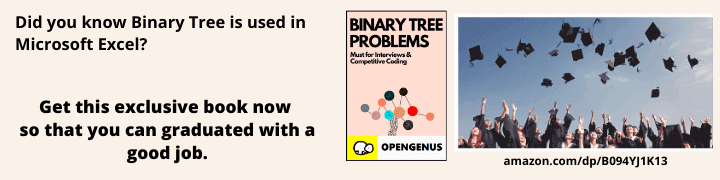
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 5 minutes
With Standard template library available in C++, many functions are easier to implement. One of them present is sort function as well which we are going to discuss.
The main aim of this function is to sort any vector given by specifying certain parameters like comparison order.
Syntax of using sort()
Before
sort(begining index, end index) // ascending order
// OR
sort(begining index, end index, greater<int>()) // descending order
NOTE : By default, sort() sorts an array in ascending order.
We can define our own comparison function comp() and use it as well like:
sort(begining index, end index, comp()) // custom order
Example 1: Sorting integer in ascending order
The program below helps us to undestand the passing of parameters of given array to the pre-defined sort functaion for having the result in ascending order.
// C++ program to demonstrate default behaviour of
// sort() in STL.
//Ascending Order
#include <bits/stdc++.h>
using namespace std;
int main()
{
int arr[] = {100,90,80,70,60,50,40,30,20,10};
int n = sizeof(arr)/sizeof(arr[0]);
sort(arr, arr+n); //arr = 0 arr+n=9
cout << "\nArray after sorting using "
"default sort is : \n";
for (int i = 0; i < n; ++i)
cout << arr[i] << " ";
return 0;
}
Output :
Array after sorting using default sort is :
10 20 30 40 50 60 70 80 90 100
Example 2: Sorting integer in descending order
The code helps one to understand the greater<>() which helps us to get the result in descending order.
// C++ program to demonstrate descending order sort using
// greater<>().
//Descending order
#include <bits/stdc++.h>
using namespace std;
int main()
{
int arr[] = {10 ,20 ,30 ,40 ,50 ,60 ,70 ,80 ,90 ,100 };
int n = sizeof(arr)/sizeof(arr[0]);
sort(arr, arr+n, greater<int>());
cout << "\nArray after sorting using "
"default sort is : \n";
for (int i = 0; i < n; ++i)
cout << arr[i] << " ";
return 0;
}
greater() function is used to sort in descending order. This function does a comparison in a way that puts greater element before.
Output :
Array after sorting using default sort is :
100 90 80 70 60 50 40 30 20 10
How to sort in particular order?
We can also write our own comparator function and pass it as a third parameter.
This comparator function that returns a value which is convertible to bool, which indicates whether the passed first argument should be placed before the passed second argument or not.
In the code below, suppose intervals {8,10} and {4,12} are passed as arguments in the compareInterval function(comparator function). As i1.first (=8) > i2.first (=4), so our function returns false, which tells us that first argument should not be placed before second argument and so sorting will be done in order like {4,12} first and then {8,10} as next.
Example :
// A C++ program to demonstrate STL sort() using
// our own comparator
#include<bits/stdc++.h>
using namespace std;
// An interval has a start time and end time
class Interval
{
public :
int start, end;
};
// Compares two intervals according to staring times.
bool compareInterval(Interval i1, Interval i2)
{
return (i1.start < i2.start);
}
int main()
{
Interval arr[] = { {8,10}, {4,12}, {5,7}, {6,9} };
int n = sizeof(arr)/sizeof(arr[0]);
// sort the intervals in increasing order of
// start time
sort(arr, arr+n, compareInterval);
cout << "Intervals sorted by start time : \n";
for (int i=0; i<n; i++)
cout << "[" << arr[i].start << "," << arr[i].end
<< "] ";
return 0;
}
Output :
Intervals sorted by start time :
[4,12] [5,7] [6,9] [8,10]
Example 3
This example shows sorting of a string by passing the begining and ending of the provided string.
// C++ program to sort a string of characters
#include<bits/stdc++.h>
using namespace std;
// function to print string in sorted order
void sortString(string &str)
{
sort(str.begin(), str.end());
cout << str;
}
// Driver program to test above function
int main()
{
string s = "OpenGenus IQ";
sortString(s);
return 0;
}
Output :
GIOQeennpsu
Example 4
This examples is a combination of user defined function (class and operator overloading) and the comparator which was explained earlier in this article.
We have 2 classes Player and PlayerComparator which have 2 operators overloaded "< & ()".
#include <list>
#include <string>
#include <algorithm>
#include <iostream>
class Player
{
public :
int id;
string name;
Player(int playerId, string playerName) :
id(playerId), name(playerName)
{
}
bool operator <(const Player & playerObj) const
{
return id < playerObj.id;
}
};
class PlayerComparator
{
public :
// Compare 2 Player objects using name
bool operator ()(const Player & player1, const Player & player2)
{
if(player1.name == player2.name)
return player1 < player2;
return player1.name < player2.name;
}
};
int main()
{
list<Player> listofPlayers = { Player(20, "Ram"),
Player(13, "Kriti"),
Player(34, "Priya"),
Player(3, "Aman"),
Player(45, "Sakshi"),};
cout<<"****Playes in the list Initially ****"<<std::endl;
for(Player & player : listofPlayers)
cout<<player.id<< " :: "<<player.name<<std::endl;
// Sort List by default criteria i.e < operator of Player
listofPlayers.sort();
cout<<"****After Sorting By ID ****"<<std::endl;
for(Player & player : listofPlayers)
cout<<player.id<< " :: "<<player.name<<std::endl;
cout<<"****After Sorting By Name ****"<<std::endl;
// Sorting List using Lambda function as comparator
listofPlayers.sort([](const Player & player1, const Player & player2)
{
if(player1.name == player2.name)
return player1 < player2;
return player1.name < player2.name;
});
for(Player & player : listofPlayers)
cout<<player.id<< " :: "<<player.name<<std::endl;
cout<<"****After Sorting By Name ****"<<std::endl;
// Sorting List using Function Objects as comparator
listofPlayers.sort(PlayerComparator());
for(Player & player : listofPlayers)
cout<<player.id<< " :: "<<player.name<<std::endl;
return 0;
}
Output :
****Playes in the list Initially ****
20 :: Ram
13 :: Kriti
34 :: Priya
3 :: Aman
45 :: Sakshi
****After Sorting By ID ****
3 :: Aman
13 :: Kriti
20 :: Ram
34 :: Priya
45 :: Sakshi
****After Sorting By Name ****
3 :: Aman
13 :: Kriti
34 :: Priya
20 :: Ram
45 :: Sakshi
****After Sorting By Name ****
3 :: Aman
13 :: Kriti
34 :: Priya
20 :: Ram
45 :: Sakshi