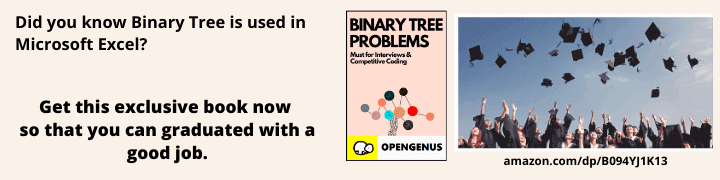
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
We will discuss the Stopwatch Console Application in Java today. In this application, input can be taken at the same time as output is printed on the console using multithreading. Some of its basic features include stopping, pausing, restarting, and quitting.
We'll discuss:
- Introduction
- Features of application
- Multithreading
- How the Stopwatch Application works?
- Code
- What are volatile variables?
- Example
- Conclusion
Introduction
The Stopwatch Console Application is a program that helps in measuring the elapsed time in a particular task. The program is developed in Java language, and it is a console-based application. It provides features like stopping the timer, recording intermediate laps and resetting the timer.
It is useful for a wide range of applications like timing athletic events, measuring cooking time, and much more. The Stopwatch Console Application provides a simple, accurate, and easy-to-use method of measuring time.
Features of application
Features of Stopwatch Console Application in Java
-
User input: The code takes user input through the Scanner class to determine whether to
start
,pause
,resume
, orstop
the stopwatch. -
Pause/resume: The code allows the user to pause and resume the stopwatch by setting the paused flag accordingly. The code also calculates the pause duration and subtracts it from the elapsed time to accurately display the elapsed time when the stopwatch is resumed.
-
Multithreaded operation: The code runs the stopwatch on a separate thread, using the Thread class. This allows the stopwatch to continue running in the background, even while the user is entering input to pause, resume, or stop the stopwatch.
-
Timer formatting: The elapsed time is formatted as a string and displayed to the console in the format
hh:mm:ss:ms
. -
Error handling: The code includes error handling, in the form of try-catch blocks, to handle any exceptions that may occur while printing the elapsed time to the console or sleeping the stopwatch thread.
Multithreading
Multithreading in Java is a feature that allows multiple threads of execution to run concurrently within the same program. It enables the creation of lightweight and independent tasks, which can run parallel to the main thread and enhance the performance of the program.
A thread is an independent path of execution within a program, and a program can contain multiple threads that run concurrently. In Java, each thread has its own stack and runs in parallel with other threads in the program.
The java.lang.Thread
class provides several methods to create and control threads, such as start()
, run()
, join()
, and sleep()
. The Runnable interface is also used to define the task that the thread will execute.
Multithreading is useful in various applications, including improving the performance of CPU-bound tasks, providing a responsive user interface, and managing the execution of multiple tasks simultaneously.
If you want to read about Multithreading in detail, visit the article.
How the Stopwatch Application works?
The stopwatch application in this code works by using the System.currentTimeMillis()
method to get the current time in milliseconds, and then calculating the elapsed time by subtracting the start time from the current time. The elapsed time is calculated and displayed on the console using a separate thread, which runs until the running flag is set to false.
The application prompts the user to either start the stopwatch (by pressing "S") or quit the program (by pressing "Q"). If the user presses "S", the startStopwatch()
method is called and a new thread is created to run the stopwatch. The thread runs a loop that continuously calculates the elapsed time and formats it as a string, which is then printed to the console.
While the stopwatch is running, the user can pause (by pressing "P"), resume (by pressing "R"), or stop (by pressing "Enter") the stopwatch. If the user presses "P", the paused flag is set to true and the pause duration is calculated and stored. If the user presses "R", the paused flag is set to false and the pause duration is updated. If the user presses "Enter", the running flag is set to false, causing the stopwatch thread to exit, and the stopwatch is stopped.
Code
// import the scanner class for the user input
import java.util.Scanner;
public class Main {
// create a scanner object for user input
static Scanner sc = new Scanner(System.in);
// running flag to control the stopwatch loop
static volatile boolean running = true;
// paused flag to pause/resume the stopwatch
static volatile boolean paused = false;
// string to store userInput
static String userInput;
public static void main(String[] args) {
// prompt the user to start or quit the program
System.out.println("Press 'S' to start, 'Q' to quit");
// read the user input
userInput = sc.nextLine();
// check if the user eants to start or quit the program
if (userInput.equalsIgnoreCase("S")) {
startStopwatch();
} else if (userInput.equalsIgnoreCase("Q")) {
System.out.println("\nExiting program");
} else {
System.out.println("Invalid input. Press 'S' to start, 'Q' to quit");
}
}
private static void startStopwatch() {
// code is wriiten below for this method
}
}
This code is a simple implementation of a stopwatch console application in Java. The code starts by creating a scanner object, sc
, to read the user input. It then declares two flags, running and paused, and a userInput string to store the user input.
In the main method, the code prompts the user to either start the stopwatch by pressing "S" or quit the program by pressing "Q". The userInput string is then used to store the user's input.
The code then checks if the user wants to start the stopwatch by comparing the user input to "S". If the user inputs "S", the startStopwatch method is called. If the user inputs "Q", a message is displayed saying the program is exiting. If the user inputs anything else, an error message is displayed, reminding the user to press "S" to start or "Q" to quit.
private static void startStopwatch() {
// get the current time in milliseconds
final long startTime = System.currentTimeMillis();
// array to store the elapsed time
final long[] elapsedTime = {0};
// array to store the pause duration
final long[] pauseDuration = {0};
// create a new thread for the stopwatch
Thread stopwatchThread = new Thread(() -> {
// loop until running is set to false
while (running) {
// check if the stopwatch is not paused
if (!paused) {
// calculate the elapsed time
elapsedTime[0] = System.currentTimeMillis() - startTime - pauseDuration[0];
}
// format hte elapsed time as a string
String timer = String.format("\rElapsed time: %02d:%02d:%02d:%03d ", elapsedTime[0] / 3600000, (elapsedTime[0] % 3600000) / 60000, (elapsedTime[0] % 60000) / 1000, elapsedTime[0] % 1000);
try {
// print the elapsed time to the console
System.out.write(timer.getBytes());
} catch (Exception e) {
// print the stack trace if an error occurs
e.printStackTrace();
}
try {
// sleep for 1 millisecond
Thread.sleep(1);
} catch (InterruptedException e) {
// print the stack trace if an error occurs
e.printStackTrace();
}
}
});
// start the stopwatch thread
stopwatchThread.start();
// prompt the user to pause, resume, or stop the stopwatch
System.out.println("Press 'P' to pause, 'R' to resume, 'Enter' to stop");
// loop until running is set to false
while (running) {
// read the user input
userInput = sc.nextLine();
// check if the user wants to pause the stopwatch
if (userInput.equalsIgnoreCase("P")) {
paused = true;
pauseDuration[0] = System.currentTimeMillis() - startTime - elapsedTime[0];
System.out.println("\nPaused");
// check if the user wants to resume the stopwatch
} else if (userInput.equalsIgnoreCase("R")) {
paused = false;
pauseDuration[0] = System.currentTimeMillis() - startTime - elapsedTime[0];
System.out.println("\nResumed");
// check if the user wants to stop the stopwatch
} else {
running = false;
System.out.println("\nStopped");
}
}
}
The startStopwatch method is the core of the stopwatch application. It is responsible for measuring and displaying the elapsed time.
The method starts by capturing the current time in milliseconds using System.currentTimeMillis()
and storing it in the startTime
variable. Then, it declares two arrays elapsedTime
and pauseDuration
, both of length 1, to store the elapsed time and pause duration, respectively.
Next, a new thread stopwatchThread
is created using a lambda expression. The lambda expression implements the run
method of the Runnable
interface. This thread is responsible for continuously measuring the elapsed time and printing it to the console.
The stopwatchThread
uses a while loop that runs until the running
flag is set to false. Within the loop, the elapsedTime
is calculated by subtracting the startTime
from the current time in milliseconds and subtracting the pauseDuration
.
The elapsed time is then formatted as a string in the format of hh:mm:ss:SSS
using the String.format
method and stored in the timer
variable. This string is printed to the console using System.out.write(timer.getBytes())
. After printing the elapsed time, the thread sleeps for 1 millisecond using Thread.sleep(1)
.
Finally, the stopwatchThread
is started using the start
method. The program then prompts the user to either pause ('P'), resume ('R'), or stop the stopwatch. The user input is read and processed in a separate while loop that runs until the running
flag is set to false.
If the user inputs 'P', the paused
flag is set to true, and the pauseDuration
is updated to reflect the current pause. If the user inputs 'R', the paused
flag is set to false, and the pauseDuration
is updated to reflect the current pause. If the user inputs anything else or Enter
, the running
flag is set to false, and the stopwatch is stopped.
Note: The reason why the variables elapsedTime
and pausedDuration
need to be final
or effectively final
is because they are being used within an anonymous inner class or a lambda expression in this code. In Java, variables that are used within anonymous inner classes or lambda expressions must be either final or effectively final.
What are volatile variables?
A volatile variable is used to indicate that a variable's value will be modified by different threads. When a field is declared volatile, the Java memory model ensures that all threads see a consistent value for the variable, even if it is modified by another thread. This is useful when writing multi-threaded programs, as it ensures that changes to a volatile variable are visible to all threads, preventing issues with thread synchronization.
By using volatile with the elapsedTime
and pausedDuration
variables, the program ensures that all threads see a consistent value for these variables even if they are being modified by another thread. This helps to prevent issues with thread synchronization and ensures that all threads have access to the correct values.
Example
Take a look at the code's result,
Conclusion
In conclusion, the stopwatch program provides a simple and efficient solution for measuring elapsed time. The program uses Multi-Threading concept to print the output in the console at the same time when it take input from the user.