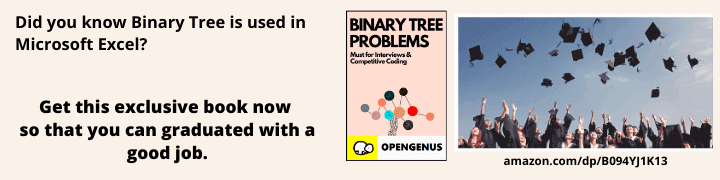
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes
A switch statement allows a variable to be tested for equality against a list of values. Each value is called a case, and the variable being switched on is checked for each case.
These statements are a substitute for long if statements that compare a variable to several integral values.
Note : For menu driven program the are used inside do...while loop.
Syntax :
switch (n)
{
case 1: // code to be executed if n = 1;
break;
case 2: // code to be executed if n = 2;
break;
default: // code to be executed if n doesn't match any cases
}
Important points about switch case statement
1)The expression provided in the switch should result in a constant value otherwise it would not be valid.
Valid expressions for switch:
// Constant expressions allowed
switch(1+2+23)
switch(1*2+3%4)
Invalid switch expressions for switch:
// Variable expression not allowed
switch(ab+cd)
switch(a+b+c)
- Duplicate case values are not allowed.
Valid expressions for switch:
switch(n)
{
case 1 : //some statemnets
break;
case 2 : //some statements
break;
default : //some statements
}
Inalid expressions for switch:
switch(n)
{
case 1 : //some statemnets
break;
case 1 : //some statements
break;
default : //some statements
}
- The default statement is optional.Even if the switch case statement do not have a default statement, it would run without any problem.
Valid expressions for switch:
//With default
switch(n)
{
case 1 : //some statemnets
break;
case 2 : //some statements
break;
default : //some statements
}
Valid expressions for switch:
//Without default
switch(n)
{
case 1 : //some statemnets
break;
case 2 : //some statements
break;
}
- The break statement is used inside the switch to terminate a statement sequence. When a break statement is reached, the switch terminates, and the flow of control jumps to the next line following the switch statement.
The break statement is optional. If omitted, execution will continue on into the next case. The flow of control will fall through to subsequent cases until a break is reached.
With break and
//With break
//Without default
switch(n)
{
case 1 : cout<<"1 \n";
break;
case 2 : cout<<"2 \n";
break;
}
Output :
//n=1
1
//n=2
2
Without break and default
// Without break
//Without default
switch(n)
{
case 1 : cout<<"1 \n";
case 2 : cout<<"2 \n";
}
Output :
//n=1
1
2
//n=2
2
NOTE : There are many more cases regarding the above mentioned step please do try it yourself for better undersatnding of switch case.
- Nesting of switch statements are allowed, which means you can have switch statements inside another switch. However nested switch statements should be avoided as it makes program more complex and less readable.
Example
// Following is a simple program to demonstrate
// syntax of switch.
#include <iostream>
using namespace std;
int main()
{
int x = 1;
switch (x)
{
case 1: cout<<"Choice is 1 ";
break;
case 2: cout<<"Choice is 2";
break;
case 3: cout<<"Choice is 3";
break;
default: cout<<"Choice other than 1, 2 and 3";
break;
}
return 0;
}
Output :
Choice is 1
Question
Consider the following C++ code:
int main()
{
int choice = -2 ;
switch(choice)
{
case -1:
printf("\nCase -1");
break;
case -2:
printf("\nCase -2");
break;
}
return 0;
}