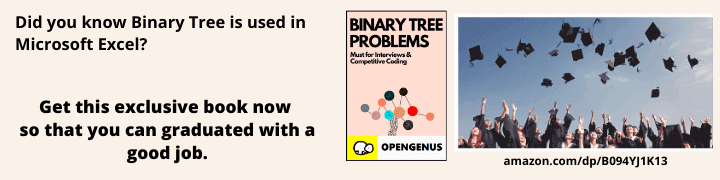
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
This article explains the basics of type conversion in Java, as well as potential problems to be aware of.
Table of contents:
- Typecasting
- Implicit typecasting
- Explicit typecasting
- Type conversion with methods
- Type conversion to String
- Type conversion from String
Typecasting
In Java, data type conversion on the language level is called typecasting, it can be done manually by adding (name-of-type) in parenthesis before the expression. For example, converting integer data type to the double data type:
public class Typecasting {
public static void main (String[] args) {
int integerNumber = 10;
double doubleNumber = (double) integerNumber;
System.out.println(integerNumber); // Output: 10
System.out.println(doubleNumber); // Output: 10.0
}
}
Implicit typecasting
With primitive data types, Java automates the conversion in the direction of bigger size, for example, integer to double, this is called implicit typecasting.
public class Typecasting {
public static void main (String[] args) {
int integerNumber = 10;
// Automatic type conversion on variable initialization
double doubleNumber = integerNumber;
// Automatic type conversion on passing arguments to method
double square = squareArea(integerNumber);
System.out.println(doubleNumber); // Output: 10.0
System.out.println(square); // Output: 100.0
}
// Method supposed to accept single argument of type double
static double squareArea (double side) {
return side * side;
}
}
For numeric primitive data types automatic conversion (widening) runs without data losses as follows:
byte -> short -> int -> float -> long -> double
public class Typecasting {
public static void main (String[] args) {
byte number_byte = 127;
// Convert byte to short
short number_short = number_byte;
// Convert short to int
int number_int = number_short;
// Convert int to float
float number_float = number_int;
// Convert float to double
double number_double = number_float;
// Convert byte to double
double fromByteToDouble = number_byte;
System.out.print(""+ number_byte + '\n' + // Output: 127
number_short + '\n' + // 127
number_int + '\n' + // 127
number_float + '\n' + // 127.0
number_double + '\n' + // 127.0
fromByteToDouble + '\n'); // 127.0
}
}
Converting from int to float (32 bits to 32 bits), and from long to double (64 bits to 64 bits) can result in data losses despite the actual size being the same. This is because float and double data types are stored as floating-point numbers and need extra space to store the exponent part.
In cases when data type conversion is not defined within the Java language or can result in data losses the compiler reports the type mismatch error:
public class Typecasting {
public static void main (String[] args) {
// Converting double to int
double doubleNumber = 100;
int integerNumber = doubleNumber; // type mismatch error
// double uses 64 bits
// and int with 32 bits available has not enough place
// Converting boolean to byte
boolean booleanValue = true; // type mismatch error
byte byteValue = value; // Java language does not define boolean to byte conversion
// so compiler prohibits cast
}
}
Explicit typecasting
Explicit typecasting - with (name-of-datatype) in parenthesis makes the compiler ignore type mismatch error and convert data types in any direction, including narrowing direction.
With the numeric data types compiler ignores bits that do not fit into the final data type. In some cases, such conversion does not lose any data, such as converting from double 15.0 to integer 15, but in other cases, data can be lost.
public class Typecasting {
public static void main (String[] args) {
// Double without fractional part to integer
double doubleNumber = 15;
int integerNumber = (int) doubleNumber;
System.out.println(integerNumber); // 15 - no information loss
// Double with fractional part to integer
doubleNumber = 13.93451234;
integerNumber = (int) doubleNumber;
System.out.println(integerNumber); // 13 - decimal part is truncated
// Integer to char
int letterNumber = 65;
char letter = (char) letterNumber;
System.out.println(letterNumber); // 65
System.out.println(letter); // A
}
}
As the code above shows, typecasting from numeric to alphabetical primitive data types works as well - this is because all characters use numerical representation and standard conversion tables. The ASCII table, for example, decodes the letter A with the number 65.
Type conversion with methods
As the cast is a language-level operation, any cast operation not defined or prohibited at the language level results in a compiler error. As in the code examples above, Java does not support type casting from boolean to other primitive types, although boolean primitive takes only one bit of memory space and may fit into other types easily.
One should not simply give up though, as the good news is that there is no restriction on type conversion through user-defined methods. For example, convert boolean to numeric representation:
public class Typecasting {
public static void main (String[] args) {
// Boolean to byte
boolean bool = true;
byte number = bool; // type mismatch error
byte number = boolToByte(bool);
System.out.println(number); // Output: 1
}
// Output 1 if true and 0 if false
static byte boolToByte (Boolean value) {
return value ? (byte) 1 : (byte) 0;
}
}
As you have probably guessed, such a mature language as Java already has methods for type conversions that are not supported by typecasts. They can be found in Classes corresponding to each of the primitive types: Integer for int, Character for char, and also in String class.
Convert primitive data type to String
The String class has a series of String.valueOf(primitive-data-type) methods so each primitive data type can be converted to String. The print() uses these methods to show output for different primitives.
public class Typecasting {
public static void main (String[] args) {
int number = 124;
// typecast int to String
String numberToString = (String) number; // Type mismatch error - can not cast int to String
// Convert int to String
String numberAsString = String.valueOf(number);
System.out.println(numberAsString); // Output: 124
}
}
Convert String to primitive data type
Java provides useful methods for String parsing, so it is easy to work with the user input or incoming data. As the String.valueOf() methods are defined in String class as the class of the resulting value, definitions for parsing of String methods are defined in different Classes corresponding to primitive data types.
There are two options to get value from String: valueOf() and parse() methods. The only difference is that valueOf returns an object, and parse returns a primitive data type.
public class Typecasting {
public static void main (String[] args) {
String input = "125";
Double inputValue1 = Double.valueOf(input); // valueOf returns Double object
double inputValue2 = Double.parseDouble(input); // parseDouble returns double primitive
System.out.println(inputValue1 + inputValue2); // Output: 250.0
}
}
If the string variable cannot be converted/parsed into the expected data type then at a runtime NumberFormatException exception occurs.
With this article at OpenGenus, you must have the complete idea of Type Conversion in Java.