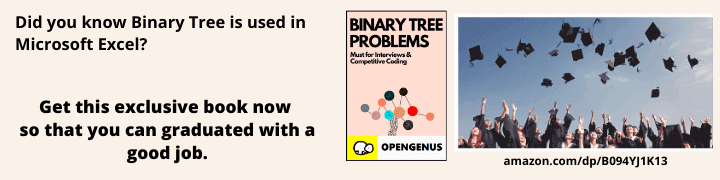
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 25 minutes | Coding time: 5 minutes
There are libraries through which allow us to read and write data to the standard output.For this purpose we include the iostream header file in our code. Similarly,to be able to read and write data to a file we have another library to our rescue namely fstream.Here we will be discussing the different data types and functionality which we will be useful to us in writing and reading data from a file.
There are namely three data types in this header file and we will be discussing their uses below:
-
ofstream
-
ifstream
-
fstream
-
ofstream
Ofstream basically denotes the output file in which the data is to be written.
- ifstream
Ifstream denotes the input file from which the data has to be read.
- fstream
fstream denotes the header file through which we are able to write and read data from the file.
Talking about the different modes in which you can open a file
- ios::app: append mode to append data to the file
- ios::ate: open a file in this mode for output and read/write controlling to the end of the file
- ios::in: open file in this mode for reading
- ios::out: open file in this mode for writing
- ios::trunk: the previous contents of the file will be truncated at the time of opening the file
We will learn three basic operations:
- Opening and closing a file
- Appending data to the front of a file
- Appending data to end of a file (default)
Opening a file in C++
To be able to perform read and write operations on a file it must be firstly opened and then only we are allowed to perform these instructions on the file.
We use ifstream object to open a file for only reading purposes and we don't have the permissions to edit the file and while opening the file by making an ofstream object of fstream object we have the permission to perform write operations on the file.
SYNTAX FOR OPENING A FILE
void open(const char *filename, ios::openmode mode);
Here the first argument denotes the filename which needs to be opened.However do make sure that the file is present in the folder where you have stored your C++ file.The second argument denotes the mode in which the files needs to be opened.We use ios:: which means input output streams.
SYNTAX FOR CLOSING A FILE
void close();
SYNTAX FOR OPENING A FILE FOR READING AND WRITING PURPOSE
fstream file;
file.open("opengenus_test.dat", ios::out | ios::in );
EXAMPLE OF READING AND WRITING IN A FILE IN C++
#include<iostream>
#include<fstream>
using namespace std;
int main()
{
//opening a file in writing mode which is default.
ofstream file;
file.open("opengenus_test.dat");
COUT<<"ENTER YOUR MESSAGE";
//taking in put from user to write to test.dat file
string message;
cin>>mesage;
file<< message << endl;
//We need to close every file which you open.
file.close();
cout<<"THE OUTPUT IS";
//Opening a file in read mode
ifstream file;
file.open("opengenus_test.dat");
file >> message;
//Printing the data
cout << message << endl;
//Closing the file
file.close();
}
The output of the file is:
ENTER YOUR MESSAGE
OpenGenus IQ
THE OUTPUT IS
OpenGenus IQ
Adding data to front of file
Here we will be opening the first file in read mode and print the data of it into the another file and after that open the new file in read mode and print the appended file.
In short, the steps are as follows:
fin.open("opengenus_file.txt");
fout.open("opengenus_file2.txt",ios::app);
fout<<fin.rdbuf();
f.open("file2.txt");
//Printing the file
while(f >> message)
{
cout << message << " ";
}
Following is the complete C++ code:
#include<iostream>
#include<fstream>
using namespace std;
int main()
{
//Object for file operations
fstream f;
//Object for opening file in read mode
ifstream fin;
//Opening the file
fin.open("opengenus_file.txt");
//Object creation for opening file in write and read mode
ofstream fout;
fout.open("opengenus_file2.txt",ios::app);
if(!fin.is_open())
{
cout<<"File does not exist";
}
else
{
//writing the contents of the first file to the second file
fout<<fin.rdbuf();
}
string message;
f.open("opengenus_file2.txt");
//Printing the file
while(f>>message)
{
cout<<message<<" " ;
}
}
Appending to end of file in C++
Here we are going to take a input from a user and we are going to append the input at the end of file. By default, appending to the file takes place at the end of the file.
In short, the steps are:
file.open("opengenus_test.txt",ios::out | ios::app);
// appending the message to the file
file << message << endl;
Following is the complete C++ code:
#include<iostream>
#inlcude<fstream>
using namespace std;
int main()
{
//object of the file to perform operations on it
fstream file;
//here we are going to open the file in append mode (ios::app) or printing mode (ios::out) both
file.open("opengenus_test.txt",ios::out | ios::app);
string message;
cout<<"ENTER THE MESSAGE TO APPEND TO THE FILE";
cin>>message;
//appending the message to the file
file<<message<<endl;
//closing the file
file.close();
}
Thanking you for your time and hoping this will help you where ever you want to make use of it. Enjoy.