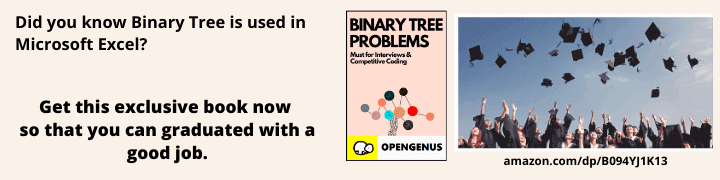
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 10 minutes
In this article, we will work with ZIP file system in Java and see how we can move or copy files to ZIP, delete files from ZIP and rename a file in ZIP.
Before going into the code and details, we will go through some basic information.
A File System in Computer is Something which tells us how a file can Retrieved and Stored in Computer. In Java we can use a Zip File System in extension to the default file system.
As the name Suggests Zip File System. It Deals with all the files having extension of .zip and .rar files .
In Java a Zip File system is created multiple times for every zip or jar file.So what it means?
For Example :- If you have to access one jar file or zip file .A zip file system will be initialised using the reference of the File system provider for only that specific jar file.It cannot be used for another jar file at the same time.
Configuration(Properties) of Zip File System
- All the Configuration of Zip file System are specified in a HashMap which is included in the java.util package
- Create:- Value allowed true or false.The Default value is false.If the value true user allowed to create a zip file if it doesn't exists .We will learn about this property in the following code.
- Encode :- It accepts the String which tell us the encoding scheme.This help user to specify the encoding of the zip file.
Creating instance of File System
To Execute basic file system commands like copy and move files we firstly need to create a instance of the file system as shown in the code
URI uri = URI.create("jar:file:/codeSamples/zipfs/zipfstest.zip");
FileSystem fs = FileSystems.newFileSystem(uri, env);
Here newFileSystem() is a static method which help us to intialise a new file system for specific jar file .This method is overloaded with various other parameters as shown in the image below.
What is env in the above code?
Hashmap which describes the properties of zipfileSystem mentioned above in this article.The unique key is defined as the property of the filsystem
Map<String, String> env = new HashMap<>();
env.put("create", "true");
Manuplating data into Zip File
Once you have an instance of a zip file system, you can invoke the methods of the java.nio.file.FileSystem to perform operations such as copying, moving, and renaming files, as well as modifying file attributes.
Moving or Copying files into Zip or jar filesystem
Go through this code and then, we will understand how it working.
import java.util.*;
import java.net.URI;
import java.nio.file.Path;
import java.nio.file.*;
public class ZipFSPUser {
public static void main(String [] args) throws Throwable {
Map<String, String> env = new HashMap<>();
env.put("create", "true");
URI uri = URI.create("jar:file:/codeSamples/zipfs/zipfstest.zip");
try {
FileSystem zipfs = FileSystems.newFileSystem(uri, env);
Path externalTxtFile = Paths.get("/codeSamples/zipfs/SomeTextFile.txt");
Path pathInZipfile = zipfs.getPath("/SomeTextFile.txt");
// copy a file into the zip file
Files.copy( externalTxtFile,pathInZipfile,
StandardCopyOption.REPLACE_EXISTING );
}
catch(Exceptio e){
System.out.print(e);
}
}
}
Understanding the code above:-
Intially be seeing the code we can easily see that there is a HashMap which contains the properties of the filesystem and instance of FileSystem of the zip file .
The next step is to specify the path of the source file in the default filesystem i.e (Computer filesystem) and the destination filesystem is the zip folder and the path is given according to zip folder and in zip file system the zip folder is the root folder.What does it means?
It means if we have 2 folders in zip file it will be accesible
through the following command
/(Folder Name)/Files/
Deleting Files in Zip Folder
So how de we delete a file in file System ?
Similarily we will delete a file in zip folder because zip folder is now a zip file system.
The following is the code for deletion of File in file system
import java.util.*;
import java.net.URI;
import java.nio.file.Path;
import java.nio.file.*;
import java.nio.file.StandardCopyOption;
public class ZPFSDelete {
public static void main(String [] args) throws Exception {
Map<String, String> zip_properties = new HashMap<>();
zip_properties.put("create", "false");
URI zip_disk = URI.create("jar:file:/my_zip_file.zip");
try {
(FileSystem zipfs = FileSystems.newFileSystem(zip_disk, zip_properties));
Path pathInZipfile = zipfs.getPath("/source.sql");
Files.delete(pathInZipfile);
System.out.println("File successfully deleted");
}
catch(Exception e)
{
System.out.print(e);
}
}
}
Understanding the code:-
So what are we doing in the code?
So zip file system is nothing it is another file system in which can delete the file like ant other file system.File.delete() is the method used to delete to file in a file system .Similaritly for deleting a file in zip file(Zip File System) File.delete() will be used to delete the file.
Renaming a file in zip folder
Go through this code and then, we will understand how it working.
import java.util.*;
import java.net.URI;
import java.nio.file.Path;
import java.nio.file.*;
import java.nio.file.StandardCopyOption;
public class ZPFSDelete {
public static void main(String [] args) throws Exception {
Map<String, String> zip_properties = new HashMap<>();
zip_properties.put("create", "false");
URI zip_disk = URI.create("jar:file:/my_zip_file.zip");
try {
(FileSystem zipfs = FileSystems.newFileSystem(zip_disk, zip_properties));
Path pathInZipfile = zipfs.getPath("/source.sql");
Path renameFile=zipfs.getPath("/source1.sql");
File obj=new File(String.valueOf(pathinZipfile);
//Doing this because file constructor only accepts it in string
if(obj.renameto(renameFile)){
System.out.print("Renamed");
}
else{
System.out.print("Not renamed");
}
}
catch(Exception e)
{
System.out.print(e);
}
}
}
Understanding the code:-
So renaming a file in zip folder is same as renaming a file in default file system .
We are creating a instance of file in zip file system and path of a new file to which it have to be renamed.
Using the renameto() function it is renamed to the Renamed file.
The parameters of the fucntion is the destination or path of the Destination renamed file.
Thank You