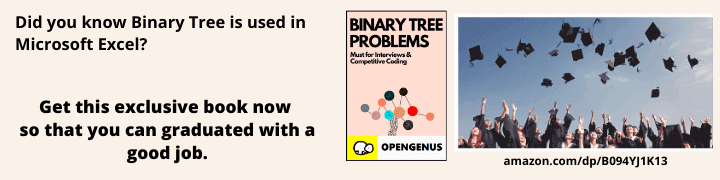
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of Contents
I. Requirements
II. Image Processing and its importance in ML
III. Brightness and Contrast
IV. Importance of Brightness and Contrast
V. Manipulating Brightness and Contrast
VI. Conclusion
Requirements
Note that you need Python and other modules listed below installed on your device before you run the code snippets.
- Python
- Numpy
- OpenCV
- Scikit-Image
- PIL
- Imageio
Image processing took a new turn when Machine Learning applications found a way to vectorize images and learn from them. Image data henceforth became the most valueable type of data with applications such as object detection and recognition, image classification, facial recognition, medical imaging, and image restoration and enhancement.
These are just a few examples of how image processing is required in machine learning applications, but how are images prepared for the machine learning pipeline to handle? What are the key features of image processing? Read on to find out...
What is the importance of image processing in Machine Learning?
Machine learning models learn to make predictions based on the patterns and relationships present in the input data. If the input data is of poor quality, it can be difficult for the model to learn these patterns and relationships, which can result in poor performance.
Image processing is an important preprocessing step in machine learning because it can greatly improve the quality of the input data, which can in turn improve the performance of machine learning models.
- Brightness and Contrast: The two most important features of an image. What do they refer to?
Brightness refers to the overall lightness or darkness of an image. When the brightness of an image needs to be adjusted, a constant value is added or subtracted from every pixel in the image, making the entire image appear lighter or darker respectively.
For example consider an image with 16 pixels. This is represented as a 4x4 matrix with each cell denoting the magnitude of the individual pixel.
0 0 0 0
0 0 0 0
0 0 0 0
0 0 0 0
- Now let's see how we can manipulate the brightness of such an image using Python.
image = [[0 for x in range(4)] for y in range(4)]
brightness_increase = 50
# This will increase the brightness 50 times
for i in range(len(image)):
for j in range(len(image[i])):
image[i][j] += brightness_increase
if image[i][j] > 255:
image[i][j] = 255
- Thus our image matrix will look something like this:
The image matrix after applying the method above:
50 50 50 50
50 50 50 50
50 50 50 50
50 50 50 50
Contrast on the other hand, refers to the difference between the lightest and darkest areas of an image. When the contrast of an image needs to be adjusted, the pixel range of the entire image is stretched or compressed making the lighter areas appear lighter and the darker areas appear darker.
- From the same example of a 4x4 image above, contrast manipulation is a bit complex. The following function explains how to change the contrast values for such an image.
- The code calculates the average pixel value of the image and iterates over each pixel, increasing its contrast by the specified value and finally clamping the output to the valid range of [0,255].
- Formula to calculate contrast value:
(max pixel value - min pixel value) * contrast scale + given pixel value
image = [[0 for x in range(4)] for y in range(4)]
contrast_increase = 1.5
# Control contrast by changing the value above
# Find the average pixel value
avg_pixel = sum([sum(row) for row in image]) / (len(image) * len(image[0]))
for i in range(len(image)):
for j in range(len(image[i])):
# Increase the contrast by scaling the distance from the average pixel value
image[i][j] = (image[i][j] - avg_pixel) * contrast_increase + avg_pixel
# Ensure pixel values remain within the valid range [0, 255]
if image[i][j] > 255:
image[i][j] = 255
elif image[i][j] < 0:
image[i][j] = 0
Why are Brightness and Contrast important in Image Processing?
Brightness and contrast are important aspects of image processing because they can greatly affect the visual quality of an image. Adjusting or normalizing the brightness and contrast of an image can improve its overall quality, making it easier for an ML model to interpret. For example, brightness and contrast can be manipulated to:
-
Enhance image quality by correcting for lighting imbalances and sharpening the details.
-
Extract useful information from images, such as edges and corners for detection tasks.
-
Normalize images so that the whole dataset has same properties.
All these steps make it easier for machine learning models to learn from the images.
How can one manipulate the Brightness and Contrast of larger images?
Now, the code seen above can manipulate the brightness and contrast of images without any problem, but often the images turn out to be more complex and require parameters that are excessive to code.
To solve that, there are several Python libraries that enable us to manipulate the brightness and contrast of an image efficiently and robustly.
- OpenCV: OpenCV is an open-source library that was originally developed by Intel. Perhaps the most popular computer vision library, it is widely used for computer vision tasks such as object detection, face detection, and image segmentation, but it also contains many useful functions for general image processing and supports other programming languages such as C++.
Here is an example that shows how one can manipulate the brightness and contrast of an image in OpenCV:
import cv2
image = cv2.imread('image.jpg')
new_image = cv2.convertScaleAbs(image, alpha=1.5, beta=50)
# alpha controls contrast, beta controls brightness
cv2.imshow('Original Image', image)
cv2.imshow('New Image', new_image)
cv2.waitKey(0)
- Scikit-Image: Scikit-image is another powerful library for image processing in Python. It is rather complicated and has a steeper learning curve, but it provides a wide range of algorithms for image processing, including functions for adjusting brightness and contrast.
Here is an example that shows how one can manipulate the brightness and contrast of an image using Scikit-Image:
from skimage import io, exposure
image = io.imread('image.jpg')
new_image = exposure.adjust_gamma(image, gamma=0.5, gain=1)
# gamma < 1 will brighten the image, gamma > 1 will darken the image
new_image = exposure.rescale_intensity(image, in_range=(50, 200))
# increase contrast by rescaling the intensity range
io.imshow(new_image)
io.show()
- PIL: PIL (Python Imaging Library) is another popular library for image processing in Python. The biggest advantage of PIL is that it provides a wide range of functions for opening, manipulating, and saving many different image file formats such as JPEG, PNG, TIFF and many more.
Here is an example that shows how one can manipulate the brightness and contrast of an image using PIL:
from PIL import Image, ImageEnhance
image = Image.open('image.jpg')
enhancer = ImageEnhance.Brightness(image)
new_image = enhancer.enhance(1.5)
# factor > 1 will brighten the image, factor < 1 will darken the image
enhancer = ImageEnhance.Contrast(image)
new_image = enhancer.enhance(1.5)
# factor > 1 will increase contrast, factor < 1 will decrease contrast
new_image.show()
- ImageIO: Imageio is a library that mainly provides an easy interface to read and write a wide range of image data, including animated images, GIF's, volumetric data, and scientific image formats.
Here is an example that shows how one can manipulate the brightness and contrast of an image using ImageIO:
import imageio
import numpy as np
image = imageio.imread('image.jpg')
def adjust_brightness(image, factor):
mean = np.mean(image)
new_image = np.clip(image * 1.5, 0, 255).astype(np.uint8)
return new_image
def adjust_contrast(image, factor):
mean = np.mean(image)
new_image = np.clip((image - mean) * factor + mean, 0, 255).astype(np.uint8)
return new_image
image = imageio.imread('image.jpg')
new_image = adjust_contrast(image, 1.5)
# factor > 1 will increase contrast, factor < 1 will decrease contrast
new_image = adjust_brightness(image, 1.5)
# factor > 1 will brighten the image, factor < 1 will darken the image
imageio.imshow(new_image)
Conclusion:
In each of these examples above, the brightness and contrast of the image are adjusted by rescaling the intensity range of the pixel values. This can make the light areas appear lighter and the dark areas appear darker, or make the whole image lighter or darker; thereby increasing the overall contrast and brightness of the image.
The usage of these libraries will vary depending on the specific task.
For basic image manipulation tasks such as adjusting brightness and contrast, PIL and ImageIO are relatively straightforward to use. They provide a simple interface, easy-to-use functions for opening, manipulating, and saving images for simple image processing tasks.
OpenCV and Scikit-Image on the other hand more powerful libraries that provide a wider range of image processing functions. These have a steeper learning curve for beginners, but they also provide more advanced functionality for complex image processing tasks.