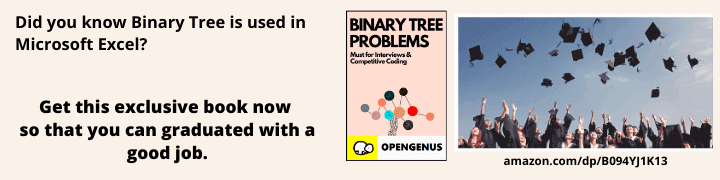
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Abstract
This OpenGenus article explores the role of sentiment analysis in understanding the emotional dynamics of mental health platforms, where individuals share their thoughts and seek support. A Python program has been given, showcasing the contextual awareness and advantages of NLTK's VADER in analyzing sentiments from mental health websites. It emphasizes the importance of understanding user emotions to enable early intervention and customize user experiences. It also highlights the future scope, like including user feedback integration and multimodal analysis, to enhance the sentiment analysis and fostering positive online mental health communities.
Table of Contents
Topics | |
---|---|
1. | Introduction |
2. | The Importance of Sentiment Analysis in Mental Health Platforms |
3. | Building a Sentiment Analysis Project in Python |
4. | Modules Used |
- NLTK's VADER | |
- Requests | |
- BeautifulSoup | |
- Pandas | |
- Matplotlib's Pyplot | |
- Seaborn | |
5. | More on VADER |
6. | Approach and Advantages |
- Comparison with Other Approaches | |
7. | Sample Evaluation Results |
8. | Conclusion |
Introduction
In an era where digital platforms serve as spaces for sharing thoughts and emotions and seeking support, sentiment analysis plays a crucial role in understanding the well-being of individuals. Mental health sites and forums, in particular, are places where people open up about their struggles, making them important for exploration through sentiment analysis.
The Importance of Sentiment Analysis in Mental Health Platforms
Understanding User Emotions:
Sentiment analysis helps in discerning the emotional tone of user-generated content. This is especially valuable in mental health platforms, where users share their experiences, thoughts and feelings. Identifying sentiments like sadness, happiness, or anxiety can provide insights into the overall emotional well-being of the community.
Early Intervention and Support:
Detecting negative sentiments early on allows timely intervention and support. Mental health professionals and moderators can be alerted about potentially concerning posts, allowing a quicker response and assistance for those in need.
Community Health Monitoring:
Analyzing sentiments collectively provides a broad perspective on the overall emotional atmosphere within a community. Tracking sentiment trends over time helps administrators identify patterns, assess the effectiveness of interventions, and adapt support strategies accordingly.
Customized User Experiences:
Utilizing sentiment analysis allows us to customize user experiences. For example, when a user consistently conveys positive sentiments, the platform can recommend uplifting content or resources. Conversely, a user exhibiting signs of distress may receive targeted resources or outreach.
Building a Sentiment Analysis Project in Python
Let's walk through a simple Python project using the VADER (Valence Aware Dictionary and sEntiment Reasoner) module from NLTK (Natural Language Toolkit) for mental health websites for sentiment analysis.
from nltk.sentiment.vader import SentimentIntensityAnalyzer
import requests
from bs4 import BeautifulSoup
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sb
def analyze_sentiment(text):
analyzer = SentimentIntensityAnalyzer()
sentiment_score = analyzer.polarity_scores(text)['compound']
if sentiment_score >= 0.05:
return 'Positive'
elif sentiment_score <= -0.05:
return 'Negative'
else:
return 'Neutral'
def get_text(url):
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
text = ' '.join([p.get_text() for p in soup.find_all('p')])
return text
else:
print(f"Failed to retrieve content from {url}. Status code: {response.status_code}")
return None
def prepare_dataset(url):
text = get_text(url)
if text:
words = text.split()
sentiments = [analyze_sentiment(word) for word in words]
df = pd.DataFrame({'Word': words, 'Sentiment': sentiments})
df.to_csv('website_sentiments.csv', index=False)
def visualize_sentiments():
df = pd.read_csv('website_sentiments.csv')
if 'Word' not in df.columns or 'Sentiment' not in df.columns:
print("Dataset columns are not as expected. Please check the dataset format.")
return
sentiment_counts = df['Sentiment'].value_counts()
plt.figure(figsize=(8, 6))
sb.barplot(x=sentiment_counts.index, y=sentiment_counts.values, palette="viridis")
plt.title('Number of Neutral, Positive, and Negative Words')
plt.xlabel('Sentiment')
plt.ylabel('Word Count')
plt.show()
website_url = "https://manochikitsa.com/topic/i-feeling-anxiety-and-dont-feel-like-doing-anything/"
prepare_dataset(website_url)
visualize_sentiments()
Modules Used
NLTK's VADER:
-
NLTK (Natural Language Toolkit): NLTK is a powerful library for working with human language data. It provides easy-to-use interfaces to lexical resources such as WordNet and various text-processing libraries.
-
VADER (Valence Aware Dictionary and sEntiment Reasoner): VADER is a sentiment analysis tool designed for analyzing sentiments in text. It is particularly useful for social media text and is pre-trained on a vast corpus of text data.
Requests:
The Requests module is used for sending HTTP requests to a specified URL. It simplifies the process of making HTTP requests and handling the responses.
BeautifulSoup:
BeautifulSoup is a Python library for pulling data out of HTML and XML files. It provides Pythonic idioms for iterating, searching, and modifying the parse tree. In this context, it's used to scrape and extract text content from HTML.
Pandas:
This module is used for data manipulation and analysis. It provides data structures like DataFrame, which is used to store and manipulate the data about words and their sentiments.
Matplotlib's pyplot:
Pyplot is part of the Matplotlib library and is used for creating visualizations such as bar charts. In this program, we have used it to visualize the number of neutral, positive, and negative words.
Seaborn:
This module is used for statistical data visualization. It's been used along with with Matplotlib to create a visually appealing bar chart.
More on VADER
VADER (Valence Aware Dictionary and sEntiment Reasoner) is a sophisticated tool that leverages a unique blend of sentiment lexicons, grammatical rules, and empirically derived heuristics. At its heart lies a sentiment lexicon, a repository of words each tagged with pre-assigned sentiment scores. These scores are not static; they dynamically interact based on the grammatical structure of sentences to compute sentiment intensity. This computation is sensitive to both the polarity of individual words and their syntactic arrangement, allowing VADER to perform a nuanced sentiment analysis.
Contrary to decision tree models, which are prevalent in machine learning for sentiment analysis, VADER adopts a rule-based approach. It does not rely on decision trees but instead uses a combination of lexical elements and grammatical heuristics. This method is adept at determining both the polarity and intensity of sentiments expressed in text.
VADER operates on a set of pre-defined rules coupled with its sentiment lexicon. The lexicon is the cornerstone, containing words pre-assigned with sentiment scores. These scores are intricately woven together, considering the grammatical makeup of the text, to yield an overall sentiment score. VADER is contextually aware, adeptly handling linguistic nuances such as negations and booster words that can significantly alter sentiment interpretation.
Approach and Advantages
The Python script follows the following steps:
- It retrieves the content of a specified URL using the requests module.
- It extracts text from the webpage using BeautifulSoup.
- The SentimentIntensityAnalyzer from the VADER module then analyzes the sentiment of the extracted text.
- The text is tokenized into words. For each word, we use the sentiment analysis tool to determine its sentiment. We create a dataset with columns 'Word' and 'Sentiment' and save it to a CSV file ('website_sentiments.csv').
- A bar chart is created using matplotlib.pyplot and seaborn to visualize the number of neutral, positive, and negative words.
The VADER approach is advantageous for several reasons:
-
Sensitivity to Context:
VADER is designed to understand the contextual nuances of sentiments, making it apt for analyzing informal and emotional language often found on mental health forums. -
Negation Handling:
VADER considers negations in sentiment analysis, which is crucial in understanding the sentiment of sentences where the meaning can be reversed by negating words. -
Emotion Intensity:
VADER not only identifies the sentiment polarity but also provides a sentiment intensity score, giving a better understanding of the emotional tone.
Comparison with Other Approaches
While there are various sentiment analysis approaches, VADER stands out in the context of mental health forums due to its context awareness and emphasis on sentiment intensity. Approaches such as other machine learning-based methods, might require extensive training data and may not perform optimally on text rich in emotional content. Their success is contingent on the availability of comprehensive training data. Rule-based approaches, on the other hand, may lack the contextual awareness necessary to capture the subtleties of emotional expression in these forums.
In the end, the choice of approach depends on the specific requirements of the sentiment analysis task and the characteristics of the data at hand. In the case of mental health forums, the VADER approach stands out for its ability to grasp the complexity of emotions expressed in the often informal and subtle language of these online communities.
Sample Evaluation Results:
After running the provided Python script on the specified mental health website, the sentiment analysis yielded the following results:
Here are the results of analysis done on a Reddit discussion:
The bar chart visually represents the number of neutral, positive, and negative words extracted from the website. This breakdown provides insights into the emotional tone of the user-generated content.
Conclusion
There are many possibilities for enhancing the presented sentiment analysis project on mental health platforms. Future improvements could involve integrating user feedback, allowing the model to adapt and evolve based on the language and expressions used within the community. This process ensures that the sentiment analysis remains finely tuned to the evolving dynamics of the platform.
Moreover, the scope of analysis can be expanded to include multimedia content such as images and videos, enabling a more comprehensive understanding of user sentiment. As mental health discussions often transcend text, incorporating a multimodal approach would provide a much better perspective on the forum's emotional landscape.
By embracing user feedback, incorporating multimodal elements and implementing real-time monitoring, sentiment analysis can evolve into a more responsive, adaptive, and supportive tool for online mental health communities. These improvements aim not only to understand sentiments better but also to contribute actively to the well-being and positive atmosphere of our digital spaces.